Explore the open source features and advantages of Golang
Golang (also known as Go language) is an open source programming language developed by Google. Since its release in 2009, it has gradually become a popular programming language. Golang has many open source features and advantages. This article will explore these features in depth, combined with specific code examples, to help readers better understand the advantages of Golang.
1. Concurrency support
Golang’s powerful concurrency support is one of its most significant advantages. Goroutine is a lightweight thread in Golang that can handle concurrent tasks efficiently. The following is a simple Goroutine example:
package main import ( "fmt" "time" ) func sayHello() { for i := 0; i < 3; i++ { fmt.Println("Hello") time.Sleep(time.Second) } } func main() { go sayHello() time.Sleep(2 * time.Second) fmt.Println("Main function") }
In the above example, the sayHello
function will run in a separate Goroutine while the main function continues to execute. Through Goroutine, Golang can easily implement concurrent operations and improve program performance and efficiency.
2. Memory management
Golang has the feature of automatic garbage collection, which can automatically manage memory when the program is running to avoid memory leaks. The following is a code snippet showing Golang garbage collection:
package main import "fmt" func main() { for i := 0; i < 1000000; i++ { s := make([]int, 1000) _ = s } var m runtime.MemStats runtime.ReadMemStats(&m) fmt.Printf("TotalAlloc: %d ", m.TotalAlloc) }
Through the runtime.MemStats
and runtime.ReadMemStats
functions, you can get the memory usage of the current program, help Developers have better control over memory management.
3. Rich standard library
Golang’s standard library provides a wealth of functions, covering network programming, data analysis, encryption and decryption, etc. The following is a simple HTTP server example:
package main import ( "net/http" "fmt" ) func handler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, Golang!") } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) }
The above code shows how to use Golang's standard library to build a simple HTTP server, register the processor function through http.HandleFunc
, and then call http.ListenAndServe
Start the server and implement a simple Web service.
4. Efficient compilation and execution speed
Golang’s compilation speed is very fast, and the entire program can be compiled within a few seconds, which greatly improves development efficiency. At the same time, Golang's execution speed is also very fast, its optimization and processing capabilities are strong, and it is suitable for handling high-concurrency scenarios.
In summary, Golang, as an open source programming language, has the advantages of strong concurrency support, automatic garbage collection, rich standard library and efficient compilation and execution speed. Through the above specific code examples, I hope readers can better understand and master the powerful features of Golang, and use its advantages in actual development to improve programming efficiency and program performance.
The above is the detailed content of Explore the open source features and advantages of Golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


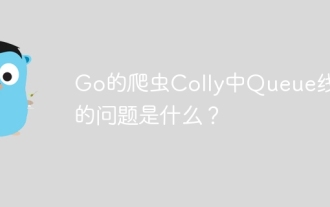
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
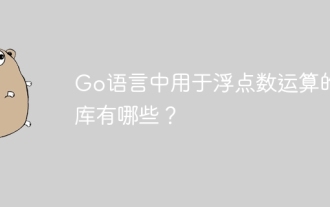
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
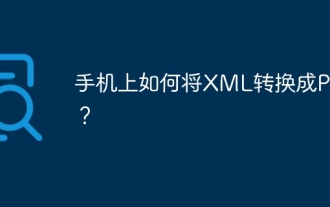
It is not easy to convert XML to PDF directly on your phone, but it can be achieved with the help of cloud services. It is recommended to use a lightweight mobile app to upload XML files and receive generated PDFs, and convert them with cloud APIs. Cloud APIs use serverless computing services, and choosing the right platform is crucial. Complexity, error handling, security, and optimization strategies need to be considered when handling XML parsing and PDF generation. The entire process requires the front-end app and the back-end API to work together, and it requires some understanding of a variety of technologies.
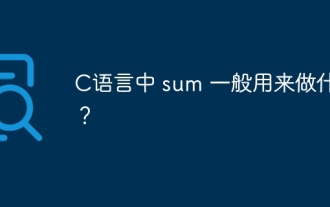
There is no function named "sum" in the C language standard library. "sum" is usually defined by programmers or provided in specific libraries, and its functionality depends on the specific implementation. Common scenarios are summing for arrays, and can also be used in other data structures, such as linked lists. In addition, "sum" is also used in fields such as image processing and statistical analysis. An excellent "sum" function should have good readability, robustness and efficiency.
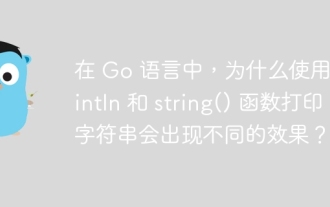
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
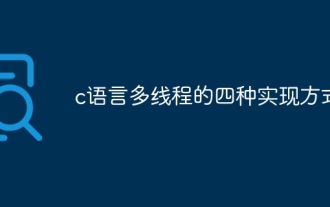
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
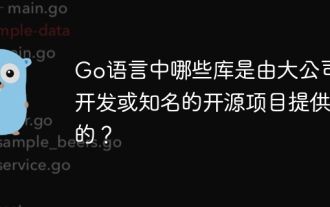
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
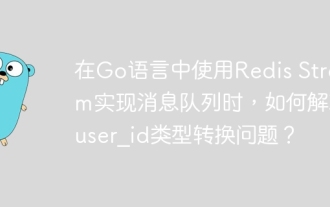
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
