


Detailed explanation of common functions of jQuery and sharing of development skills
jQuery is a popular JavaScript library that is widely used in web development. It simplifies the implementation of HTML document operations, event processing, animation effects and other functions, greatly improving development efficiency. This article will introduce in detail some commonly used functions in jQuery, share some development techniques, and provide specific code examples.
1. Selectors
Selectors are an important concept in jQuery, which allow developers to select elements on the page through CSS selectors. For example, to select the element with the id "example", you can use $("#example"); to select the element with the class "test", you can use $(".test"). The following are some commonly used selector examples:
$("#content") // 选择id为content的元素 $(".item") // 选择class为item的元素 $("ul li") // 选择ul下的所有li元素 $("input[type='text']") // 选择type为text的input元素
2. Event Handling
In jQuery, event handling functions can be used to manage user interaction with page elements. Common events include click, mouseenter, mouseleave, etc. The following is a simple example of click event processing:
$("#button").click(function(){ alert("按钮被点击了!"); });
3. Animation Effects
jQuery provides rich animation effects, which can allow page elements to achieve smooth dynamic effects. Common animation effects include fade in and out (fadeIn, fadeOut), slide (slideDown, slideUp), etc. The following is a simple fade-in effect example:
$("#box").fadeIn(1000);
4. AJAX Requests
Using jQuery, you can easily send AJAX requests and interact with the server-side data. You can use the $.ajax method to send a request and process the returned data in the callback function. The following is a simple AJAX request example:
$.ajax({ url: "example.php", type: "GET", success: function(data){ $("#result").html(data); } });
Development skills sharing:
- Chain call: jQuery supports chain calls, which can combine multiple operations Connected together to improve code simplicity and readability. For example:
$("#content").css("color", "red").fadeOut(1000).fadeIn(1000);
- Event delegation: You can use event delegation to reduce the number of event processing functions and improve page performance. By binding the event to the parent element, the event is processed based on its target. For example:
$("ul").on("click", "li", function(){ alert($(this).text()); });
- Optimize performance: When using jQuery, pay attention to performance optimization and avoid frequent DOM operations and use of selectors. You can use variables to cache selector results to reduce repeated searches.
To sum up, jQuery is a powerful and easy-to-use JavaScript library that can help developers easily implement various dynamic effects and interactive functions. Through the introduction and sample code of this article, I hope readers can become more proficient in using jQuery for Web development and play a greater role in the project.
The above is the detailed content of Detailed explanation of common functions of jQuery and sharing of development skills. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


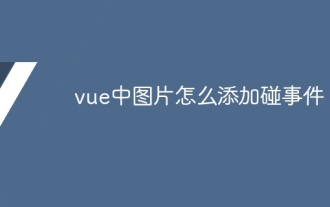
How to add click event to image in Vue? Import the Vue instance. Create a Vue instance. Add images to HTML templates. Add click events using the v-on:click directive. Define the handleClick method in the Vue instance.
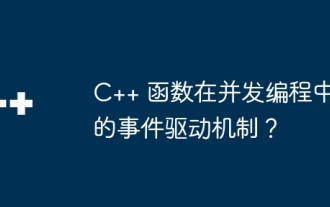
The event-driven mechanism in concurrent programming responds to external events by executing callback functions when events occur. In C++, the event-driven mechanism can be implemented with function pointers: function pointers can register callback functions to be executed when events occur. Lambda expressions can also implement event callbacks, allowing the creation of anonymous function objects. The actual case uses function pointers to implement GUI button click events, calling the callback function and printing messages when the event occurs.
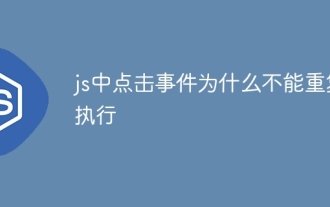
Click events in JavaScript cannot be executed repeatedly because of the event bubbling mechanism. To solve this problem, you can take the following measures: Use event capture: Specify an event listener to fire before the event bubbles up. Handing over events: Use event.stopPropagation() to stop event bubbling. Use a timer: trigger the event listener again after some time.
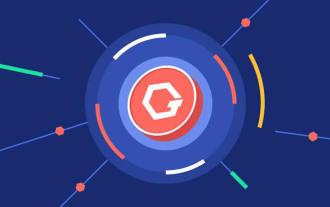
What is GateToken(GT) currency? GT (GateToken) is the native asset on the GateChain chain and the official platform currency of Gate.io. The value of GT coins is closely related to the development of Gate.io and GateChain ecology. What is GateChain? GateChain was born in 2018 and is a new generation of high-performance public chain launched by Gate.io. GateChain focuses on protecting the security of users' on-chain assets and providing convenient decentralized transaction services. GateChain's goal is to build an enterprise-level secure and efficient decentralized digital asset storage, distribution and transaction ecosystem. Gatechain has original
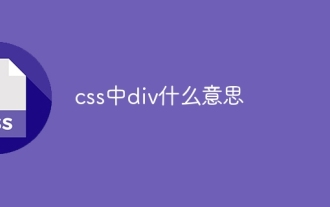
A DIV in CSS is a document separator or container used for grouping content, creating layouts, adding style, and interactivity. In HTML, the DIV element uses the syntax <div></div>, where div represents an element to which attributes and content can be added. DIV is a block-level element that occupies an entire line in the browser.
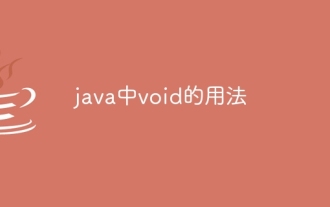
void in Java means that the method does not return any value and is often used to perform operations or initialize objects. The declaration format of void method is: void methodName(), and the calling method is methodName(). The void method is often used for: 1. Performing operations without returning a value; 2. Initializing objects; 3. Performing event processing operations; 4. Coroutines.
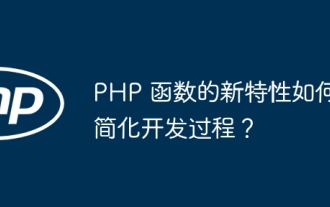
The new features of PHP functions greatly simplify the development process, including: Arrow function: Provides concise anonymous function syntax to reduce code redundancy. Property type declaration: Specify types for class properties, enhance code readability and reliability, and automatically perform type checking at runtime. null operator: concisely checks and handles null values, can be used to handle optional parameters.
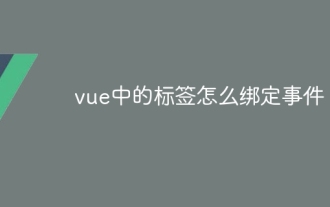
Use the v-on directive in Vue.js to bind label events. The steps are as follows: Select the label to which the event is to be bound. Use the v-on directive to specify the event type and how to handle the event. Specify the Vue method to call in the directive value.
