


PHP classes and objects: learn object-oriented programming from scratch in simple terms
PHP classes and objects are the basis of object-oriented programming, which may be difficult for beginners to understand. In this guide, PHP editor Banana will start from scratch and introduce the concepts and basic principles of PHP classes and objects in a simple and easy-to-understand manner to help readers easily understand the important concepts of object-oriented programming. Whether you are a newbie or an experienced developer, this article will provide you with helpful guidance and help you better master the knowledge of PHP classes and objects.
In PHP, a class is the template of an object, which defines the structure of the object's data and methods. An object is an instance of a class. It is created according to the template of the class and has all the data and methods of the class.
To create a class, you can use the class
keyword, followed by the class name. The class name should start with a capital letter. In the definition of a class, you can control the visibility of data using the public
, protected
, and private
keywords.
class MyClass { public $public_data; protected $protected_data; private $private_data; public function __construct($public_data, $protected_data, $private_data) { $this->public_data = $public_data; $this->protected_data = $protected_data; $this->private_data = $private_data; } public function publicMethod() { echo "This is a public method. "; } protected function protectedMethod() { echo "This is a protected method. "; } private function privateMethod() { echo "This is a private method. "; } }
To create an object, you can use the new
keyword, followed by the class name.
$myObject = new MyClass("public data", "protected data", "private data");
To access an object's data and methods, you can use the object's arrow notation (->
).
echo $myObject->public_data; // 输出:public data $myObject->publicMethod(); // 输出:This is a public method.
To call a protected method of an object, you can use the parent::
operator.
class ChildClass extends MyClass { public function callProtectedMethod() { parent::protectedMethod(); // 输出:This is a protected method. } } $childObject = new ChildClass(); $childObject->callProtectedMethod(); // 输出:This is a protected method.
To call the private method of an object, you can use the self::
operator.
class MyClass { private function privateMethod() { echo "This is a private method. "; } public function callPrivateMethod() { self::privateMethod(); // 输出:This is a private method. } } $myObject = new MyClass(); $myObject->callPrivateMethod(); // 输出:This is a private method.
The above is the detailed content of PHP classes and objects: learn object-oriented programming from scratch in simple terms. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


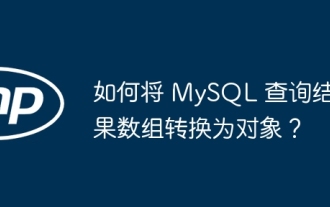
Here's how to convert a MySQL query result array into an object: Create an empty object array. Loop through the resulting array and create a new object for each row. Use a foreach loop to assign the key-value pairs of each row to the corresponding properties of the new object. Adds a new object to the object array. Close the database connection.
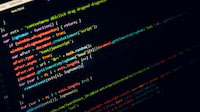
Introduction In today's rapidly evolving digital world, it is crucial to build robust, flexible and maintainable WEB applications. The PHPmvc architecture provides an ideal solution to achieve this goal. MVC (Model-View-Controller) is a widely used design pattern that separates various aspects of an application into independent components. The foundation of MVC architecture The core principle of MVC architecture is separation of concerns: Model: encapsulates the data and business logic of the application. View: Responsible for presenting data and handling user interaction. Controller: Coordinates the interaction between models and views, manages user requests and business logic. PHPMVC Architecture The phpMVC architecture follows the traditional MVC pattern, but also introduces language-specific features. The following is PHPMVC
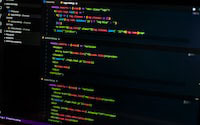
SOLID principles are a set of guiding principles in object-oriented programming design patterns that aim to improve the quality and maintainability of software design. Proposed by Robert C. Martin, SOLID principles include: Single Responsibility Principle (SRP): A class should be responsible for only one task, and this task should be encapsulated in the class. This can improve the maintainability and reusability of the class. classUser{private$id;private$name;private$email;publicfunction__construct($id,$nam
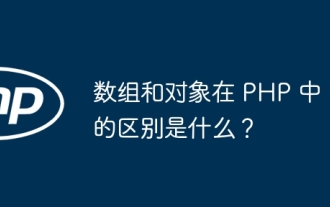
In PHP, an array is an ordered sequence, and elements are accessed by index; an object is an entity with properties and methods, created through the new keyword. Array access is via index, object access is via properties/methods. Array values are passed and object references are passed.
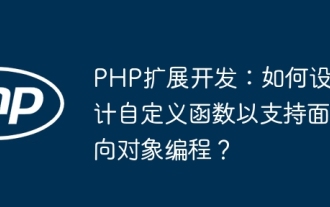
PHP extensions can support object-oriented programming by designing custom functions to create objects, access properties, and call methods. First create a custom function to instantiate the object, and then define functions that get properties and call methods. In actual combat, we can customize the function to create a MyClass object, obtain its my_property attribute, and call its my_method method.
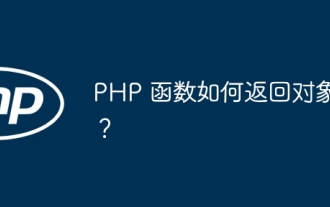
PHP functions can encapsulate data into a custom structure by returning an object using a return statement followed by an object instance. Syntax: functionget_object():object{}. This allows creating objects with custom properties and methods and processing data in the form of objects.
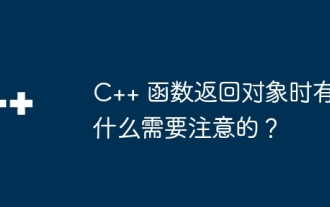
In C++, there are three points to note when a function returns an object: The life cycle of the object is managed by the caller to prevent memory leaks. Avoid dangling pointers and ensure the object remains valid after the function returns by dynamically allocating memory or returning the object itself. The compiler may optimize copy generation of the returned object to improve performance, but if the object is passed by value semantics, no copy generation is required.
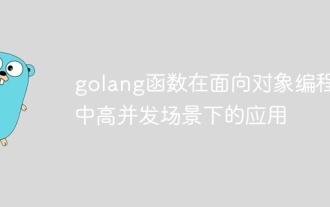
In high-concurrency scenarios of object-oriented programming, functions are widely used in the Go language: Functions as methods: Functions can be attached to structures to implement object-oriented programming, conveniently operating structure data and providing specific functions. Functions as concurrent execution bodies: Functions can be used as goroutine execution bodies to implement concurrent task execution and improve program efficiency. Function as callback: Functions can be passed as parameters to other functions and be called when specific events or operations occur, providing a flexible callback mechanism.
