Advantages and challenges of object-oriented programming in Golang
Golang is a programming language developed by Google that has unique advantages and challenges in object-oriented programming. This article will discuss the advantages and challenges of object-oriented programming in Golang, and illustrate it with specific code examples.
1. Advantages of Golang object-oriented programming
1. Simple and efficient
Golang adopts a concise syntax design, making the amount of code less and easy to maintain. Its static type system and compile-time type checking can find most errors during the compilation phase, effectively reducing the occurrence of bugs. The following is an example of a simple class and method:
type Person struct { Name string Age int } func (p *Person) SayHello() { fmt.Printf("Hello, my name is %s and I am %d years old. ", p.Name, p.Age) }
2. Concurrency support
Golang has built-in support for concurrent programming and provides mechanisms such as goroutine and channel, which can be easily implemented Multithreaded programming. This ability makes Golang suitable for handling high-concurrency scenarios and improves program performance. The following is an example of using goroutine:
func main() { go func() { fmt.Println("Hello from goroutine!") }() fmt.Println("Hello from main goroutine!") time.Sleep(1 * time.Second) }
2. Challenges of object-oriented programming in Golang
1. Change in object-oriented thinking
For those who are accustomed to traditional object-oriented programming languages For developers, Golang's object-oriented implementation may require a certain adaptation period. Golang does not have the concept of classes, but uses structures and methods to implement object behavior. The following is an example of using embedded structures:
type Animal struct { Name string } func (a *Animal) Speak() { fmt.Printf("%s makes a sound ", a.Name) } type Dog struct { Animal Breed string } func main() { dog := Dog{Animal{"Dog"}, "Labrador"} dog.Speak() }
2. Lack of inheritance and polymorphism
Golang does not have the concepts of inheritance and polymorphism in traditional object-oriented languages, which may limit Application of certain design patterns. Developers need to implement similar functions through a combination of interfaces. The following is a simple interface combination example:
type Speaker interface { Speak() } type Cat struct { Name string } func (c Cat) Speak() { fmt.Printf("%s says meow ", c.Name) } func main() { var speaker Speaker speaker = Cat{"Whiskers"} speaker.Speak() }
Conclusion
Although Golang has some unique advantages and challenges in object-oriented programming, by adapting and learning, developers can Develop using Golang features. Through the discussion and code examples in this article, I hope readers will have a certain understanding of Golang object-oriented programming and can better apply it in actual development.
The above is the detailed content of Advantages and challenges of object-oriented programming in Golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
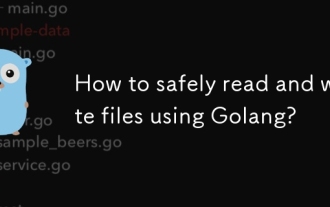
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
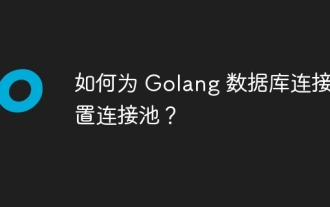
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
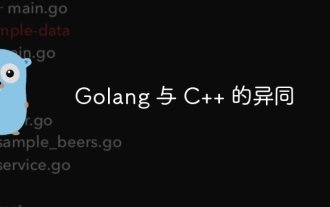
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
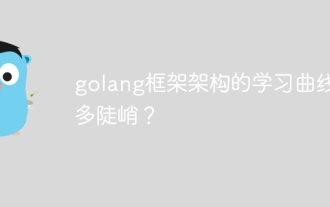
The learning curve of the Go framework architecture depends on familiarity with the Go language and back-end development and the complexity of the chosen framework: a good understanding of the basics of the Go language. It helps to have backend development experience. Frameworks that differ in complexity lead to differences in learning curves.
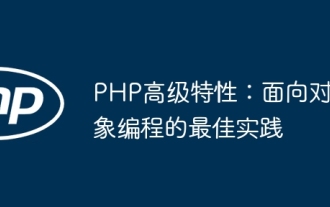
OOP best practices in PHP include naming conventions, interfaces and abstract classes, inheritance and polymorphism, and dependency injection. Practical cases include: using warehouse mode to manage data and using strategy mode to implement sorting.
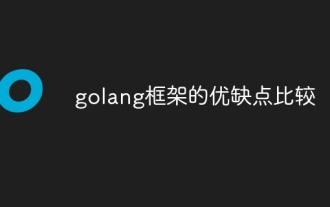
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
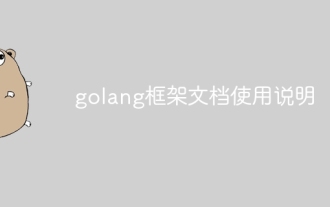
How to use Go framework documentation? Determine the document type: official website, GitHub repository, third-party resource. Understand the documentation structure: getting started, in-depth tutorials, reference manuals. Locate the information as needed: Use the organizational structure or the search function. Understand terms and concepts: Read carefully and understand new terms and concepts. Practical case: Use Beego to create a simple web server. Other Go framework documentation: Gin, Echo, Buffalo, Fiber.
