Optimizing performance: Golang request handling tips
Optimizing performance: Golang request processing skills
With the popularity of Internet applications, the requirements for website and server performance are getting higher and higher. When processing a large number of requests, how to optimize the performance of the Go language has become an important issue. This article will introduce some techniques to improve Golang request processing performance and demonstrate them through specific code examples.
1. Use concurrent processing
The Go language inherently supports concurrency, and concurrent processing of requests can be implemented through goroutine and channel. When processing multiple requests, each request can be processed in a goroutine to improve concurrency performance. The following is a simple sample code:
package main import ( "fmt" "net/http" "time" ) func handler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, World!") } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) }
In the handler function that handles requests, you can use goroutine to process requests according to the actual situation, for example:
func handler(w http.ResponseWriter, r *http.Request) { go func() { // 处理请求 time.Sleep(1 * time.Second) fmt.Fprintf(w, "Hello, World!") }() }
By using goroutine to process requests concurrently, you can Improve the server's concurrency capability and speed up request response.
2. Use connection pool
When processing HTTP requests, you can use the connection pool to reduce the number of connection creation and destruction times and improve performance. The following is a sample code using a connection pool:
package main import ( "fmt" "net/http" "time" ) var httpClient = &http.Client{ Transport: &http.Transport{ MaxIdleConns: 100, MaxIdleConnsPerHost: 100, IdleConnTimeout: 90 * time.Second, }, } func handler(w http.ResponseWriter, r *http.Request) { resp, err := httpClient.Get("http://example.com") if err != nil { fmt.Fprintf(w, "Error: %v", err) return } defer resp.Body.Close() // 处理响应数据 } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) }
By using a connection pool, you can reuse connections when processing HTTP requests, avoid frequently creating and destroying connections, and improve performance and efficiency.
3. Use cache
When processing requests, you can use cache to store some calculation results, reduce repeated calculations, and improve performance. The following is a simple cache sample code:
package main import ( "fmt" "net/http" "sync" ) var cache = make(map[string]string) var mu sync.RWMutex func handler(w http.ResponseWriter, r *http.Request) { key := r.URL.Path mu.RLock() if val, ok := cache[key]; ok { fmt.Fprintf(w, "Cached Value: %s", val) mu.RUnlock() return } mu.RUnlock() mu.Lock() defer mu.Unlock() // 计算结果 result := "Hello, World!" cache[key] = result fmt.Fprintf(w, "New Value: %s", result) } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) }
By using cache, you can avoid repeated calculations and improve the speed and efficiency of request processing.
The above are some tips for improving Golang request processing performance. Through methods such as concurrent processing, connection pooling and caching, you can effectively optimize performance and improve the application's response speed and concurrency capabilities. Hope this article helps you!
The above is the detailed content of Optimizing performance: Golang request handling tips. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


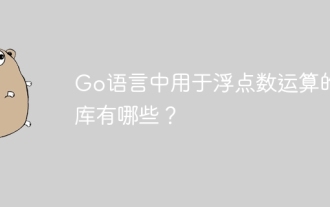
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
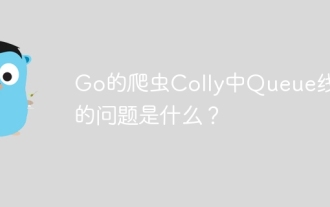
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
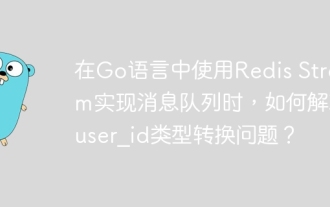
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
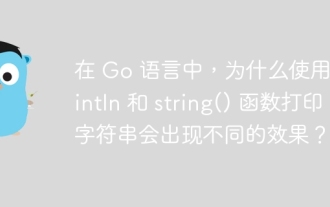
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
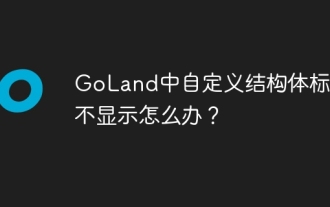
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
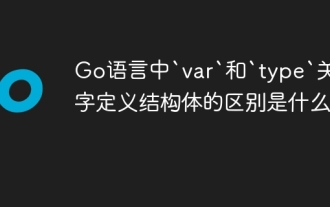
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
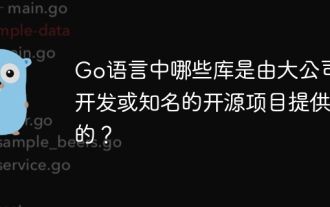
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
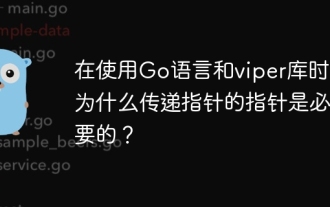
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
