Common operation methods of jQuery objects
jQuery is a JavaScript library widely used in front-end development. It provides a wealth of operation methods to simplify tasks such as DOM operations, event processing, and animation effects. In this article, we will introduce some common jQuery object manipulation methods, and attach specific code examples for reference.
1. Create a jQuery object
In jQuery, you can create a jQuery object in several different ways:
- Select elements through a selector:
// 选取所有 class 为 .myClass 的元素 var $elements = $('.myClass'); // 选取 id 为 #myId 的元素 var $element = $('#myId');
- Directly pass in DOM elements to create jQuery objects:
var $element = $(document.getElementById('myElement'));
2. Common jQuery object operation methods
- css () method: used to set or get the style attributes of the element.
// 设置元素背景色为红色 $element.css('background-color', 'red'); // 获取元素宽度 var width = $element.css('width');
- addClass() and removeClass() methods: Class names used to add or remove elements.
// 添加一个新的类 $element.addClass('newClass'); // 移除一个类 $element.removeClass('oldClass');
- attr() and prop() methods: used to set or get the attributes of the element.
// 设置元素的 src 属性 $element.attr('src', 'newImage.jpg'); // 获取元素的 value 属性 var value = $element.prop('value');
- val() method: used to set or get the value of the form element.
// 设置 input 元素的值 $('input').val('new value'); // 获取 select 元素的值 var selectedValue = $('select').val();
- on() and off() methods: used to bind or unbind event handling functions.
// 绑定点击事件处理函数 $element.on('click', function() { alert('Clicked!'); }); // 解绑点击事件处理函数 $element.off('click');
- animate() method: used to achieve animation effects of elements.
// 实现元素移动到指定位置 $element.animate({left: '100px', top: '100px'}, 1000);
These are common object manipulation methods in jQuery, through which you can quickly and easily operate on page elements. I hope the above code examples can help readers better understand how to use jQuery.
The above is the detailed content of Common operation methods of jQuery objects. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


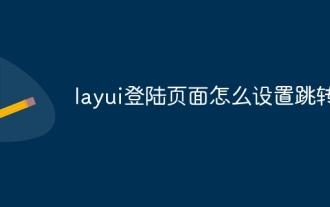
Layui login page jump setting steps: Add jump code: Add judgment in the login form submit button click event, and jump to the specified page through window.location.href after successful login. Modify the form configuration: add a hidden input field to the form element of lay-filter="login", with the name "redirect" and the value being the target page address.
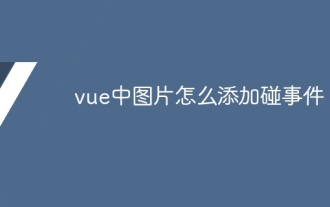
How to add click event to image in Vue? Import the Vue instance. Create a Vue instance. Add images to HTML templates. Add click events using the v-on:click directive. Define the handleClick method in the Vue instance.
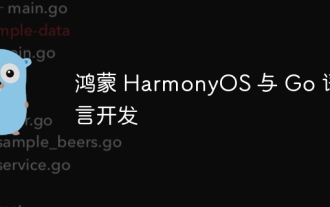
Introduction to HarmonyOS and Go language development HarmonyOS is a distributed operating system developed by Huawei, and Go is a modern programming language. The combination of the two provides a powerful solution for developing distributed applications. This article will introduce how to use Go language for development in HarmonyOS, and deepen understanding through practical cases. Installation and Setup To use Go language to develop HarmonyOS applications, you need to install GoSDK and HarmonyOSSDK first. The specific steps are as follows: #Install GoSDKgogetgithub.com/golang/go#Set PATH
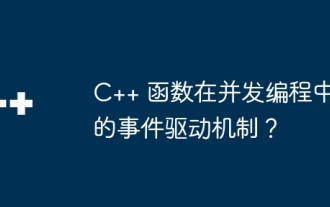
The event-driven mechanism in concurrent programming responds to external events by executing callback functions when events occur. In C++, the event-driven mechanism can be implemented with function pointers: function pointers can register callback functions to be executed when events occur. Lambda expressions can also implement event callbacks, allowing the creation of anonymous function objects. The actual case uses function pointers to implement GUI button click events, calling the callback function and printing messages when the event occurs.

Java is a popular programming language with powerful file handling capabilities. In Java, traversing a folder and getting all file names is a common operation, which can help us quickly locate and process files in a specific directory. This article will introduce how to implement a method of traversing a folder and getting all file names in Java, and provide specific code examples. 1. Use the recursive method to traverse the folder. We can use the recursive method to traverse the folder. The recursive method is a way of calling itself, which can effectively traverse the folder.
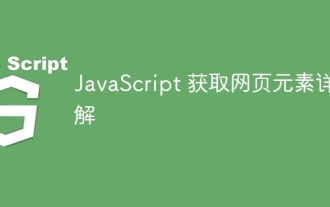
Answer: JavaScript provides a variety of methods for obtaining web page elements, including using ids, tag names, class names, and CSS selectors. Detailed description: getElementById(id): Get elements based on unique id. getElementsByTagName(tag): Gets the element group with the specified tag name. getElementsByClassName(class): Gets the element group with the specified class name. querySelector(selector): Use CSS selector to get the first matching element. querySelectorAll(selector): Get all matches using CSS selector
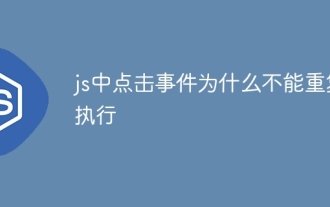
Click events in JavaScript cannot be executed repeatedly because of the event bubbling mechanism. To solve this problem, you can take the following measures: Use event capture: Specify an event listener to fire before the event bubbles up. Handing over events: Use event.stopPropagation() to stop event bubbling. Use a timer: trigger the event listener again after some time.
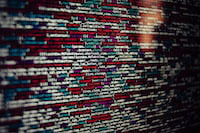
Tkinter is a powerful GUI toolkit in the Python standard library for creating cross-platform graphical user interfaces (GUIs). It is based on the Tcl/Tk toolkit and provides simple and intuitive syntax, allowing Python developers to create complex user interfaces easily and quickly. Advantages of Tkinter Cross-platform compatibility: Tkinter applications run on all major operating systems such as windows, Mac and Linux. Easy to use: Its syntax is clear and easy to learn, making it easy for both beginners and experienced developers to master. Extensibility: Tkinter provides a variety of widgets and controls, enabling developers to create a wide variety of user interfaces. Integration: It is related to P
