Best practices of Java Jersey framework to make your RESTful API better
- Use annotations: Jersey Framework provides a wealth of annotations to simplify the development of RESTful api. For example, the @Path annotation is used to specify the path of the resource, the @GET, @POST, @PUT, and @DELETE annotations are used to specify the Http method of the resource, and the @Produces and @Consumes annotations are used to specify the media of the resource. type. Using these annotations can make the code more concise and easier to maintain.
@Path("/users") public class UserResource { @GET @Produces(MediaType.APPLICATioN_JSON) public List<User> getAllUsers() { return userService.getAllUsers(); } @POST @Consumes(MediaType.APPLICATION_jsON) public User createUser(User user) { return userService.createUser(user); } @PUT @Path("/{id}") @Consumes(MediaType.APPLICATION_JSON) public User updateUser(@PathParam("id") Long id, User user) { return userService.updateUser(id, user); } @DELETE @Path("/{id}") public void deleteUser(@PathParam("id") Long id) { userService.deleteUser(id); } }
- Using filters: The Jersey framework provides filters for processing data during request and response processing. For example, you can use filters to verify the permissions of requests, log
- of requests, or compress requests and responses.
public class LoggingFilter implements Filter { @Override public void doFilter(FilterChain chain, Request request, Response response) throws IOException, ServletException { long startTime = System.currentTimeMillis(); chain.doFilter(request, response); long endTime = System.currentTimeMillis(); logger.info("Request {} {} took {} ms", request.getMethod(), request.getPath(), endTime - startTime); } }
- Use resource configuration: The Jersey framework provides resource configuration classes for configuring resource attributes. For example, you can use the resource configuration class to specify the media type of the resource,
- caching policy, configuration of cross-domain requests, etc.
public class UserResourceConfig extends ResourceConfig { public UserResourceConfig() { reGISter(UserResource.class); register(LoggingFilter.class); } }
- Use dependency injection: The Jersey framework supports dependency injection, which can make the code more modular and easier to maintain. Services, DAOs, and other components can be injected into resources using dependency injection.
public class UserResource { @Inject private UserService userService; @GET @Produces(MediaType.APPLICATION_JSON) public List<User> getAllUsers() { return userService.getAllUsers(); } }
- Performance Optimization: When developing RESTful API, performance Optimization is very important. The following methods can be used to optimize the performance of the API:
- Use caching: You can use caching to reduce the number of accesses to
- the database or other slow resources. Use compression: You can use compression to reduce the response size of the API.
- Use asynchronous processing: You can use asynchronous processing to improve the
- concurrency of the API.
- Security Security: Security is also very important when developing RESTful APIs. You can use the following methods to improve the security of your API:
- Using
- https: HTTPS can be used to encrypt requests and responses. Use authentication: You can use authentication to verify the legitimacy of the request.
- Use authorization: You can use authorization to control access to resources.
>Soft Exam Advanced Exam Preparation Skills/Past Exam Questions/Preparation Essence Materials" target="_blank">Click to download for free>>Soft Exam Advanced Exam Preparation Skills/Past Exam Questions/Exam Preparation Essence Materials
The above is the detailed content of Best practices of Java Jersey framework to make your RESTful API better. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
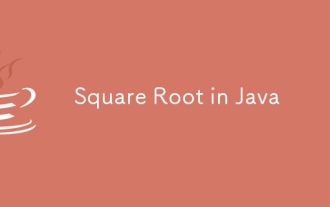
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
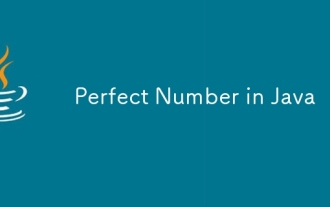
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
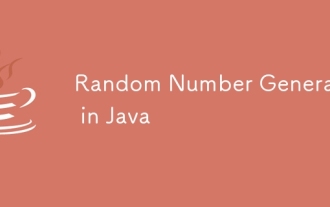
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
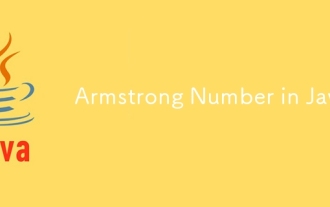
Guide to the Armstrong Number in Java. Here we discuss an introduction to Armstrong's number in java along with some of the code.
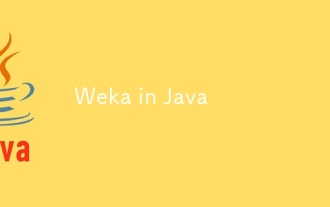
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
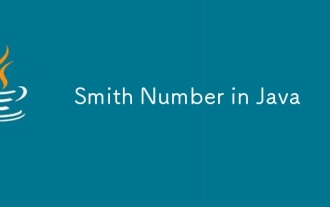
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
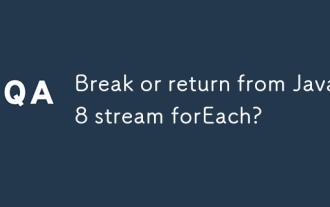
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
