Improve Golang program to solve accuracy problem
To optimize Golang programs and solve the problem of precision loss, specific code examples are needed
In the daily programming process, we often encounter the problem of precision loss, especially When using Golang for numerical calculations. These precision losses may be caused by floating point calculations, type conversions, etc., which have a certain impact on the accuracy and performance of the program. This article will introduce how to optimize Golang programs, solve the problem of precision loss, and provide specific code examples.
1. Use a high-precision calculation library
If you need to perform high-precision numerical calculations in your program, you can consider using Golang's high-precision calculation library, such as math/big
Bag. This package provides support for arbitrary-precision integer and rational number operations, which can avoid the problem of precision loss. The following is a simple example:
package main import ( "fmt" "math/big" ) func main() { a := new(big.Float).SetPrec(128).SetFloat64(0.1) b := new(big.Float).SetPrec(128).SetFloat64(0.2) c := new(big.Float).Add(a, b) fmt.Println(c) }
In this example, we use the math/big
package to perform high-precision floating point calculations, avoiding the precision caused by ordinary floating point calculations Lost problem.
2. Use the Decimal type
In addition to using a high-precision calculation library, you can also consider using the Decimal type to represent numerical values. The Decimal type provides greater precision and better control over the precision of numeric values. The following is a simple example:
package main import ( "fmt" "github.com/shopspring/decimal" ) func main() { a := decimal.NewFromFloat(0.1) b := decimal.NewFromFloat(0.2) c := a.Add(b) fmt.Println(c) }
In this example, we use the Decimal type provided by the github.com/shopspring/decimal
package to perform numerical calculations to avoid the problem of precision loss. .
3. Decimal point precision control
In addition to using the high-precision calculation library and the Decimal type, you can also solve the problem of precision loss by controlling the decimal point precision. You can set the precision before performing floating point calculations to avoid loss of precision. The following is an example:
package main import ( "fmt" ) func main() { a := 0.1 b := 0.2 sum := a + b c := fmt.Sprintf("%.10f", sum) fmt.Println(c) }
In this example, we set the decimal point precision to 10 through the fmt.Sprintf
function before performing floating point calculations, thereby avoiding the problem of precision loss.
To sum up, by using high-precision calculation libraries, Decimal types and controlling decimal point precision, we can effectively optimize Golang programs and solve the problem of precision loss. It is hoped that these specific code examples can help readers better deal with the problem of precision loss and improve the accuracy and performance of the program.
The above is the detailed content of Improve Golang program to solve accuracy problem. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


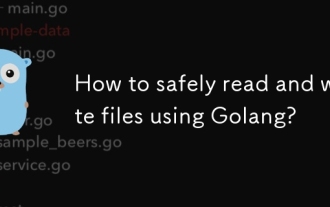
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
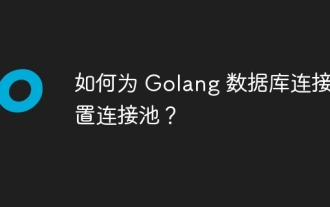
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
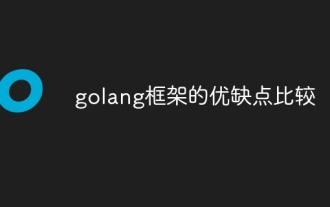
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Recently, "Black Myth: Wukong" has attracted huge attention around the world. The number of people online at the same time on each platform has reached a new high. This game has achieved great commercial success on multiple platforms. The Xbox version of "Black Myth: Wukong" has been postponed. Although "Black Myth: Wukong" has been released on PC and PS5 platforms, there has been no definite news about its Xbox version. It is understood that the official has confirmed that "Black Myth: Wukong" will be launched on the Xbox platform. However, the specific launch date has not yet been announced. It was recently reported that the Xbox version's delay was due to technical issues. According to a relevant blogger, he learned from communications with developers and "Xbox insiders" during Gamescom that the Xbox version of "Black Myth: Wukong" exists.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
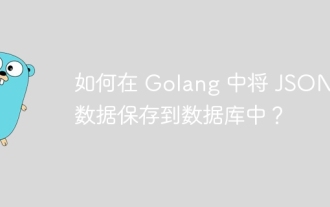
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
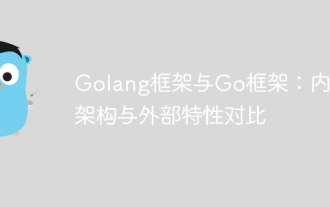
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
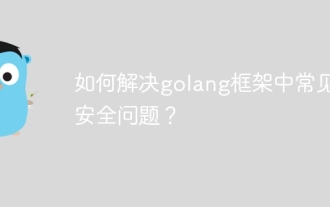
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
