Deep dive: Does Golang support macro definitions?
Does Golang support macro definition?
Golang is a statically typed, concurrency-supported, compiled programming language. Its concise syntax and efficient performance make it popular in the Internet industry. However, some developers may be wondering, does Golang support macro definition, a common feature in some other programming languages? This article will explore this issue in depth and analyze it with specific code examples.
Macro definitions are widely used in some programming languages. Macros can be used to achieve code reuse, simplify code, and improve code readability. In traditional programming languages such as C language, macro definition is a powerful tool that can replace code in the preprocessing stage to achieve some specific programming purposes. So, is there a similar function in a modern programming language like Golang?
First of all, it needs to be clear that Golang does not support macro definition in the traditional sense. In Golang, there are no operations such as macro expansion and macro replacement, and there is no preprocessor similar to the C language. The original design intention of Golang is to keep the language as simple and clear as possible and avoid excessive syntactic sugar and complexity so that programmers can more easily understand and maintain the code.
Although Golang does not have the feature of macro definition, in some actual development scenarios, some techniques can be used to simulate the function of macro definition. For example, you can use functions instead of macro definitions to achieve code reuse and simplification through function parameters and return values. The following uses a specific code example to demonstrate this implementation.
package main import "fmt" // 定义一个模拟的宏函数,实现将两个数字相加 func Add(a, b int) int { return a + b } func main() { num1 := 10 num2 := 20 // 调用模拟的宏函数实现加法运算 sum := Add(num1, num2) fmt.Printf("The sum of %d and %d is %d ", num1, num2, sum) }
In the above sample code, a function named Add is defined to simulate the function of a macro to add two numbers. In the main function, directly call the Add function to perform the addition operation and output the result. In this way, functions similar to macro definition can be achieved, which can simplify the code and improve readability.
In general, although Golang does not directly support macro definitions in the traditional sense, similar functions can be achieved through some techniques and methods. Programmers can choose appropriate methods to simplify code and improve efficiency based on actual needs and scenarios. When writing Golang code, you should follow the design principles of the language itself, give full play to its advantages, and write clear and efficient code.
To sum up, although Golang does not support macro definition, similar functions can be achieved through functions and other methods, and programmers can choose and apply them according to the actual situation. When learning and using Golang, you need to have a deep understanding of its syntax features and design concepts, and write robust and efficient code based on actual needs.
The above is the detailed content of Deep dive: Does Golang support macro definitions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


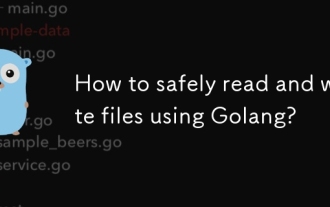
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
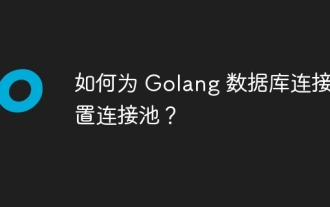
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
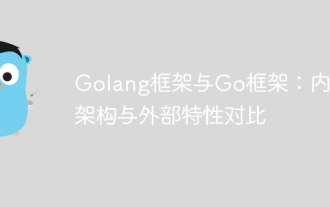
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
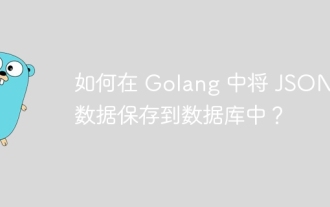
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
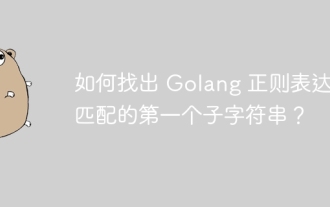
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
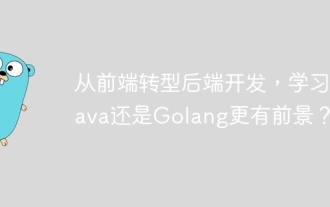
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
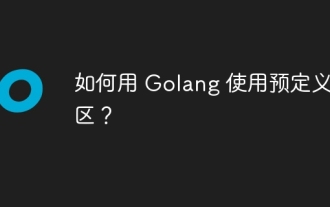
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
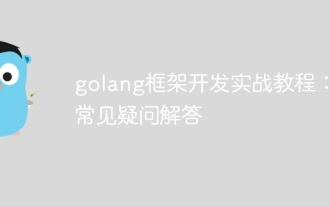
Go framework development FAQ: Framework selection: Depends on application requirements and developer preferences, such as Gin (API), Echo (extensible), Beego (ORM), Iris (performance). Installation and use: Use the gomod command to install, import the framework and use it. Database interaction: Use ORM libraries, such as gorm, to establish database connections and operations. Authentication and authorization: Use session management and authentication middleware such as gin-contrib/sessions. Practical case: Use the Gin framework to build a simple blog API that provides POST, GET and other functions.
