Best practices for asynchronous programming in Golang
Best Practices of Golang Asynchronous Programming
With the continuous development of Internet applications and services, the demand for efficient concurrent processing and asynchronous programming has become more and more important. urgent. In the Go language, you can also use features such as goroutines and channels to implement asynchronous programming. This article will introduce the best practices of asynchronous programming in Golang and provide some specific code examples.
1. Use goroutines to implement concurrent processing
In the Go language, goroutines are lightweight threads that can execute code blocks concurrently. Through goroutines, concurrent processing can be easily implemented and the performance of the program can be improved. The following is a simple sample code that shows how to use goroutines to execute tasks concurrently:
package main import ( "fmt" "time" ) func main() { for i := 1; i <= 3; i++ { go func(i int) { fmt.Println("Goroutine", i, "started") time.Sleep(time.Second) fmt.Println("Goroutine", i, "finished") }(i) } // 等待所有goroutine执行完成 time.Sleep(3 * time.Second) }
In the above code, we start 3 goroutines in a loop and output some information in each goroutine, and then Use time.Sleep
to wait for all goroutines to complete execution.
2. Use channels for data exchange
In asynchronous programming, data exchange between goroutines is often required. The Go language provides a data structure called a channel that can safely pass data between goroutines. The following is a simple example code that shows how to use channels for data exchange:
package main import "fmt" func main() { ch := make(chan int) go func() { ch <- 42 }() val := <-ch fmt.Println("Received value from channel:", val) }
In the above code, we create a channel of integer type and send a channel to the channel in a goroutine The integer value 42 is then received from the channel in the main goroutine and printed out.
3. Use sync.WaitGroup to manage goroutines
In actual asynchronous programming scenarios, it is often necessary to wait for a group of goroutines to be executed before proceeding to the next step. The Go language provides the WaitGroup type in the sync package, which can easily manage the execution of a group of goroutines. Here is a sample code that demonstrates how to use WaitGroup to wait for a group of goroutines to finish executing:
package main import ( "fmt" "sync" "time" ) func main() { var wg sync.WaitGroup for i := 1; i <= 3; i++ { wg.Add(1) go func(i int) { defer wg.Done() fmt.Println("Goroutine", i, "started") time.Sleep(time.Second) fmt.Println("Goroutine", i, "finished") }(i) } wg.Wait() fmt.Println("All goroutines finished") }
In the above code, we first call wg.Add(1)
to tell the WaitGroup that we are going to wait The number of goroutines is increased by 1, and then at the end of each goroutine, defer wg.Done()
is used to inform WaitGroup that the goroutine has been executed. Finally, wg.Wait()
waits for all goroutines to be executed.
Conclusion
Through the introduction and sample code of this article, I hope readers can master the best practices of Golang asynchronous programming and be able to flexibly use them in actual projects. In asynchronous programming, in addition to the goroutines, channels, and WaitGroup mentioned above, there are other concurrency primitives and patterns that can further improve program performance and maintainability. Readers can continue to study and practice in depth and explore more mysteries of asynchronous programming.
The above is the detailed content of Best practices for asynchronous programming in Golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
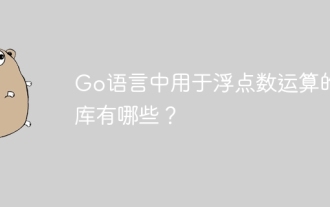
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
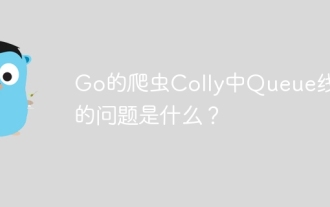
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
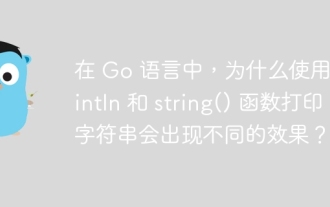
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
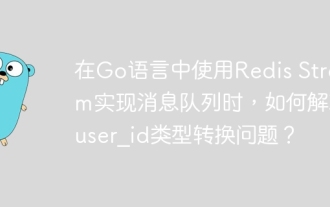
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
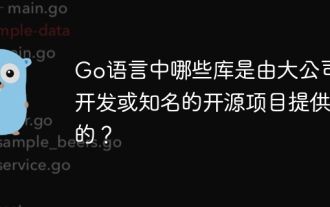
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
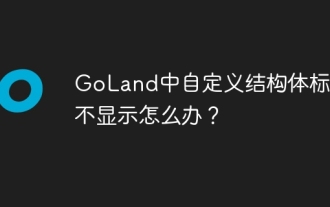
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
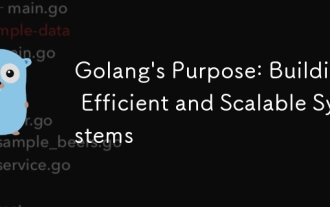
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
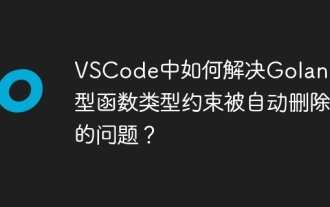
Automatic deletion of Golang generic function type constraints in VSCode Users may encounter a strange problem when writing Golang code using VSCode. when...
