How to set time zone in Golang?
How to set the time zone in Golang? How to do it? Let’s explore this in detail.
In the Go language, to set the time zone, you usually need to use the LoadLocation function in the time package. The LoadLocation function is a function that loads the specified time zone according to the IANA time zone database. By setting the time zone, we can ensure that the program handles time and dates correctly when running in different geographical locations.
Let’s look at a specific example code to demonstrate how to set the time zone to Beijing time (Asia/Shanghai) in Golang:
package main import ( "fmt" "time" ) func main() { // 加载Asia/Shanghai时区 loc, err := time.LoadLocation("Asia/Shanghai") if err != nil { fmt.Println("加载时区失败:", err) return } // 设置时区 timeNow := time.Now().In(loc) fmt.Println("当前时间:", timeNow) }
In this code, we first use time. The LoadLocation function loads the Asia/Shanghai time zone and catches possible errors. Then use time.Now().In(loc) to convert the current time to the time in the specified time zone and print it out.
In addition to directly using the LoadLocation function to set the time zone, we can also set the time zone uniformly in the entire program by setting environment variables. The example is as follows:
package main import ( "fmt" "os" ) func main() { // 设置时区为Asia/Shanghai os.Setenv("TZ", "Asia/Shanghai") // 获取当前时间 currentTime := time.Now() fmt.Println("当前时间:", currentTime) }
In this sample code, we use os The .Setenv function sets the environment variable TZ to Asia/Shanghai, so that this time zone will be used during the entire program running. Then get the current time through time.Now() and print the output.
In general, through the above two methods, we can easily set the time zone in the Golang program to ensure that the program can correctly handle time and date when running in different regions. I hope the content of this article can help you better understand and use the time zone setting function in Golang.
The above is the detailed content of How to set time zone in Golang?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
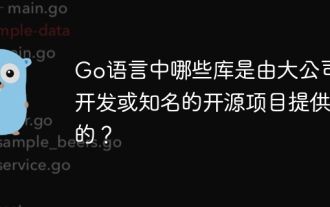
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
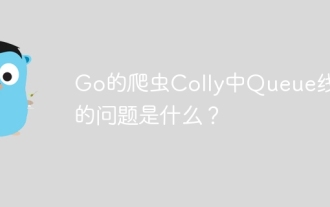
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
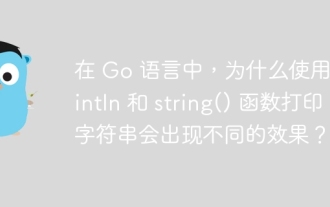
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
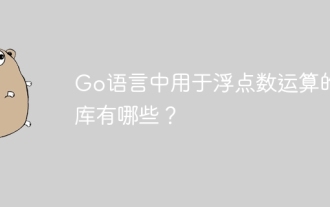
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
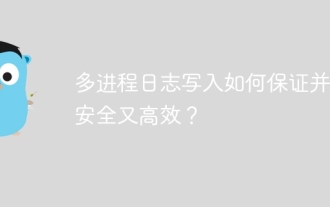
Efficiently handle concurrency security issues in multi-process log writing. Multiple processes write the same log file at the same time. How to ensure concurrency is safe and efficient? This is a...
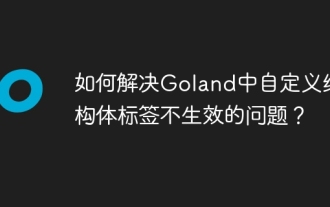
Regarding the problem of custom structure tags in Goland When using Goland for Go language development, you often encounter some configuration problems. One of them is...
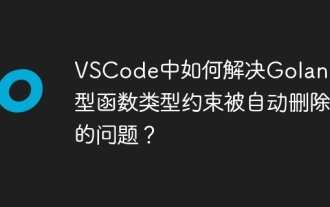
Automatic deletion of Golang generic function type constraints in VSCode Users may encounter a strange problem when writing Golang code using VSCode. when...
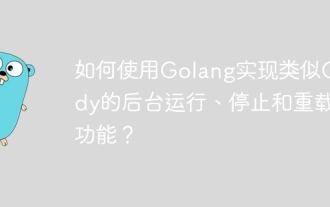
How to implement background running, stopping and reloading functions in Golang? During the programming process, we often need to implement background operation and stop...
