


'PHP Object-Oriented Programming Design Patterns: Understanding SOLID Principles and Their Applications'
PHP Object-oriented programming design patterns have always been a hot topic studied by developers. In this article, PHP editor Strawberry will delve into the SOLID principles to help readers understand and apply these design principles. The SOLID principle is the cornerstone of object-oriented programming, including the single responsibility principle, the open and closed principle, the Liskov substitution principle, the interface isolation principle and the dependency inversion principle. By learning and practicing these principles, developers can write more flexible, maintainable, and scalable PHP code.
- Single Responsibility Principle (SRP): A class should be responsible for only one task, and this task should be encapsulated in the class. This can improve the maintainability and reusability of the class.
class User { private $id; private $name; private $email; public function __construct($id, $name, $email) { $this->id = $id; $this->name = $name; $this->email = $email; } public function getId() { return $this->id; } public function getName() { return $this->name; } public function getEmail() { return $this->email; } } class UserRepository { private $connection; public function __construct($connection) { $this->connection = $connection; } public function save(User $user) { $stmt = $this->connection->prepare("INSERT INTO users (name, email) VALUES (?, ?)"); $stmt->execute([$user->getName(), $user->getEmail()]); } public function find($id) { $stmt = $this->connection->prepare("SELECT * FROM users WHERE id = ?"); $stmt->execute([$id]); $result = $stmt->fetch(); if ($result) { return new User($result["id"], $result["name"], $result["email"]); } return null; } }
- Open-Closed Principle (OCP): Software entities (classes, modules, etc.) should be open to extensions and closed to modifications. This can improve the flexibility of the software and reduce software maintenance costs.
interface Shape { public function getArea(); } class Rectangle implements Shape { private $width; private $height; public function __construct($width, $height) { $this->width = $width; $this->height = $height; } public function getArea() { return $this->width * $this->height; } } class Circle implements Shape { private $radius; public function __construct($radius) { $this->radius = $radius; } public function getArea() { return pi() * $this->radius ** 2; } } class ShapeCalculator { public function calculateTotalArea($shapes) { $totalArea = 0; foreach ($shapes as $shape) { $totalArea += $shape->getArea(); } return $totalArea; } }
- Liskov Substitution Principle (LSP): A subclass can replace its parent class without affecting the correctness of the program. This increases the flexibility of the software and makes it easier to refactor.
class Animal { public function eat() { echo "Animal is eating."; } } class Dog extends Animal { public function bark() { echo "Dog is barking."; } } class Cat extends Animal { public function meow() { echo "Cat is meowing."; } } function feedAnimal(Animal $animal) { $animal->eat(); } feedAnimal(new Dog()); // prints "Dog is eating." feedAnimal(new Cat()); // prints "Cat is eating."
- Interface Segregation Principle (ISP): Multiple specialized interfaces should be used instead of one universal interface. This can improve the readability of the software and reduce software maintenance costs.
interface Printable { public function print(); } interface Scannable { public function scan(); } interface Faxable { public function fax(); } class Printer implements Printable { public function print() { echo "Printer is printing."; } } class Scanner implements Scannable { public function scan() { echo "Scanner is scanning."; } } class FaxMachine implements Faxable { public function fax() { echo "Fax machine is faxing."; } } class MultifunctionDevice implements Printable, Scannable, Faxable { public function print() { echo "Multifunction device is printing."; } public function scan() {
The above is the detailed content of 'PHP Object-Oriented Programming Design Patterns: Understanding SOLID Principles and Their Applications'. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


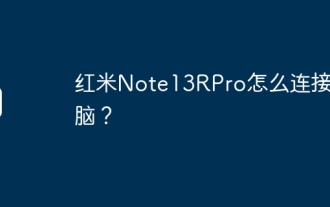
The phone Redmi Note13RPro has been very popular recently. Many consumers have purchased this phone. However, many users are using this phone for the first time, so they don’t know how to connect the Redmi Note13RPro to the computer. In this regard, the editor is here to explain to you Detailed tutorial introduction is provided. How to connect Redmi Note13RPro to the computer? 1. Use a USB data cable to connect the Redmi phone to the USB interface of the computer. 2. Open the phone settings, click Options, and turn on USB debugging. 3. Open the device manager on your computer and find the mobile device option. 4. Right-click the mobile device, select Update Driver, and then select Automatically search for updated drivers. 5. If the computer does not automatically search for the driver,
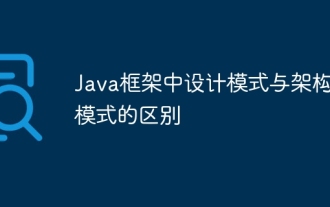
In the Java framework, the difference between design patterns and architectural patterns is that design patterns define abstract solutions to common problems in software design, focusing on the interaction between classes and objects, such as factory patterns. Architectural patterns define the relationship between system structures and modules, focusing on the organization and interaction of system components, such as layered architecture.
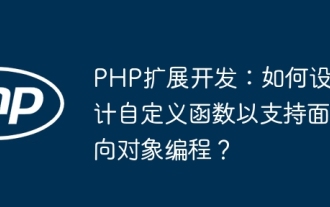
PHP extensions can support object-oriented programming by designing custom functions to create objects, access properties, and call methods. First create a custom function to instantiate the object, and then define functions that get properties and call methods. In actual combat, we can customize the function to create a MyClass object, obtain its my_property attribute, and call its my_method method.
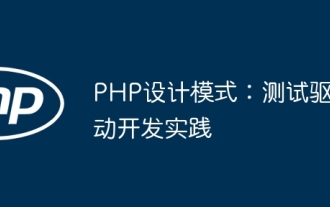
TDD is used to write high-quality PHP code. The steps include: writing test cases, describing the expected functionality and making them fail. Write code so that only the test cases pass without excessive optimization or detailed design. After the test cases pass, optimize and refactor the code to improve readability, maintainability, and scalability.
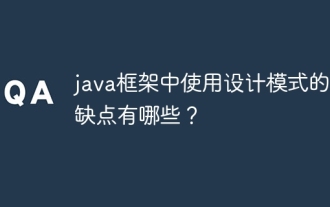
The advantages of using design patterns in Java frameworks include: enhanced code readability, maintainability, and scalability. Disadvantages include complexity, performance overhead, and steep learning curve due to overuse. Practical case: Proxy mode is used to lazy load objects. Use design patterns wisely to take advantage of their advantages and minimize their disadvantages.
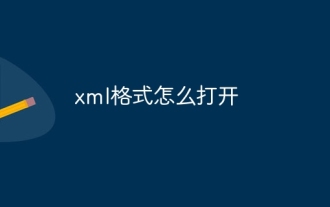
Use most text editors to open XML files; if you need a more intuitive tree display, you can use an XML editor, such as Oxygen XML Editor or XMLSpy; if you process XML data in a program, you need to use a programming language (such as Python) and XML libraries (such as xml.etree.ElementTree) to parse.
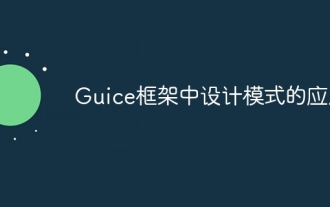
The Guice framework applies a number of design patterns, including: Singleton pattern: ensuring that a class has only one instance through the @Singleton annotation. Factory method pattern: Create a factory method through the @Provides annotation and obtain the object instance during dependency injection. Strategy mode: Encapsulate the algorithm into different strategy classes and specify the specific strategy through the @Named annotation.
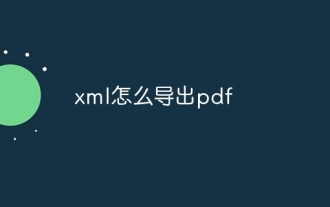
There are two ways to export XML to PDF: using XSLT and using XML data binding libraries. XSLT: Create an XSLT stylesheet, specify the PDF format to convert XML data using the XSLT processor. XML Data binding library: Import XML Data binding library Create PDF Document object loading XML data export PDF files. Which method is better for PDF files depends on the requirements. XSLT provides flexibility, while the data binding library is simple to implement; for simple conversions, the data binding library is better, and for complex conversions, XSLT is more suitable.
