What network protocols does Go language support?
Go language, as a powerful programming language, provides rich network programming support and can easily handle various network protocols. This article will introduce some common network protocols supported by the Go language and provide some specific code examples. Let’s find out.
1. TCP protocol
TCP (Transmission Control Protocol) is a reliable, connection-oriented transport layer protocol. In the Go language, use the net
package to create a TCP connection and perform data transmission.
package main import ( "fmt" "net" ) func main() { conn, err := net.Dial("tcp", "example.com:80") if err != nil { fmt.Println("Error connecting:", err) return } defer conn.Close() conn.Write([]byte("GET / HTTP/1.1 Host: example.com ")) buffer := make([]byte, 1024) n, err := conn.Read(buffer) if err != nil { fmt.Println("Error reading:", err) return } fmt.Println("Received:", string(buffer[:n])) }
2. UDP Protocol
UDP (User Datagram Protocol) is a connectionless transport layer protocol, suitable for scenarios that require fast data transmission but do not require data reliability. In the Go language, the UDP protocol can be used through the net
package.
package main import ( "fmt" "net" ) func main() { conn, err := net.Dial("udp", "example.com:12345") if err != nil { fmt.Println("Error connecting:", err) return } defer conn.Close() conn.Write([]byte("Hello, UDP Server!")) buffer := make([]byte, 1024) n, err := conn.Read(buffer) if err != nil { fmt.Println("Error reading:", err) return } fmt.Println("Received:", string(buffer[:n])) }
3. HTTP protocol
HTTP (Hypertext Transfer Protocol) is an application layer protocol commonly used to transmit data between web browsers and web servers. The Go language provides the net/http
package for quickly creating HTTP clients and servers.
package main import ( "fmt" "io/ioutil" "net/http" ) func main() { resp, err := http.Get("https://www.example.com") if err != nil { fmt.Println("Error fetching URL:", err) return } defer resp.Body.Close() body, err := ioutil.ReadAll(resp.Body) if err != nil { fmt.Println("Error reading response:", err) return } fmt.Println("Response:", string(body)) }
4. WebSocket Protocol
WebSocket is a protocol for full-duplex communication over a single TCP connection, typically used in real-time applications. In the Go language, you can use the gorilla/websocket
package to implement WebSocket communication.
package main import ( "fmt" "github.com/gorilla/websocket" "net/url" ) func main() { u := url.URL{Scheme: "ws", Host: "example.com", Path: "/ws"} conn, _, err := websocket.DefaultDialer.Dial(u.String(), nil) if err != nil { fmt.Println("Error connecting to WebSocket:", err) return } defer conn.Close() err = conn.WriteMessage(websocket.TextMessage, []byte("Hello, WebSocket Server!")) if err != nil { fmt.Println("Error sending message:", err) return } _, message, err := conn.ReadMessage() if err != nil { fmt.Println("Error reading message:", err) return } fmt.Println("Received:", string(message)) }
Through the above code examples, we can see that Go language supports multiple network protocols, making developing network applications easier and more efficient. In actual development, appropriate network protocols can be selected and implemented according to needs to meet the needs of different scenarios. Hope this article will be helpful to you.
The above is the detailed content of What network protocols does Go language support?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


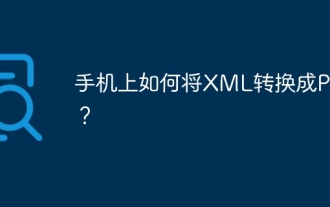
It is not easy to convert XML to PDF directly on your phone, but it can be achieved with the help of cloud services. It is recommended to use a lightweight mobile app to upload XML files and receive generated PDFs, and convert them with cloud APIs. Cloud APIs use serverless computing services, and choosing the right platform is crucial. Complexity, error handling, security, and optimization strategies need to be considered when handling XML parsing and PDF generation. The entire process requires the front-end app and the back-end API to work together, and it requires some understanding of a variety of technologies.
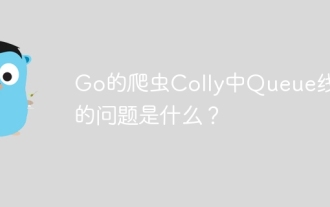
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
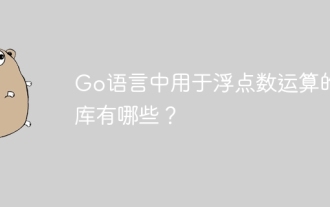
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
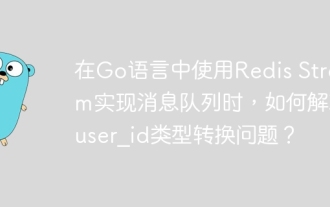
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
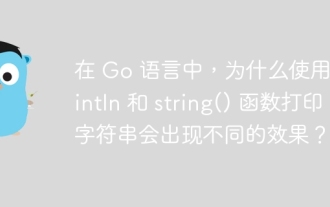
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
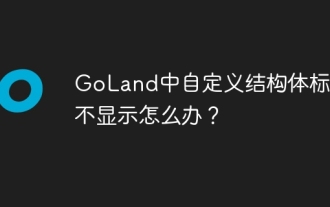
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
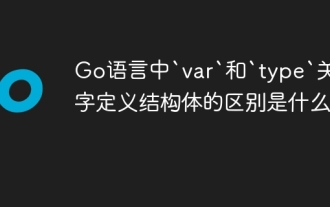
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
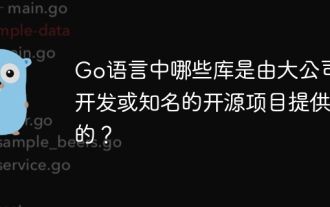
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
