


PHP development practice: dealing with missing numbers in arrays
PHP Development Practice: Handling Missing Numbers in Arrays
In actual development, we often encounter situations where we need to handle missing numbers in arrays. Missing numbers may be due to data transfer errors, database query issues, or other reasons. In PHP development, we can use some simple methods to deal with missing numbers in the array to ensure the accuracy and completeness of the data. Here's how to handle missing numbers in an array in PHP, with specific code examples.
1. Use the range function to generate a complete number array
When dealing with the problem of missing numbers, you first need to extract the numbers in the original array and generate a complete number array, and then compare Difference of two arrays to find missing numbers. We can use the range function in PHP to quickly generate a complete array of numbers, and then use the array_diff function to find the missing numbers.
$originalArray = [1, 2, 4, 6, 9]; // 原始数组 $min = min($originalArray); // 获取最小值 $max = max($originalArray); // 获取最大值 $completeArray = range($min, $max); // 生成完整的数字数组 $missingNumbers = array_diff($completeArray, $originalArray); // 找出缺失的数字 print_r($missingNumbers); // 输出缺失的数字
2. Use a loop to traverse the array to find missing numbers
In addition to using PHP built-in functions, we can also use a loop to traverse the original array to find missing numbers. The specific method is to use a loop to determine whether the number exists in the original array one by one. If it does not exist, it is a missing number.
$originalArray = [3, 5, 7, 9, 11]; // 原始数组 $min = min($originalArray); // 获取最小值 $max = max($originalArray); // 获取最大值 $missingNumbers = []; for ($i = $min; $i <= $max; $i++) { if (!in_array($i, $originalArray)) { $missingNumbers[] = $i; // 找出缺失的数字 } } print_r($missingNumbers); // 输出缺失的数字
3. Use the array_diff_key function to find missing numbers
In addition to the array_diff function, we can also use the array_diff_key function to find missing numbers. This function can compare the key names of arrays instead of key values, so it is suitable for associative arrays or situations where key names are required as the basis for judgment.
$originalArray = [1 => 'Apple', 2 => 'Banana', 4 => 'Orange', 5 => 'Grape']; // 原始关联数组 $completeArray = array_combine(range(min(array_keys($originalArray)), max(array_keys($originalArray)), range(0, 0)); // 生成完整的键名数组 $missingKeys = array_diff_key($completeArray, $originalArray); // 找出缺失的键名 $missingNumbers = array_values($missingKeys); // 转换为缺失的数字 print_r($missingNumbers); // 输出缺失的数字
Through the above methods, we can easily handle the problem of missing numbers in the array in PHP development. In actual projects, choose appropriate methods to handle missing numbers based on specific needs to ensure data integrity and accuracy. I hope the above content is helpful to you, and I wish you smooth application in PHP development!
The above is the detailed content of PHP development practice: dealing with missing numbers in arrays. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
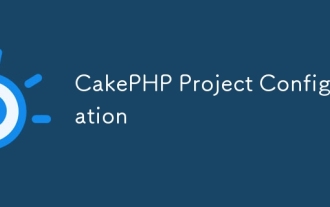
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
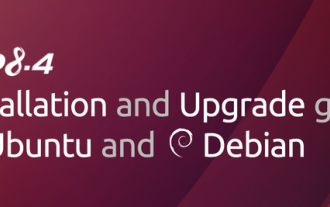
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
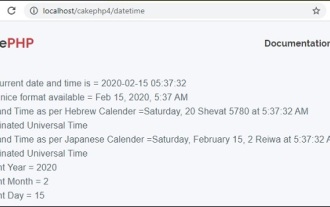
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
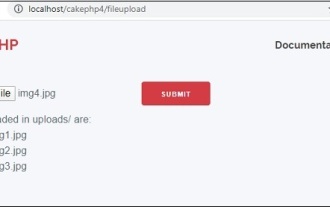
To work on file upload we are going to use the form helper. Here, is an example for file upload.
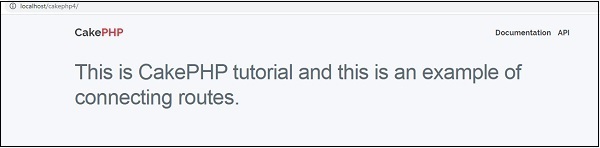
In this chapter, we are going to learn the following topics related to routing ?
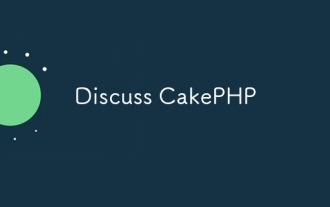
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
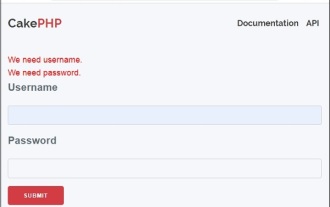
Validator can be created by adding the following two lines in the controller.
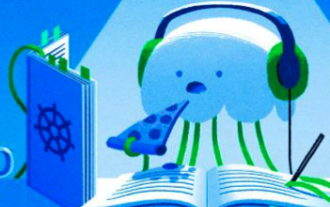
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
