


Discuss the development prospects of Golang in the field of cloud computing
Golang (also known as Go language), as a programming language with high development efficiency and excellent performance, has been receiving widespread attention and application. In the field of cloud computing, Golang has shown considerable potential. Its concurrency model, memory management and performance advantages make it a popular choice for cloud native application development. This article will start from the technical level and analyze the potential and advantages of Golang in the field of cloud computing through specific code examples.
Concurrency model
Golang implements a simple and powerful concurrency model with its built-in goroutine and channel mechanisms, which can better utilize multi-core processors and improve the concurrency capabilities of the program. In cloud computing, when a large number of concurrent requests need to be processed, Golang's concurrency model can handle tasks more efficiently and improve system performance and throughput.
package main import ( "fmt" "time" ) func worker(id int, jobs <-chan int, results chan<- int) { for j := range jobs { fmt.Printf("Worker %d processing job %d ", id, j) time.Sleep(time.Second) results <- j * 2 } } func main() { jobs := make(chan int, 5) results := make(chan int, 5) for w := 1; w <= 3; w++ { go worker(w, jobs, results) } for j := 1; j <= 5; j++ { jobs <- j } close(jobs) for a := 1; a <= 5; a++ { <-results } }
In the above example, we created 3 worker goroutines to process 5 tasks concurrently. Each task takes 1 second, and the task can be completed faster through goroutine processing at the same time. This concurrency model can be well applied to scenarios of handling large-scale requests in cloud computing.
Memory Management
Golang has the feature of automatic memory management, which can effectively prevent memory leaks through the garbage collection mechanism and reduce the burden on developers. In cloud computing, it is very important to ensure system stability and resource utilization. Golang's memory management can help developers better optimize and maintain the system.
package main import "fmt" func main() { s := make([]int, 0, 10) fmt.Println("Capacity:", cap(s)) // 输出 Capacity: 10 for i := 0; i < 20; i++ { s = append(s, i) if cap(s) != len(s) { newS := make([]int, len(s)) copy(newS, s) s = newS } } fmt.Println("Final slice:", s) }
In this example, we show how to continuously append elements to a slice in a loop and reallocate memory space when needed. Golang's built-in functions make
and append
can help developers manage memory more conveniently and avoid problems such as memory leaks.
Performance advantages
Golang has excellent performance in terms of performance. The compiled program runs quickly and consumes less resources. In a cloud computing environment, high performance is crucial. Golang can meet scenarios with high performance requirements and ensure rapid system response and efficient use of resources.
package main import ( "fmt" "time" ) func fib(n int) int { if n <= 1 { return n } return fib(n-1) + fib(n-2) } func main() { start := time.Now() result := fib(40) // 计算第40个斐波那契数 elapsed := time.Since(start) fmt.Printf("Result: %d ", result) fmt.Printf("Time taken: %s ", elapsed) }
In the above example, we calculated the 40th Fibonacci number using recursion. Through Golang's efficient performance, this computing task can be completed in a relatively short time, demonstrating its advantages in the field of cloud computing.
To sum up, Golang, as an efficient and excellent programming language with excellent concurrency performance, has huge potential in the field of cloud computing. Through its rich features and advantages, it can help developers better improve system performance, stability and efficiency in cloud native application development. I hope the code examples in this article can help readers better understand the application and potential of Golang in the field of cloud computing.
The above is the detailed content of Discuss the development prospects of Golang in the field of cloud computing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

According to news from this site on July 31, technology giant Amazon sued Finnish telecommunications company Nokia in the federal court of Delaware on Tuesday, accusing it of infringing on more than a dozen Amazon patents related to cloud computing technology. 1. Amazon stated in the lawsuit that Nokia abused Amazon Cloud Computing Service (AWS) related technologies, including cloud computing infrastructure, security and performance technologies, to enhance its own cloud service products. Amazon launched AWS in 2006 and its groundbreaking cloud computing technology had been developed since the early 2000s, the complaint said. "Amazon is a pioneer in cloud computing, and now Nokia is using Amazon's patented cloud computing innovations without permission," the complaint reads. Amazon asks court for injunction to block
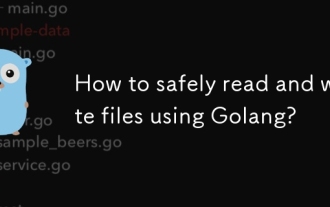
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
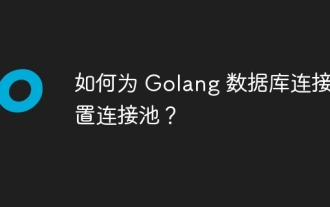
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
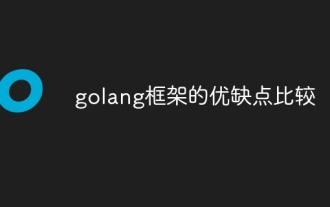
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
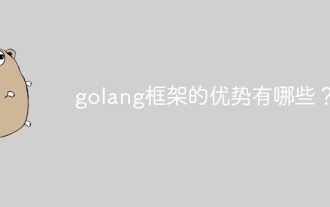
Advantages of the Golang Framework Golang is a high-performance, concurrent programming language that is particularly suitable for microservices and distributed systems. The Golang framework makes developing these applications easier by providing a set of ready-made components and tools. Here are some of the key advantages of the Golang framework: 1. High performance and concurrency: Golang itself is known for its high performance and concurrency. It uses goroutines, a lightweight threading mechanism that allows concurrent execution of code, thereby improving application throughput and responsiveness. 2. Modularity and reusability: Golang framework encourages modularity and reusable code. By breaking the application into independent modules, you can easily maintain and update the code
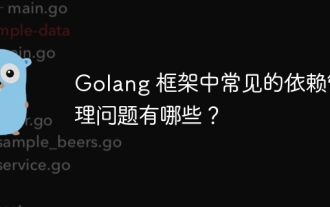
Common problems and solutions in Go framework dependency management: Dependency conflicts: Use dependency management tools, specify the accepted version range, and check for dependency conflicts. Vendor lock-in: Resolved by code duplication, GoModulesV2 file locking, or regular cleaning of the vendor directory. Security vulnerabilities: Use security auditing tools, choose reputable providers, monitor security bulletins and keep dependencies updated.
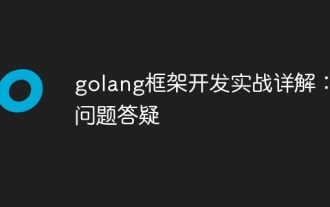
In Go framework development, common challenges and their solutions are: Error handling: Use the errors package for management, and use middleware to centrally handle errors. Authentication and authorization: Integrate third-party libraries and create custom middleware to check credentials. Concurrency processing: Use goroutines, mutexes, and channels to control resource access. Unit testing: Use gotest packages, mocks, and stubs for isolation, and code coverage tools to ensure sufficiency. Deployment and monitoring: Use Docker containers to package deployments, set up data backups, and track performance and errors with logging and monitoring tools.
