


Learn by doing: Best practices for object-oriented programming in Golang
Learn from practice: Best practices for object-oriented programming in Golang
As Golang (Go language) has become more and more widely used in recent years, more and more More and more developers are beginning to explore Golang’s object-oriented programming (OOP) features. Although Golang is a programming language designed with concurrency as its core, it is not a pure object-oriented language itself, but by flexibly using its features, we can still achieve good object-oriented programming practices. This article will explore some of the best practices for object-oriented programming in Golang and illustrate them with specific code examples.
1. Structures and methods
In Golang, we can use structures to define data structures and operate these data through methods. Structures can be thought of as replacements for classes in object-oriented programming, while methods can be thought of as functions in classes. The following is a simple example:
package main import "fmt" type Rectangle struct { width float64 height float64 } func (r Rectangle) Area() float64 { return r.width * r.height } func main() { rect := Rectangle{width: 10, height: 5} fmt.Println("Rectangle Area:", rect.Area()) }
In the above code, we define a Rectangle
structure, including width
and height
Two fields, and then define a Area
method for calculating the area of the rectangle. In the main
function, we instantiate a Rectangle
object and call its Area
method to calculate the area.
2. Interface
The interface in Golang is an abstract type that defines a set of methods. Any type that implements all methods defined in the interface implements the interface by default. Interfaces play a role in constraints and specifications in object-oriented programming, which can improve the flexibility and reusability of code. Here is a simple example:
package main import ( "fmt" ) type Shape interface { Area() float64 } type Rectangle struct { width float64 height float64 } func (r Rectangle) Area() float64 { return r.width * r.height } func PrintArea(s Shape) { fmt.Println("Shape Area:", s.Area()) } func main() { rect := Rectangle{width: 10, height: 5} PrintArea(rect) }
In the above code, we define an interface named Shape
, which contains an Area
method. Then, our Rectangle
structure implements the Area
method of the Shape
interface. Finally, in the main
function, we call the PrintArea
function and pass in the Rectangle
object as a parameter.
Through the above example, we can see the power of interfaces, how to make different types have Area
methods, which can be passed into the PrintArea
function for unification deal with.
3. Package organization
In actual development, we often encapsulate a set of related functions into a package, and implement code organization and reuse through the import of packages. In Golang, packages are the basic unit of code organization and reuse. Good package organization can improve the maintainability and readability of code. The following is a simple example:
Suppose we have a package named shapes
, which contains definitions and operation methods for different shapes:
package shapes type Shape interface { Area() float64 } type Rectangle struct { width float64 height float64 } func (r Rectangle) Area() float64 { return r.width * r.height }
We will The above code is saved in the shapes.go
file of the shapes
package. Then you can import and use the package in the main program like this:
package main import ( "fmt" "your_module_path/shapes" ) func main() { rect := shapes.Rectangle{width: 10, height: 5} fmt.Println("Rectangle Area:", rect.Area()) }
Through the above examples, we show the best practices of object-oriented programming in Golang, including the use of structures and methods, and the use of interfaces. Definition and implementation, as well as package organization and import. These practices can help developers better utilize the features of Golang for object-oriented programming and improve the maintainability and readability of the code. Hope this article helps you!
The above is the detailed content of Learn by doing: Best practices for object-oriented programming in Golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
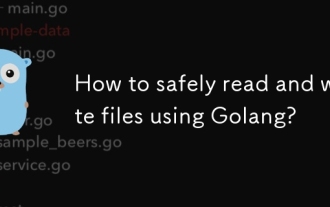
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
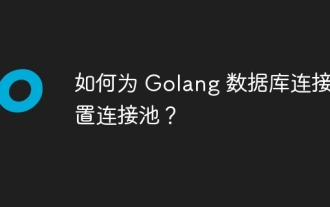
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
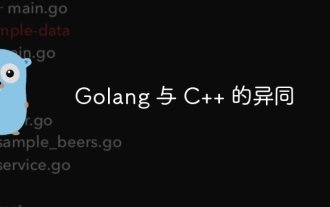
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
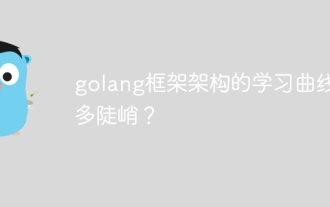
The learning curve of the Go framework architecture depends on familiarity with the Go language and back-end development and the complexity of the chosen framework: a good understanding of the basics of the Go language. It helps to have backend development experience. Frameworks that differ in complexity lead to differences in learning curves.
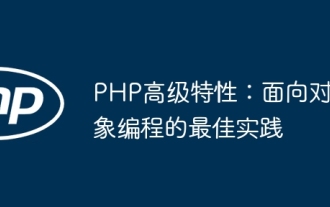
OOP best practices in PHP include naming conventions, interfaces and abstract classes, inheritance and polymorphism, and dependency injection. Practical cases include: using warehouse mode to manage data and using strategy mode to implement sorting.
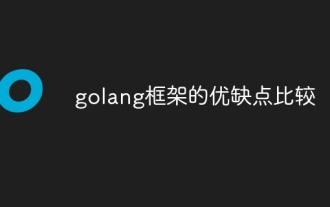
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
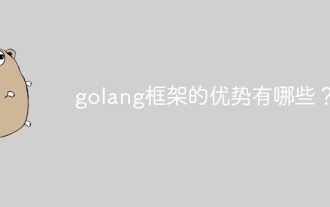
Advantages of the Golang Framework Golang is a high-performance, concurrent programming language that is particularly suitable for microservices and distributed systems. The Golang framework makes developing these applications easier by providing a set of ready-made components and tools. Here are some of the key advantages of the Golang framework: 1. High performance and concurrency: Golang itself is known for its high performance and concurrency. It uses goroutines, a lightweight threading mechanism that allows concurrent execution of code, thereby improving application throughput and responsiveness. 2. Modularity and reusability: Golang framework encourages modularity and reusable code. By breaking the application into independent modules, you can easily maintain and update the code
