PHP method definition and usage guide
PHP method definition and usage guide
PHP is a powerful server-side scripting language that is widely used in web development. In PHP, methods (also called functions) are a mechanism for encapsulating reusable blocks of code. This article will introduce you to the definition and use of PHP methods, with specific code examples for reference.
Definition of method
In PHP, the definition of method follows the following syntax format:
function 方法名(参数1, 参数2, ...) { // 方法体 }
The method definition starts with the keyword function
, followed by Method name, multiple parameters can be included in parentheses, separated by commas. Write the code for the method body within curly braces.
The following is a simple example that defines a method named addNumbers
, which implements the addition of two numbers and returns the result:
function addNumbers($num1, $num2) { $sum = $num1 + $num2; return $sum; }
Call of method
After defining the method, we can call the method through the method name and pass in the corresponding parameters. Method calls can be made wherever needed.
$result = addNumbers(5, 3); echo "5 + 3 = " . $result; // 输出:5 + 3 = 8
Parameter passing
The method can accept zero or more parameters, and the parameter passing can be by value or by reference.
Value passing: Copy the value of the parameter and pass it to the method. Modification of the parameter within the method will not affect the original value.
function increment($num) { $num++; return $num; } $value = 5; $newValue = increment($value); echo $value; // 输出:5 echo $newValue; // 输出:6
Pass by reference: Pass the memory address of the parameter to the method. Modification of the parameter within the method will affect the original value.
function incrementByReference(&$num) { $num++; } $value = 5; incrementByReference($value); echo $value; // 输出:6
Return value
The method can return a value through the return
keyword. If there is no return
statement in the method, the method will return null
.
function getMessage() { return "Hello, PHP!"; } $message = getMessage(); echo $message; // 输出:Hello, PHP!
Built-in functions
PHP has many useful built-in functions that can be called and used directly without defining them yourself. For example, strlen()
is used to get the length of a string, strtoupper()
is used to convert a string to uppercase, etc.
$string = "Hello, World!"; $length = strlen($string); $uppercase = strtoupper($string); echo $length; // 输出:13 echo $uppercase; // 输出:HELLO, WORLD!
Conclusion
Through the introduction of this article, you should have a preliminary understanding of the definition and use of PHP methods. Methods are an important concept in PHP programming, which can help us better organize code and improve code reusability and maintainability. I hope this article will help you learn PHP.
The above is the PHP method definition and usage guide in this article, hope
The above is the detailed content of PHP method definition and usage guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


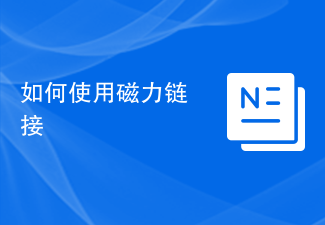
Magnet link is a link method for downloading resources, which is more convenient and efficient than traditional download methods. Magnet links allow you to download resources in a peer-to-peer manner without relying on an intermediary server. This article will introduce how to use magnet links and what to pay attention to. 1. What is a magnet link? A magnet link is a download method based on the P2P (Peer-to-Peer) protocol. Through magnet links, users can directly connect to the publisher of the resource to complete resource sharing and downloading. Compared with traditional downloading methods, magnetic
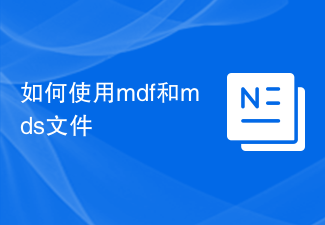
How to use mdf files and mds files With the continuous advancement of computer technology, we can store and share data in a variety of ways. In the field of digital media, we often encounter some special file formats. In this article, we will discuss a common file format - mdf and mds files, and introduce how to use them. First, we need to understand the meaning of mdf files and mds files. mdf is the extension of the CD/DVD image file, and the mds file is the metadata file of the mdf file.
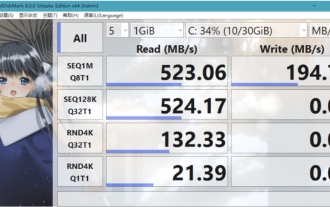
CrystalDiskMark is a small HDD benchmark tool for hard drives that quickly measures sequential and random read/write speeds. Next, let the editor introduce CrystalDiskMark to you and how to use crystaldiskmark~ 1. Introduction to CrystalDiskMark CrystalDiskMark is a widely used disk performance testing tool used to evaluate the read and write speed and performance of mechanical hard drives and solid-state drives (SSD). Random I/O performance. It is a free Windows application and provides a user-friendly interface and various test modes to evaluate different aspects of hard drive performance and is widely used in hardware reviews
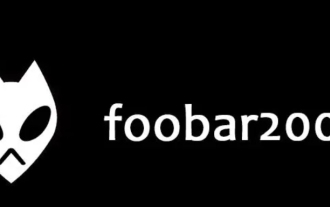
foobar2000 is a software that can listen to music resources at any time. It brings you all kinds of music with lossless sound quality. The enhanced version of the music player allows you to get a more comprehensive and comfortable music experience. Its design concept is to play the advanced audio on the computer The device is transplanted to mobile phones to provide a more convenient and efficient music playback experience. The interface design is simple, clear and easy to use. It adopts a minimalist design style without too many decorations and cumbersome operations to get started quickly. It also supports a variety of skins and Theme, personalize settings according to your own preferences, and create an exclusive music player that supports the playback of multiple audio formats. It also supports the audio gain function to adjust the volume according to your own hearing conditions to avoid hearing damage caused by excessive volume. Next, let me help you
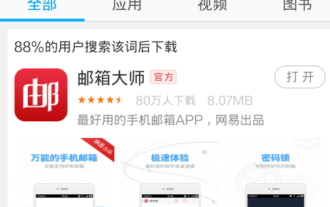
NetEase Mailbox, as an email address widely used by Chinese netizens, has always won the trust of users with its stable and efficient services. NetEase Mailbox Master is an email software specially created for mobile phone users. It greatly simplifies the process of sending and receiving emails and makes our email processing more convenient. So how to use NetEase Mailbox Master, and what specific functions it has. Below, the editor of this site will give you a detailed introduction, hoping to help you! First, you can search and download the NetEase Mailbox Master app in the mobile app store. Search for "NetEase Mailbox Master" in App Store or Baidu Mobile Assistant, and then follow the prompts to install it. After the download and installation is completed, we open the NetEase email account and log in. The login interface is as shown below
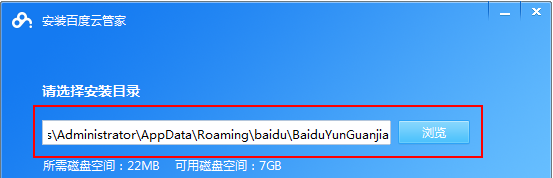
Cloud storage has become an indispensable part of our daily life and work nowadays. As one of the leading cloud storage services in China, Baidu Netdisk has won the favor of a large number of users with its powerful storage functions, efficient transmission speed and convenient operation experience. And whether you want to back up important files, share information, watch videos online, or listen to music, Baidu Cloud Disk can meet your needs. However, many users may not understand the specific use method of Baidu Netdisk app, so this tutorial will introduce in detail how to use Baidu Netdisk app. Users who are still confused can follow this article to learn more. ! How to use Baidu Cloud Network Disk: 1. Installation First, when downloading and installing Baidu Cloud software, please select the custom installation option.
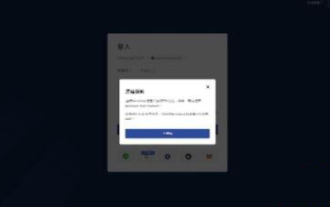
MetaMask (also called Little Fox Wallet in Chinese) is a free and well-received encryption wallet software. Currently, BTCC supports binding to the MetaMask wallet. After binding, you can use the MetaMask wallet to quickly log in, store value, buy coins, etc., and you can also get 20 USDT trial bonus for the first time binding. In the BTCCMetaMask wallet tutorial, we will introduce in detail how to register and use MetaMask, and how to bind and use the Little Fox wallet in BTCC. What is MetaMask wallet? With over 30 million users, MetaMask Little Fox Wallet is one of the most popular cryptocurrency wallets today. It is free to use and can be installed on the network as an extension
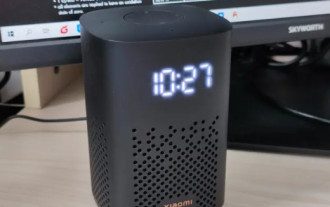
After long pressing the play button of the speaker, connect to wifi in the software and you can use it. Tutorial Applicable Model: Xiaomi 12 System: EMUI11.0 Version: Xiaoai Classmate 2.4.21 Analysis 1 First find the play button of the speaker, and press and hold to enter the network distribution mode. 2 Log in to your Xiaomi account in the Xiaoai Speaker software on your phone and click to add a new Xiaoai Speaker. 3. After entering the name and password of the wifi, you can call Xiao Ai to use it. Supplement: What functions does Xiaoai Speaker have? 1 Xiaoai Speaker has system functions, social functions, entertainment functions, knowledge functions, life functions, smart home, and training plans. Summary/Notes: The Xiao Ai App must be installed on your mobile phone in advance for easy connection and use.
