Understand the characteristics and advantages of Golang coroutines
Golang is a very powerful programming language in the field of concurrent programming. The underlying layer supports lightweight coroutines (Goroutines) and has built-in concurrency primitives and tools. This article will focus on the characteristics and advantages of Golang coroutines, and illustrate them with specific code examples.
1. Characteristics of Golang coroutines
- Lightweight: Golang’s coroutines are lightweight, and the cost of creation and destruction is very low. A program can create thousands of coroutines without causing a large consumption of system resources.
- Concurrency: Golang's coroutines are executed concurrently, and concurrent processing can be achieved between multiple coroutines, thereby improving the performance of the program.
- Simple and easy to use: Golang provides a simple and easy-to-use coroutine scheduling mechanism. Developers only need to focus on the business logic without paying too much attention to the underlying details.
- Communication mechanism: Golang provides Channel as a communication pipeline between coroutines to facilitate data transfer and synchronization operations between coroutines.
2. Advantages of Golang coroutines
- Efficient use of multi-cores: Golang’s coroutines can run simultaneously on multi-core processors, effectively Utilize multi-core resources to improve program concurrency performance.
- Avoid problems caused by shared state: Golang's coroutines share data through communication, avoiding the complexity of synchronization and locking caused by using shared state in traditional concurrent programming.
- Handle blocking operations gracefully: Using coroutine-based concurrency mode can simplify the code for processing blocking IO operations, avoiding the use of callback functions or blocking threads in traditional multi-threaded programming.
3. Code example of Golang coroutine
The following is a simple example to demonstrate the use of Golang coroutine. Suppose we have a requirement: concurrently process a batch of tasks and The result is output to the channel.
package main import ( "fmt" "time" ) func worker(id int, jobs <-chan int, results chan<- int) { for job := range jobs { fmt.Printf("Worker %d processing job %d ", id, job) time.Sleep(time.Second) // 模拟任务处理耗时 results <- job * 2 } } func main() { jobs := make(chan int, 5) results := make(chan int, 5) // 启动3个协程进行任务处理 for i := 1; i <= 3; i++ { go worker(i, jobs, results) } // 向jobs通道发送任务 for j := 1; j <= 5; j++ { jobs <- j } close(jobs) // 获取处理结果 for k := 1; k <= 5; k++ { result := <-results fmt.Printf("Result: %d ", result) } }
In this example, we created two channels (jobs and results) and started 3 coroutines to process tasks concurrently. Each coroutine continuously obtains tasks from the jobs channel through a for loop, and sends the results to the results channel after processing.
Through this example, we can see the simple and efficient concurrent programming method of Golang coroutines, as well as the convenience of communication between coroutines through channels.
Conclusion
Golang's coroutine is a powerful concurrent programming tool, which is lightweight, efficient and concise. By rationally utilizing coroutines, high concurrency can be easily achieved. program. We hope that through the introduction and examples of this article, readers will have a deeper understanding of the characteristics and advantages of Golang coroutines, so that they can better utilize and apply coroutines in actual project development.
The above is the detailed content of Understand the characteristics and advantages of Golang coroutines. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
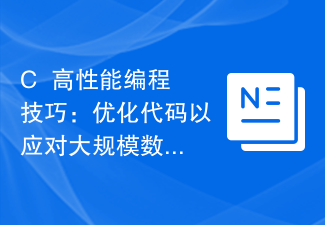
C++ is a high-performance programming language that provides developers with flexibility and scalability. Especially in large-scale data processing scenarios, the efficiency and fast computing speed of C++ are very important. This article will introduce some techniques for optimizing C++ code to cope with large-scale data processing needs. Using STL containers instead of traditional arrays In C++ programming, arrays are one of the commonly used data structures. However, in large-scale data processing, using STL containers, such as vector, deque, list, set, etc., can be more
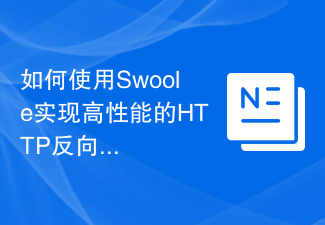
How to use Swoole to implement a high-performance HTTP reverse proxy server Swoole is a high-performance, asynchronous, and concurrent network communication framework based on the PHP language. It provides a series of network functions and can be used to implement HTTP servers, WebSocket servers, etc. In this article, we will introduce how to use Swoole to implement a high-performance HTTP reverse proxy server and provide specific code examples. Environment configuration First, we need to install the Swoole extension on the server
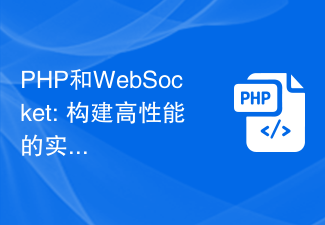
PHP and WebSocket: Building high-performance real-time applications As the Internet develops and user needs increase, real-time applications are becoming more and more common. The traditional HTTP protocol has some limitations when processing real-time data, such as the need for frequent polling or long polling to obtain the latest data. To solve this problem, WebSocket came into being. WebSocket is an advanced communication protocol that provides two-way communication capabilities, allowing real-time sending and receiving between the browser and the server.
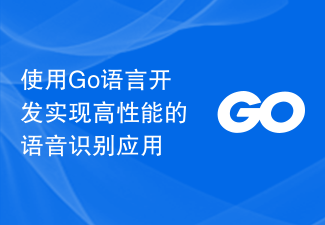
With the continuous development of science and technology, speech recognition technology has also made great progress and application. Speech recognition applications are widely used in voice assistants, smart speakers, virtual reality and other fields, providing people with a more convenient and intelligent way of interaction. How to implement high-performance speech recognition applications has become a question worth exploring. In recent years, Go language, as a high-performance programming language, has attracted much attention in the development of speech recognition applications. The Go language has the characteristics of high concurrency, concise writing, and fast execution speed. It is very suitable for building high-performance
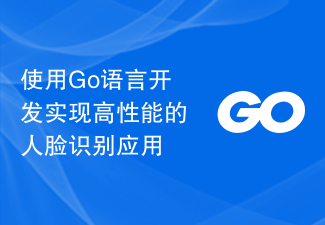
Use Go language to develop high-performance face recognition applications Abstract: Face recognition technology is a very popular application field in today's Internet era. This article introduces the steps and processes for developing high-performance face recognition applications using Go language. By using the concurrency, high performance, and ease-of-use features of the Go language, developers can more easily build high-performance face recognition applications. Introduction: In today's information society, face recognition technology is widely used in security monitoring, face payment, face unlocking and other fields. With the rapid development of the Internet
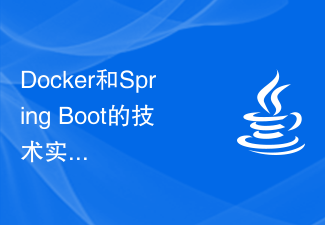
Technical practice of Docker and SpringBoot: quickly build high-performance application services Introduction: In today's information age, the development and deployment of Internet applications have become increasingly important. With the rapid development of cloud computing and virtualization technology, Docker, as a lightweight container technology, has received widespread attention and application. SpringBoot has also been widely recognized as a framework for rapid development and deployment of Java applications. This article will explore how to combine Docker and SpringB

High-performance data processing and implementation methods at the bottom of PHP require specific code examples. In modern web application development, data processing is a very common and important part. How to achieve high-performance data processing at the bottom of PHP is a skill and method that every PHP developer needs to pay attention to and master. This article introduces some methods for achieving high-performance data processing and provides specific code examples. Choosing the right data structure When dealing with large amounts of data, choosing the right data structure is very important. In PHP, arrays and objects are the most commonly used
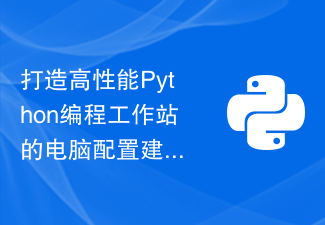
Title: Computer configuration recommendations for building a high-performance Python programming workstation. With the widespread application of the Python language in data analysis, artificial intelligence and other fields, more and more developers and researchers have an increasing demand for building high-performance Python programming workstations. When choosing a computer configuration, in addition to performance considerations, it should also be optimized according to the characteristics of Python programming to improve programming efficiency and running speed. This article will introduce how to build a high-performance Python programming workstation and provide specific
