The application of MySQL collation in database development
MySQL is a widely used relational database management system. Its flexibility and efficiency make it play an important role in database development. This article will introduce the application of MySQL in database development and provide some specific code examples.
1. Database connection
In database development, you first need to establish a connection with the MySQL database. The following is a simple Python sample code that demonstrates how to connect to a MySQL database:
import mysql.connector # 连接MySQL数据库 mydb = mysql.connector.connect( host="localhost", user="username", password="password", database="mydatabase" ) # 输出数据库连接信息 print(mydb)
In this code, we use the mysql.connector
module to establish a connection to the MySQL database , and specify the host name, user name, password, and database to connect to. After a successful connection, connection information will be output.
2. Create a table
In MySQL, data is stored in the form of tables. The following is a sample code that demonstrates how to create a table named students
in a MySQL database:
import mysql.connector mydb = mysql.connector.connect( host="localhost", user="username", password="password", database="mydatabase" ) mycursor = mydb.cursor() # 创建名为students的表格 mycursor.execute("CREATE TABLE students (id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(255), age INT)")
In this code, we use mycursor.execute() The
method executes the SQL statement and creates a students
table in the MySQL database, including the id, name and age fields.
3. Insert data
Inserting data into the created students
table is also a common operation in database development. The following is a simple sample code:
import mysql.connector mydb = mysql.connector.connect( host="localhost", user="username", password="password", database="mydatabase" ) mycursor = mydb.cursor() # 插入数据 sql = "INSERT INTO students (name, age) VALUES (%s, %s)" val = ("Alice", 20) mycursor.execute(sql, val) mydb.commit() print(mycursor.rowcount, "记录插入成功。")
In this code, we use the INSERT INTO
statement to insert a line containing the name Alice and age 20 into the students
table record, and submitted the modification through the mydb.commit()
method.
4. Querying data
In database development, querying data is a common task. The following is a sample code that demonstrates how to query data in the students
table:
import mysql.connector mydb = mysql.connector.connect( host="localhost", user="username", password="password", database="mydatabase" ) mycursor = mydb.cursor() # 查询数据 mycursor.execute("SELECT * FROM students") myresult = mycursor.fetchall() for row in myresult: print(row)
In this code, the SELECT * FROM
statement is used to query All data in the students
table, and obtain the query results through the mycursor.fetchall()
method and output them line by line.
5. Update and delete data
In database development, updating and deleting data are also common operations. The following is a sample code that demonstrates how to update data in the students
table:
import mysql.connector mydb = mysql.connector.connect( host="localhost", user="username", password="password", database="mydatabase" ) mycursor = mydb.cursor() # 更新数据 sql = "UPDATE students SET age = %s WHERE name = %s" val = (22, "Alice") mycursor.execute(sql, val) mydb.commit() print(mycursor.rowcount, "记录更新成功。")
In this code, use the UPDATE
statement to students
The age of the record named Alice in the table is updated to 22, and the modification is submitted.
6. Summary
MySQL is widely used in database development, and its flexibility and efficiency make it the first choice for developers. This article introduces some common operations of MySQL in database development and provides relevant code examples. I hope that readers can become more proficient in using MySQL database for development work through this article.
The above is the detailed content of The application of MySQL collation in database development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
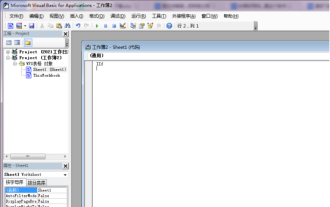
Most users use Excel to process table data. In fact, Excel also has a VBA program. Apart from experts, not many users have used this function. The iif function is often used when writing in VBA. It is actually the same as if The functions of the functions are similar. Let me introduce to you the usage of the iif function. There are iif functions in SQL statements and VBA code in Excel. The iif function is similar to the IF function in the excel worksheet. It performs true and false value judgment and returns different results based on the logically calculated true and false values. IF function usage is (condition, yes, no). IF statement and IIF function in VBA. The former IF statement is a control statement that can execute different statements according to conditions. The latter
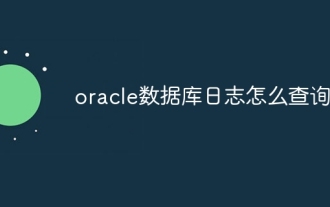
Oracle database log information can be queried by the following methods: Use SQL statements to query from the v$log view; use the LogMiner tool to analyze log files; use the ALTER SYSTEM command to view the status of the current log file; use the TRACE command to view information about specific events; use operations System tools look at the end of the log file.
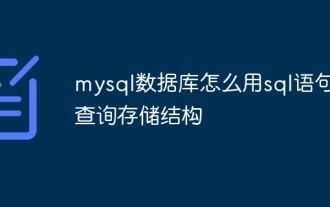
To query the MySQL database storage structure, you can use the following SQL statement: SHOW CREATE TABLE table_name; this statement will return the column definition and table option information of the table, including column name, data type, constraints and general properties of the table, such as storage engine and character set.
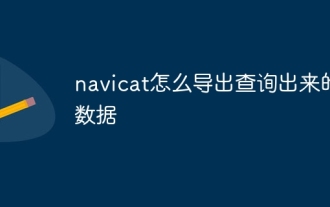
Export query results in Navicat: Execute query. Right-click the query results and select Export Data. Select the export format as needed: CSV: Field separator is comma. Excel: Includes table headers, using Excel format. SQL script: Contains SQL statements used to recreate query results. Select export options (such as encoding, line breaks). Select the export location and file name. Click "Export" to start the export.
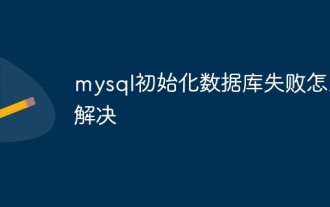
To resolve the MySQL database initialization failure issue, follow these steps: Check permissions and make sure you are using a user with appropriate permissions. If the database already exists, delete it or choose a different name. If the table already exists, delete it or choose a different name. Check the SQL statement for syntax errors. Confirm that the MySQL server is running and connectable. Verify that you are using the correct port number. Check the MySQL log file or Error Code Finder for details of other errors.
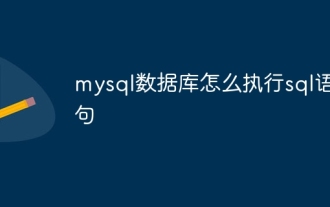
MySQL SQL statements can be executed by: Using the MySQL CLI (Command Line Interface): Log in to the database and enter the SQL statement. Using MySQL Workbench: Start the application, connect to the database, and execute statements. Use a programming language: import the MySQL connection library, create a database connection, and execute statements. Use other tools such as DB Browser for SQLite: download and install the application, open the database file, and execute the statements.
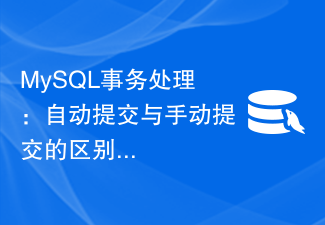
MySQL transaction processing: the difference between automatic submission and manual submission. In the MySQL database, a transaction is a set of SQL statements. Either all executions are successful or all executions fail, ensuring the consistency and integrity of the data. In MySQL, transactions can be divided into automatic submission and manual submission. The difference lies in the timing of transaction submission and the scope of control over the transaction. The following will introduce the difference between automatic submission and manual submission in detail, and give specific code examples to illustrate. 1. Automatically submit in MySQL, if it is not displayed
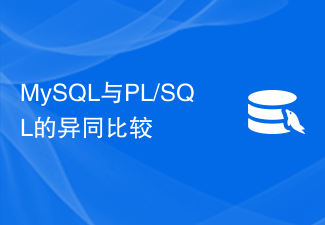
MySQL and PL/SQL are two different database management systems, representing the characteristics of relational databases and procedural languages respectively. This article will compare the similarities and differences between MySQL and PL/SQL, with specific code examples to illustrate. MySQL is a popular relational database management system that uses Structured Query Language (SQL) to manage and operate databases. PL/SQL is a procedural language unique to Oracle database and is used to write database objects such as stored procedures, triggers and functions. same
