Explore the underlying mechanism of Golang type conversion
Golang language is a statically typed language, and type conversion is one of the common operations in programs. Through type conversion, data can be converted and transferred between different types to achieve more flexible programming logic. This article will delve into the implementation principles of Golang type conversion and give specific code examples.
1. Basic concepts of type conversion in Golang
In Golang, type conversion involves converting a value from one type to another type. Type conversion in Golang is mainly divided into two types: forced type conversion and type assertion.
- Forced type conversion: To convert one type to another type, you need to explicitly use the type conversion operator.
- Type assertion: used to determine the actual type of the interface object and convert it to a specific type.
2. The implementation principle of type conversion in Golang
In Golang, the implementation principle of type conversion is mainly implemented through type assertions and interfaces. Type assertions are used to determine the actual type of an interface object and convert it to a specific type. Interface implementation implements type conversion through the type information stored in the interface object.
Type conversion in Golang is a low-level operation, which requires programmers to have a certain understanding of the underlying mechanism. In fact, type conversion in Golang is implemented through runtime reflection mechanism. Through reflection, Golang can obtain the type information of the object at runtime and perform corresponding operations.
3. Code examples
Some specific code examples are given below to better illustrate the implementation principle of Golang type conversion:
Forced type conversion example
package main import ( "fmt" ) func main() { var x int32 = 10 var y int64 y = int64(x) // 将int32类型转换为int64类型 fmt.Println(y) }
In the above example, a variable x of type int32 is converted into a variable y of type int64. Conversion between different types can be achieved by using type conversion operators.
Type assertion example
package main import ( "fmt" ) func main() { var x interface{} x = 10 y := x.(int) // 将接口对象x转换为int类型 fmt.Println(y) }
In the above example, an interface object x of type interface{} is converted into a variable y of type int. By using type assertions, you can determine the actual type of the interface object and complete the type conversion operation.
4. Summary
By having an in-depth understanding of the implementation principles of Golang type conversion, we can better use type conversion operations to implement complex logic processing in the program. When writing code, it is recommended to choose an appropriate type conversion method based on actual needs to avoid type mismatch errors. I hope this article can help readers better understand the implementation principles of type conversion in Golang.
The above is the detailed content of Explore the underlying mechanism of Golang type conversion. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
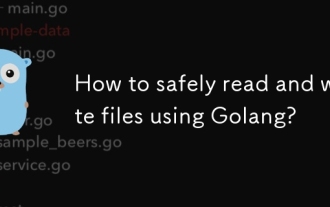
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
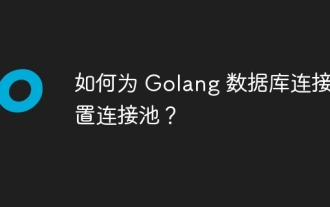
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
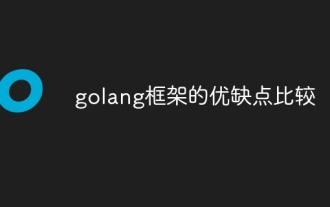
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.
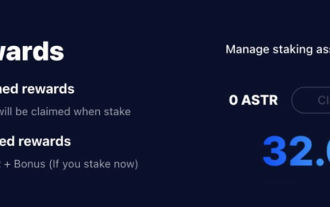
Table of Contents Astar Dapp Staking Principle Staking Revenue Dismantling of Potential Airdrop Projects: AlgemNeurolancheHealthreeAstar Degens DAOVeryLongSwap Staking Strategy & Operation "AstarDapp Staking" has been upgraded to the V3 version at the beginning of this year, and many adjustments have been made to the staking revenue rules. At present, the first staking cycle has ended, and the "voting" sub-cycle of the second staking cycle has just begun. To obtain the "extra reward" benefits, you need to grasp this critical stage (expected to last until June 26, with less than 5 days remaining). I will break down the Astar staking income in detail,

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
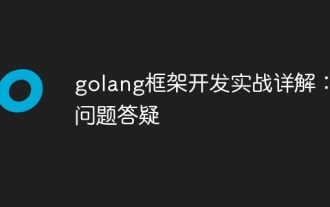
In Go framework development, common challenges and their solutions are: Error handling: Use the errors package for management, and use middleware to centrally handle errors. Authentication and authorization: Integrate third-party libraries and create custom middleware to check credentials. Concurrency processing: Use goroutines, mutexes, and channels to control resource access. Unit testing: Use gotest packages, mocks, and stubs for isolation, and code coverage tools to ensure sufficiency. Deployment and monitoring: Use Docker containers to package deployments, set up data backups, and track performance and errors with logging and monitoring tools.
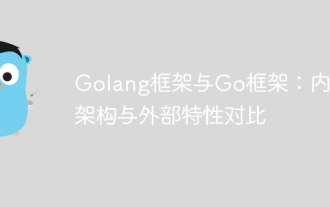
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
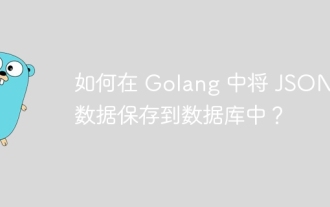
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
