


How to install and configure PHP on Ubuntu system to connect to MSSQL database
Installing and configuring PHP on an Ubuntu system to connect to an MSSQL database is a common task, especially when developing web applications. In this article, we will introduce how to install PHP, MSSQL extensions and configure database connections on Ubuntu systems, while providing specific code examples.
Step 1: Install PHP and MSSQL extensions
-
Install PHP
First, you need to make sure that PHP is installed on the Ubuntu system. You can install PHP through the following command:sudo apt update sudo apt install php
Copy after login Install MSSQL extension
Next, you need to install the MSSQL extension of PHP to connect to the MSSQL database. You can use the following command to install:sudo apt install php-mssql
Copy after login
Step 2: Configure MSSQL connection
Edit the php.ini file
Open the PHP configuration file php.ini, you can use the following command:sudo nano /etc/php/7.x/apache2/php.ini
Copy after loginAdd the following line in the php.ini file to enable the MSSQL extension:
extension=php_mssql.so
Copy after loginRestart the Apache service
After saving and exiting the php.ini file, restart the Apache service to make the changes take effect:sudo service apache2 restart
Copy after login
Step 3: Connect to the MSSQL database
Now you can connect to MSSQL database using PHP code. Here is a simple example code:
<?php $serverName = "localhost"; $connectionOptions = array("Database" => "your_database", "Uid" => "your_username", "PWD" => "your_password"); // 通过sqlsrv_connect()函数连接数据库 $conn = sqlsrv_connect($serverName, $connectionOptions); if ($conn) { echo "Connection established. "; } else { echo "Connection could not be established. "; die(print_r(sqlsrv_errors(), true)); } // 查询数据 $sql = "SELECT * FROM your_table"; $stmt = sqlsrv_query($conn, $sql); if ($stmt === false) { die(print_r(sqlsrv_errors(), true)); } while ($row = sqlsrv_fetch_array($stmt, SQLSRV_FETCH_ASSOC)) { echo $row['column_name'] . " "; } sqlsrv_free_stmt($stmt); sqlsrv_close($conn); ?>
Make sure to replace "localhost", "your_database", "your_username" and "your_password" in the example with your actual hostname, database name, username and password. The above code demonstrates the process of connecting to an MSSQL database and executing a simple query.
Through the above steps, you can successfully install and configure PHP on the Ubuntu system to connect to the MSSQL database, and use the provided code samples to perform database connection and query operations. Hope this article helps you!
The above is the detailed content of How to install and configure PHP on Ubuntu system to connect to MSSQL database. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


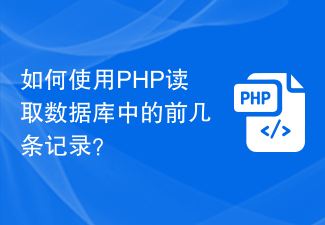
How to read the first few records in a database using PHP? When developing web applications, we often need to read data from the database and display it to the user. Sometimes, we only need to display the first few records in the database, not the entire content. This article will teach you how to use PHP to read the first few records in the database and provide specific code examples. First, assume that you have connected to the database and selected the table you want to operate on. The following is a simple database connection example:
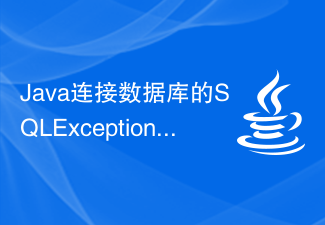
In Java programs, connecting to the database is a very common operation. Although ready-made class libraries and tools can be used to connect to the database, various abnormal situations may still occur during program development, among which SQLException is one of them. SQLException is an exception class provided by Java. It describes errors that occur when accessing the database, such as query statement errors, table non-existence, connection disconnection, etc. For Java programmers, especially those using JDBC (Java Data

Go language connects to the database by importing the database driver, establishing a database connection, executing SQL statements, using prepared statements and transaction processing. Detailed introduction: 1. Import the database driver and use the github.com/go-sql-driver/mysql package to connect to the MySQL database; 2. Establish a database connection and provide the database connection information, including the database address, user name, password, etc. Establish a database connection and so on through the sql.Open function.
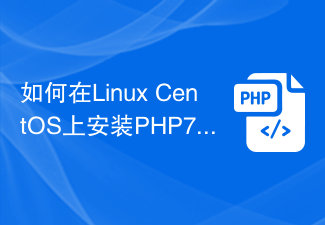
How to install PHP7 on LinuxCentOS Installing PHP7 on the LinuxCentOS operating system is a common requirement. This article will introduce you in detail how to install PHP7 on LinuxCentOS and provide specific code examples. First, you need to log in to your Linux CentOS server and do so as root or a user with sudo privileges. Next, follow the steps below to install it step by step: Step 1: Update the system at startup
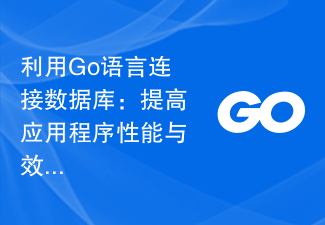
Using Go language to connect to the database: Improving application performance and efficiency As applications develop and the number of users increases, data storage and processing become more and more important. In order to improve the performance and efficiency of applications, properly connecting and operating the database is a crucial part. As a fast, reliable, and highly concurrency development language, Go language has the potential to provide efficient performance when processing databases. This article will introduce how to use Go language to connect to the database and provide some code examples. Install database driver using Go language
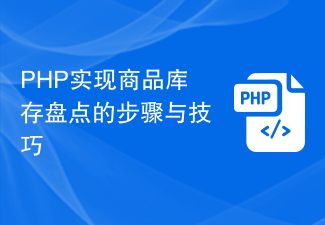
Steps and techniques for implementing product inventory in PHP In the e-commerce industry, product inventory management is a very important task. Timely and accurate inventory counting can avoid sales delays, customer complaints and other problems caused by inventory errors. This article will introduce the steps and techniques on how to use PHP to implement product inventory counting, and provide code examples. Step 1: Create a database First, we need to create a database to store product information. Create a database named "inventory" and then create a database named "prod
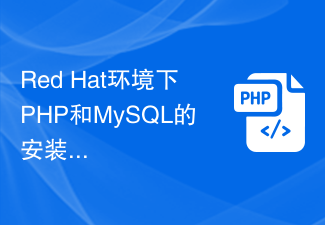
Installing PHP and MySQL in the RedHat environment is one of the basic steps to build a Web development environment. This article will detail the specific steps to install PHP and MySQL on RedHat systems and provide corresponding code examples. I hope that through the guidance of this article, you can successfully set up a PHP and MySQL environment and prepare for web development work. 1. Install PHP, log in to the RedHat system and open the terminal. Update the system package list using the following command: sudoyumup
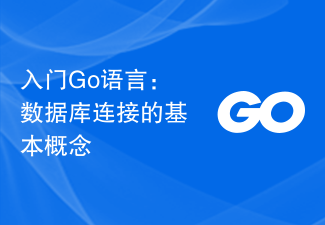
Learn Go language: basic knowledge of connecting to databases, specific code examples are required. Go language is an open source programming language. Its simple and efficient features make it loved and used by more and more developers. During the development process, it is often necessary to establish a connection with the database to perform operations such as reading, writing, updating, and deleting data. Therefore, learning how to connect to a database in Go language is a very important skill. Database driver In the Go language, a database driver is required to connect to the database. At present, the main database drivers of Go language are:
