Solution to NotImplementedError()
Mar 01, 2024 pm 03:10 PMCause of error
In python, the reason why NotImplementedError() is thrown in Tornado may be because an abstract method is not implemented or interface. These methods or interfaces are declared in the parent class but not implemented in the child class. Subclasses need to implement these methods or interfaces to work properly.
How to solve
The way to solve this problem is to implement the abstract method or interface declared by the parent class in the subclass.
If you are using a class to inherit from another class and you see this error, you should implement all abstract methods declared in the parent class in the child class.
If you are using an interface and you see this error, you should implement all methods declared in the interface in the class that implements the interface.
If you are not sure which methods need to be implemented, you can check the documentation or source code of the parent class or interface.
It should be noted that if multiple abstract methods are declared in the parent class or interface, all these methods need to be implemented in the subclass to solve this error.
Usage example
Yes, the following is a simple example that shows how to implement the abstract method declared by the parent class in the subclass.
from abc import ABC, abstractmethod class Parent(ABC): @abstractmethod def my_abstract_method(self): pass class Child(Parent): def my_abstract_method(self): print("I am implementing my_abstract_method.") # This will work c = Child() c.my_abstract_method()
In the above code, the parent class Parent declares an abstract method named my_abstract_method. In the subclass Child, we implement this method. So when we create a Child object and call my_abstract_method, it will print "I am implementing my_abstract_method."
Similarly, here is a simple example that shows how to implement a method declared in an interface in a class
from typing import List class MyInterface: def my_method(self,n:int) -> List[int]: pass class MyImplementation(MyInterface): def my_method(self,n:int) -> List[int]: return [i for i in range(n)] # this will work i = MyImplementation() print(i.my_method(5))
In the above code, a method named my_method is declared in the MyInterface class, and this method is implemented in the MyImplementation class. So when we create a MyImplementation object and call my_method, it will return a list of length n.
In short, these two examples show how to implement the methods declared in the parent class and interface in subclasses and implementation classes, so that NotImplementedError errors can be avoided
In practiceDevelopment, the NotImplementedError error may not only be caused by not implementing abstract methods or interfaces. It can be caused by bad design or implementation of the code.
For example, in some cases, you may override a method in a class, and the implementation of that method may throw NotImplementedError in some cases. This situation may be caused by certain conditions not being handled correctly.
Another common mistake is that when using a third-party library, some methods or classes in the library may throw NotImplementedError for some reasons. In this case, you should usually check documentation or source code to learn the specific cause of this error.
In summary, NotImplementedError is a common error, but it can have many different causes. Therefore, when solving this error, you need to combine the specific implementation of the code and the context of the error to find the cause.
The above is the detailed content of Solution to NotImplementedError(). For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
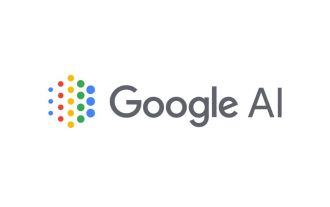
Google AI announces Gemini 1.5 Pro and Gemma 2 for developers
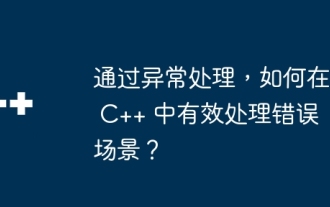
How to effectively handle error scenarios in C++ through exception handling?
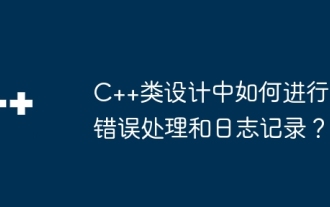
How to perform error handling and logging in C++ class design?
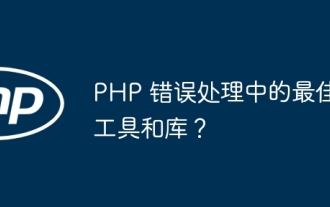
Best tools and libraries for PHP error handling?
