Use Golang to quickly implement unit conversion function
In today's society, unit conversion is an integral part of our lives. Whether in science, engineering or in daily life, we often encounter situations where we need to convert different units. In order to solve this problem, we can use the Golang programming language to quickly implement a unit conversion application. This article will introduce how to write a simple unit conversion application using Golang and provide specific code examples.
First, let’s determine which unit conversions need to be supported. In this example, we choose to implement conversions between length units, including meters, centimeters, inches, and feet. Next, we'll write a function to convert between these units.
package main import "fmt" func convertLength(value float64, fromUnit string, toUnit string) float64 { var result float64 switch fromUnit { case "m": switch toUnit { case "cm": result = value * 100 case "inch": result = value * 39.37 case "ft": result = value * 3.281 } case "cm": switch toUnit { case "m": result = value / 100 case "inch": result = value / 2.54 case "ft": result = value / 30.48 } case "inch": switch toUnit { case "m": result = value / 39.37 case "cm": result = value * 2.54 case "ft": result = value / 12 } case "ft": switch toUnit { case "m": result = value / 3.281 case "cm": result = value * 30.48 case "inch": result = value * 12 } } return result } func main() { value := 1.0 fromUnit := "m" toUnit := "cm" result := convertLength(value, fromUnit, toUnit) fmt.Printf("%.2f %s = %.2f %s ", value, fromUnit, result, toUnit) }
In the above code example, we define a convertLength
function, which accepts a floating point value, the original unit and the target unit as parameters, and then converts the relationship according to different units Calculate the converted result and return it. In the main
function, we simply call the convertLength
function to do the unit conversion and print the result.
In actual applications, this program can be extended as needed to add more unit conversion functions or implement other types of unit conversions. By using Golang, a fast and efficient programming language, we can easily implement various unit conversion functions, improve work efficiency, and make life more convenient.
We hope that the code examples provided in this article can help readers quickly implement the unit conversion function and find more interesting uses in practical applications. If you have any questions or suggestions, please leave a message for discussion. I wish you all to make more progress in your studies and work!
The above is the detailed content of Use Golang to quickly implement unit conversion function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


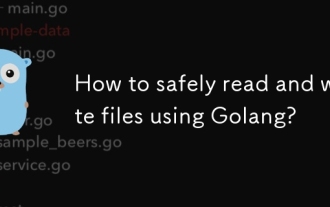
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
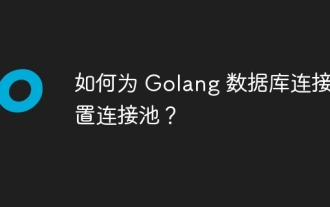
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
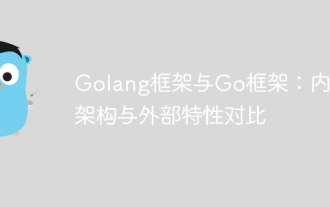
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
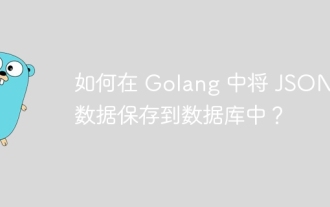
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
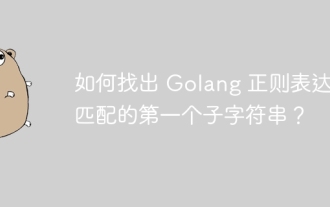
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
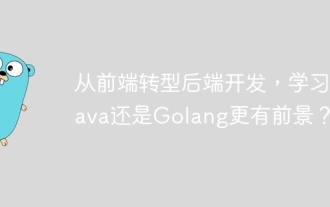
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
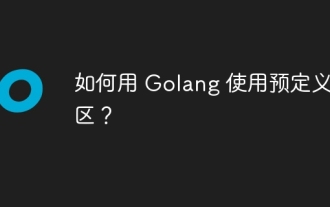
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
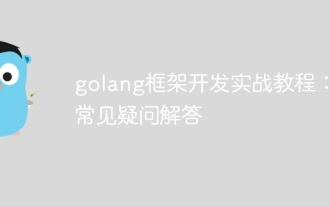
Go framework development FAQ: Framework selection: Depends on application requirements and developer preferences, such as Gin (API), Echo (extensible), Beego (ORM), Iris (performance). Installation and use: Use the gomod command to install, import the framework and use it. Database interaction: Use ORM libraries, such as gorm, to establish database connections and operations. Authentication and authorization: Use session management and authentication middleware such as gin-contrib/sessions. Practical case: Use the Gin framework to build a simple blog API that provides POST, GET and other functions.
