Analysis of application scenarios of $stmt php in programming
Title: Analysis and examples of application scenarios of the $stmt object in PHP in programming
The $stmt object (Statement Object) in PHP is PDO (PHP Data Object) extension library is an important tool for executing prepared statements, which can improve the security and efficiency of database operations. In actual programming, the $stmt object has a wide range of application scenarios. This article will analyze and explain its specific applications in various situations.
1. Basic usage of $stmt object
The basic usage of $stmt object includes steps such as preparation of prepared statements, binding of parameters, executing statements and obtaining results. The following is a simple query example:
<?php $pdo = new PDO("mysql:host=localhost;dbname=test", "username", "password"); $stmt = $pdo->prepare("SELECT * FROM users WHERE id = :id"); $stmt->bindParam(':id', $id, PDO::PARAM_INT); $id = 1; $stmt->execute(); $result = $stmt->fetchAll(PDO::FETCH_ASSOC); print_r($result); ?>
In the above code, first create a pdo object through the PDO class, then use the prepare() method to prepare a query statement, bind the parameter: id, and execute the query . Finally, use the fetchAll() method to obtain the query results.
2. Batch operations of $stmt objects
In addition to single queries, $stmt objects can also be used for batch operations, such as batch inserts, updates, deletes, etc. The following takes batch insertion as an example:
<?php $pdo = new PDO("mysql:host=localhost;dbname=test", "username", "password"); $stmt = $pdo->prepare("INSERT INTO users (name, email) VALUES (:name, :email)"); $users = [ ['Alice', 'alice@example.com'], ['Bob', 'bob@example.com'], ['Eve', 'eve@example.com'] ]; foreach ($users as $user) { $stmt->bindParam(':name', $user[0]); $stmt->bindParam(':email', $user[1]); $stmt->execute(); } echo "批量插入成功!"; ?>
In the above code, an insert statement is first prepared, and then the parameters of each record are bound in a loop and the insertion operation is performed, thereby achieving the effect of batch insertion.
3. Transaction processing of $stmt object
The $stmt object can also be combined with transaction processing to ensure the atomicity of database operations and ensure that multiple operations either all succeed or all fail. The following takes transaction processing as an example:
<?php $pdo = new PDO("mysql:host=localhost;dbname=test", "username", "password"); $pdo->beginTransaction(); try { $pdo->exec("UPDATE users SET balance = balance - 100 WHERE id = 1"); $pdo->exec("UPDATE users SET balance = balance + 100 WHERE id = 2"); $pdo->commit(); echo "转账成功!"; } catch (Exception $e) { $pdo->rollback(); echo "转账失败:" . $e->getMessage(); } ?>
In the above code, the transaction is started through the beginTransaction() method, and then two update statements are executed in sequence, and the transaction is submitted after successful execution; if any of the operations fails, return Rolls the transaction and outputs an error message.
Conclusion
Through the above examples, we can see the flexible application of $stmt objects in various scenarios, which can improve the security and efficiency of database operations. In actual development, mastering the usage of the $stmt object will be of great benefit to improving programming efficiency and code quality. I hope the content of this article can inspire readers and deepen their understanding and use of the $stmt object in PHP programming.
The above is the detailed content of Analysis of application scenarios of $stmt php in programming. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


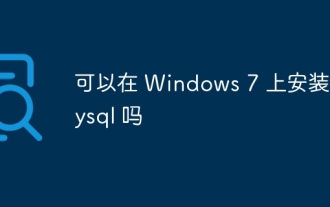
Yes, MySQL can be installed on Windows 7, and although Microsoft has stopped supporting Windows 7, MySQL is still compatible with it. However, the following points should be noted during the installation process: Download the MySQL installer for Windows. Select the appropriate version of MySQL (community or enterprise). Select the appropriate installation directory and character set during the installation process. Set the root user password and keep it properly. Connect to the database for testing. Note the compatibility and security issues on Windows 7, and it is recommended to upgrade to a supported operating system.
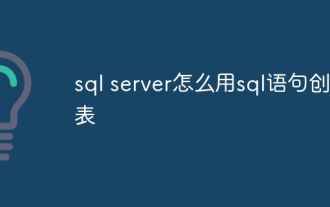
How to create tables using SQL statements in SQL Server: Open SQL Server Management Studio and connect to the database server. Select the database to create the table. Enter the CREATE TABLE statement to specify the table name, column name, data type, and constraints. Click the Execute button to create the table.
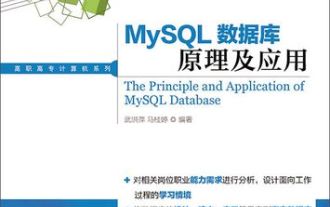
The article introduces the operation of MySQL database. First, you need to install a MySQL client, such as MySQLWorkbench or command line client. 1. Use the mysql-uroot-p command to connect to the server and log in with the root account password; 2. Use CREATEDATABASE to create a database, and USE select a database; 3. Use CREATETABLE to create a table, define fields and data types; 4. Use INSERTINTO to insert data, query data, update data by UPDATE, and delete data by DELETE. Only by mastering these steps, learning to deal with common problems and optimizing database performance can you use MySQL efficiently.
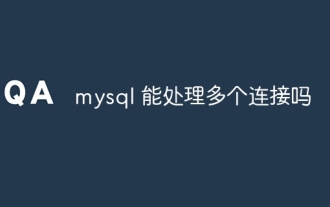
MySQL can handle multiple concurrent connections and use multi-threading/multi-processing to assign independent execution environments to each client request to ensure that they are not disturbed. However, the number of concurrent connections is affected by system resources, MySQL configuration, query performance, storage engine and network environment. Optimization requires consideration of many factors such as code level (writing efficient SQL), configuration level (adjusting max_connections), hardware level (improving server configuration).
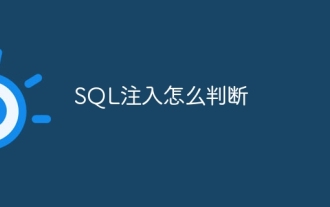
Methods to judge SQL injection include: detecting suspicious input, viewing original SQL statements, using detection tools, viewing database logs, and performing penetration testing. After the injection is detected, take measures to patch vulnerabilities, verify patches, monitor regularly, and improve developer awareness.
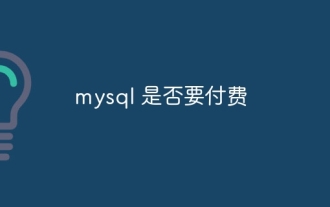
MySQL has a free community version and a paid enterprise version. The community version can be used and modified for free, but the support is limited and is suitable for applications with low stability requirements and strong technical capabilities. The Enterprise Edition provides comprehensive commercial support for applications that require a stable, reliable, high-performance database and willing to pay for support. Factors considered when choosing a version include application criticality, budgeting, and technical skills. There is no perfect option, only the most suitable option, and you need to choose carefully according to the specific situation.
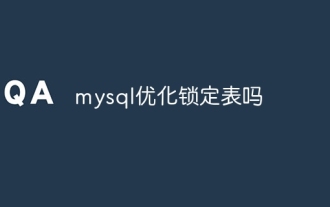
MySQL uses shared locks and exclusive locks to manage concurrency, providing three lock types: table locks, row locks and page locks. Row locks can improve concurrency, and use the FOR UPDATE statement to add exclusive locks to rows. Pessimistic locks assume conflicts, and optimistic locks judge the data through the version number. Common lock table problems manifest as slow querying, use the SHOW PROCESSLIST command to view the queries held by the lock. Optimization measures include selecting appropriate indexes, reducing transaction scope, batch operations, and optimizing SQL statements.
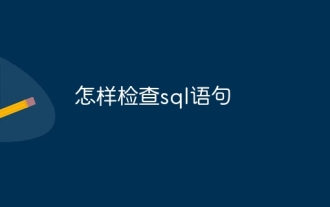
The methods to check SQL statements are: Syntax checking: Use the SQL editor or IDE. Logical check: Verify table name, column name, condition, and data type. Performance Check: Use EXPLAIN or ANALYZE to check indexes and optimize queries. Other checks: Check variables, permissions, and test queries.
