jQuery implementation of automatically updating table row numbers
jQuery is a popular JavaScript library that is widely used in web development. In web development, we often encounter situations where data needs to be displayed, and tables are a common way of displaying data. In a dynamic table, there are often operations such as deletion, addition, sorting, etc. At this time, it is necessary to automatically update the serial numbers in the table when the number of rows in the table changes. The following will introduce in detail how to use jQuery to achieve this function.
The code example is as follows:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>jQuery implementation of automatically updating table row numbers</title> <script src="https://code.jquery.com/jquery-3.5.1.min.js"></script> <style> table { border-collapse: collapse; width: 100%; } th, td { border: 1px solid black; padding: 8px; text-align: center; } th { background-color: #f2f2f2; } </style> </head> <body> <h1 id="表格示例">表格示例</h1> <table id="data-table"> <thead> <tr> <th>序号</th> <th>姓名</th> <th>年龄</th> </tr> </thead> <tbody> <tr> <td>1</td> <td>Alice</td> <td>25</td> </tr> <tr> <td>2</td> <td>Bob</td> <td>30</td> </tr> <tr> <td>3</td> <td>Charlie</td> <td>28</td> </tr> </tbody> </table> <button id="add-row">新增行</button> <button id="delete-row">删除行</button> <script> // 初始表格序号 function updateRowNumber() { $('#data-table tbody tr').each(function(index) { $(this).find('td:first').text(index + 1); }); } // 新增行 $('#add-row').on('click', function() { $('#data-table tbody').append('<tr><td></td><td>New</td><td>0</td></tr>'); updateRowNumber(); }); // 删除行 $('#delete-row').on('click', function() { $('#data-table tbody tr:last').remove(); updateRowNumber(); }); </script> </body> </html>
In the above code, a table containing name and age is first created, and a header containing serial number, name and age is added. Then I used jQuery to write two event listeners, respectively for adding rows and deleting rows. Among them, the updateRowNumber
function is used to automatically update the serial number in the table when the number of table rows changes. The operations of adding rows and deleting rows will call the updateRowNumber
function, thus realizing the automatic update of the serial number when the number of table rows changes.
Through this code example, you can easily implement the automatic update function of the serial number when the number of table rows changes, so that the table can maintain a good display effect when the data changes dynamically.
The above is the detailed content of jQuery implementation of automatically updating table row numbers. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


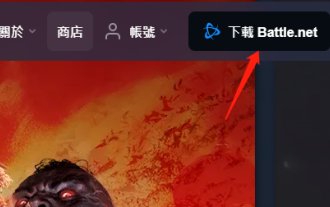
Blizzard Battle.net update keeps stuck at 45%, how to solve it? Recently, many people have been stuck at the 45% progress bar when updating software. They will still get stuck after restarting multiple times. So how to solve this situation? We can reinstall the client, switch regions, and delete files. To deal with it, this software tutorial will share the operation steps, hoping to help more people. Blizzard Battle.net update keeps stuck at 45%, how to solve it? 1. Client 1. First, you need to confirm that your client is the official version downloaded from the official website. 2. If not, users can enter the Asian server website to download. 3. After entering, click Download in the upper right corner. Note: Be sure not to select Simplified Chinese when installing.
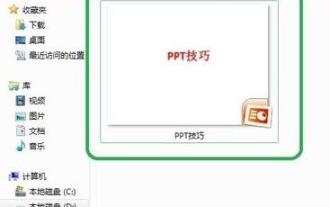
1. Create a new PPT file and name it [PPT Tips] as an example. 2. Double-click [PPT Tips] to open the PPT file. 3. Insert a table with two rows and two columns as an example. 4. Double-click on the border of the table, and the [Design] option will appear on the upper toolbar. 5. Click the [Shading] option and click [Picture]. 6. Click [Picture] to pop up the fill options dialog box with the picture as the background. 7. Find the tray you want to insert in the directory and click OK to insert the picture. 8. Right-click on the table box to bring up the settings dialog box. 9. Click [Format Cells] and check [Tile images as shading]. 10. Set [Center], [Mirror] and other functions you need, and click OK. Note: The default is for pictures to be filled in the table
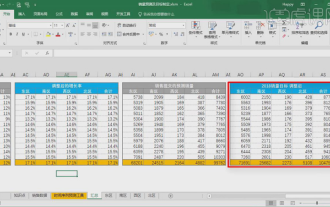
Being able to skillfully make forms is not only a necessary skill for accounting, human resources, and finance. For many sales staff, learning to make forms is also very important. Because the data related to sales is very large and complex, and it cannot be simply recorded in a document to explain the problem. In order to enable more sales staff to be proficient in using Excel to make tables, the editor will introduce the table making issues about sales forecasting. Friends in need should not miss it! 1. Open [Sales Forecast and Target Setting], xlsm, to analyze the data stored in each table. 2. Create a new [Blank Worksheet], select [Cell], and enter [Label Information]. [Drag] downward and [Fill] the month. Enter [Other] data and click [
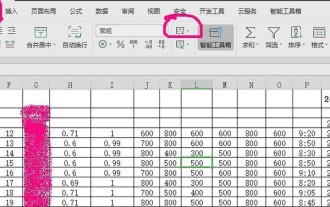
1. Open the worksheet and find the [Start]-[Conditional Formatting] button. 2. Click Column Selection and select the column to which conditional formatting will be added. 3. Click the [Conditional Formatting] button to bring up the option menu. 4. Select [Highlight conditional rules]-[Between]. 5. Fill in the rules: 20, 24, dark green text with dark fill color. 6. After confirmation, the data in the selected column will be colored with corresponding numbers, text, and cell boxes according to the settings. 7. Conditional rules without conflicts can be added repeatedly, but for conflicting rules WPS will replace the previously established conditional rules with the last added rule. 8. Repeatedly add the cell columns after [Between] rules 20-24 and [Less than] 20. 9. If you need to change the rules, you can just clear the rules and then reset the rules.

Angular.js is a freely accessible JavaScript platform for creating dynamic applications. It allows you to express various aspects of your application quickly and clearly by extending the syntax of HTML as a template language. Angular.js provides a range of tools to help you write, update and test your code. Additionally, it provides many features such as routing and form management. This guide will discuss how to install Angular on Ubuntu24. First, you need to install Node.js. Node.js is a JavaScript running environment based on the ChromeV8 engine that allows you to run JavaScript code on the server side. To be in Ub
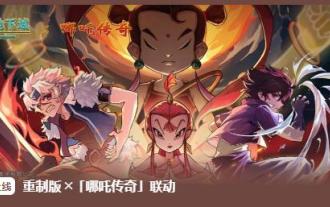
Lantern and Dungeons has been confirmed to be updated on February 29th. After the update, the remastered version of Lantern and Dungeons will be launched, and the remastered version will also be linked to the Legend of Nezha. The remastered version will also bring a new profession, and players can directly Job changes, dungeon content will also be expanded, new dungeon areas will be opened, etc. Mobile game update schedule Lantern and Dungeon updated on February 29th: Remastered version ╳ "Legend of Nezha" linkage version key content New profession, why are you invited to change jobs? Lamplighters can actually change jobs? Such cool equipment is really It makes people greedy. I heard that after changing jobs, the lantern holder can also learn many cool skills. Goro exclaimed: Thai pants are hot! The Legend of Nezha is coming together! Stepping on the hot wheel, holding the circle of heaven and earth in hand ♫ ~ The little heroes with both wisdom and courage: Nezha and Little Dragon Girl are about to come
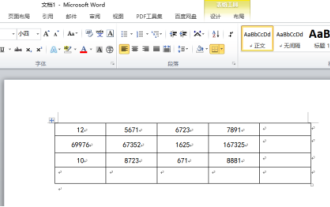
Sometimes, we often encounter counting problems in Word tables. Generally, when encountering such problems, most students will copy the Word table to Excel for calculation; some students will silently pick up the calculator. Calculate. Is there a quick way to calculate it? Of course there is, in fact the sum can also be calculated in Word. So, do you know how to do it? Today, let’s take a look together! Without further ado, friends in need should quickly collect it! Step details: 1. First, we open the Word software on the computer and open the document that needs to be processed. (As shown in the picture) 2. Next, we position the cursor on the cell where the summed value is located (as shown in the picture); then, we click [Menu Bar
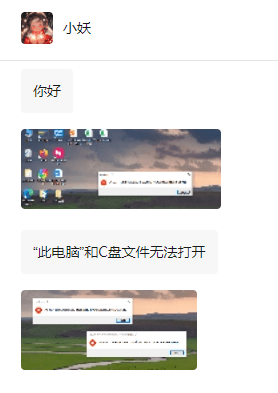
A friend's computer has such a fault. When opening "This PC" and the C drive file, it will prompt "Explorer.EXE Windows cannot access the specified device, path or file. You may not have the appropriate permissions to access the project." Including folders, files, This computer, Recycle Bin, etc., double-clicking will pop up such a window, and right-clicking to open it is normal. This is caused by a system update. If you also encounter this situation, the editor below will teach you how to solve it. 1. Open the registry editor Win+R and enter regedit, or right-click the start menu to run and enter regedit; 2. Locate the registry "Computer\HKEY_CLASSES_ROOT\PackagedCom\ClassInd"
