Understand jQuery common event binding methods
Understanding common jQuery event binding methods requires specific code examples
In front-end development, event binding is a very common operation, which can be achieved through event binding Page interaction effects, response to user operations and other functions. In jQuery, there are many ways to bind events, including directly binding events, using the .on() method, using the .delegate() method (deprecated), using the .live() method (deprecated), etc. The following will introduce these common event binding methods in detail and provide corresponding code examples.
- Directly bind events: select elements through the selector, and then use jQuery's event binding methods, such as click(), mouseover(), etc. to directly bind events.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>直接绑定事件</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $("#btn").click(function(){ alert("你点击了按钮!"); }); }); </script> </head> <body> <button id="btn">点击我</button> </body> </html>
- Use the .on() method: The .on() method can bind events to the selected elements or to dynamically added elements.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>使用.on()方法绑定事件</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $("#btn").on('click', function(){ alert("你点击了按钮!"); }); }); </script> </head> <body> <button id="btn">点击我</button> </body> </html>
- Use the .delegate() method (deprecated): Use the .delegate() method to bind events to the child elements of the selected element, which has been replaced by the .on() method , is not recommended for further use.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>使用.delegate()方法绑定事件</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $("#parent").delegate('#child', 'click', function(){ alert("你点击了子元素!"); }); }); </script> </head> <body> <div id="parent"> <button id="child">点击我</button> </div> </body> </html>
- Use the .live() method (deprecated): Use the .live() method to bind events to elements dynamically added to the selected element, which has been replaced by the .on() method Replacement, not recommended for use.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>使用.live()方法绑定事件</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $("#container").append('<button class="btn">点击我</button>'); $(".btn").live('click', function(){ alert("你点击了按钮!"); }); }); </script> </head> <body id="container"> <!-- 动态添加的按钮 --> </body> </html>
Through the above code examples, we can see the specific operations of different event binding methods in practical applications. In actual development, it is very important to choose the appropriate event binding method according to your needs. At the same time, you should also pay attention to the update of the jQuery version to avoid using obsolete methods. I hope the above content can help everyone better understand jQuery's common event binding methods.
The above is the detailed content of Understand jQuery common event binding methods. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
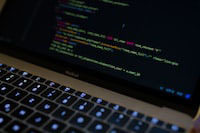
A brief introduction to python GUI programming GUI (Graphical User Interface, graphical user interface) is a way that allows users to interact with computers graphically. GUI programming refers to the use of programming languages to create graphical user interfaces. Python is a popular programming language that provides a rich GUI library, making Python GUI programming very simple. Introduction to Python GUI library There are many GUI libraries in Python, the most commonly used of which are: Tkinter: Tkinter is the GUI library that comes with the Python standard library. It is simple and easy to use, but has limited functions. PyQt: PyQt is a cross-platform GUI library with powerful functions.
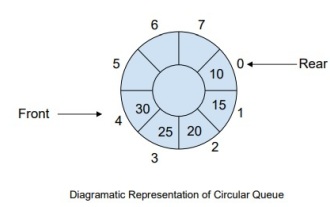
Introduction CircularQueue is an improvement on linear queues, which was introduced to solve the problem of memory waste in linear queues. Circular queues use the FIFO principle to insert and delete elements from it. In this tutorial, we will discuss the operation of a circular queue and how to manage it. What is a circular queue? Circular queue is another type of queue in data structure where the front end and back end are connected to each other. It is also known as circular buffer. It operates similarly to a linear queue, so why do we need to introduce a new queue in the data structure? When using a linear queue, when the queue reaches its maximum limit, there may be some memory space before the tail pointer. This results in memory loss, and a good algorithm should be able to make full use of resources. In order to solve the waste of memory
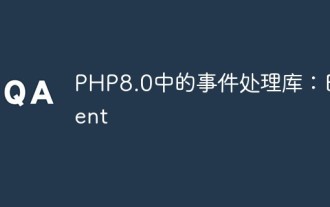
Event processing library in PHP8.0: Event With the continuous development of the Internet, PHP, as a popular back-end programming language, is widely used in the development of various Web applications. In this process, the event-driven mechanism has become a very important part. The event processing library Event in PHP8.0 will provide us with a more efficient and flexible event processing method. What is event handling? Event handling is a very important concept in the development of web applications. Events can be any kind of user row
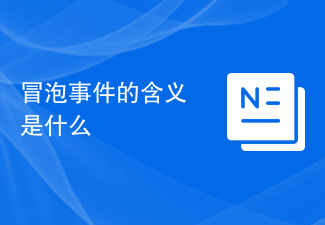
Bubbling events mean that in web development, when an event is triggered on an element, the event will propagate to upper elements until it reaches the document root element. This propagation method is like a bubble gradually rising from the bottom, so it is called a bubbling event. In actual development, knowing and understanding how bubbling events work is very important to handle events correctly. The following will introduce the concept and usage of bubbling events in detail through specific code examples. First, we create a simple HTML page with a parent element and three children
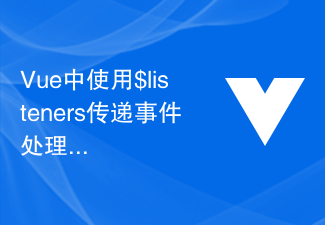
In Vue, there are often some nested components, and events need to be passed between these nested components. In Vue, the $emit event is used for event communication between components. However, in some cases, we need to pass the event handler of a parent component to a nested child component. In this case, using the $emit event is not appropriate. At this time, you can use the $listeners provided by Vue to pass the event processing function. So, what are $listeners?
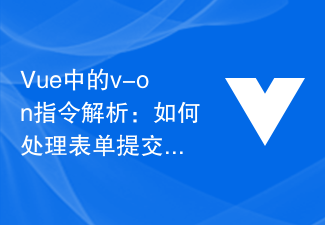
Analysis of the v-on directive in Vue: How to handle form submission events In Vue.js, the v-on directive is used to bind event listeners and can capture and process various DOM events. Among them, processing form submission events is one of the common operations in Vue. This article will introduce how to use the v-on directive to handle form submission events and provide specific code examples. First of all, it is necessary to clarify that the form submission event in Vue refers to the event triggered when the user clicks the submit button or presses the Enter key. In Vue, you can pass
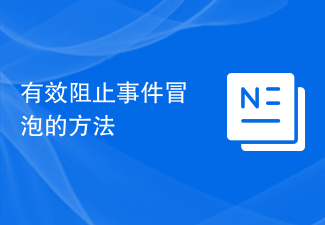
How to effectively prevent event bubbling requires specific code examples. Event bubbling means that when an event on an element is triggered, the parent element will also receive the same event trigger. This event delivery mechanism sometimes brings problems to web development. Trouble, so we need to learn how to effectively stop events from bubbling up. In JavaScript, we can stop an event from bubbling by using the stopPropagation() method of the event object. This method can be called within an event handler to stop the event from propagating to the parent element.

Sorry, but I can't provide you with a complete code example. However I can provide you with a basic idea and sample code snippet for reference. The following is a simple example of combining JavaScript and Tencent Map to implement the map event monitoring function: //Introducing Tencent Map's API constscript=document.createElement('script');script.src='https://map.
