Common problems and solutions for arrays in Golang
Common problems and solutions for arrays in Golang
In Golang programming, arrays are a common data structure, but unlike other languages, Golang The arrays in are of fixed length. In actual development, we often encounter some problems about arrays. This article will introduce some common problems and provide corresponding solutions and code examples.
Question 1: How to create an empty array?
In Golang, you can create an empty array using the following method:
var arr [5]int
In the above code, we define an integer array with a length of 5 and an initial value of 0. If you want to create an empty array, you can specify the length but not initialize any elements. This creates an empty array.
Question 2: How to traverse the elements of the array?
In Golang, you can use a for loop to traverse the elements of an array. For example:
arr := [3]int{1, 2, 3} for i := 0; i < len(arr); i++ { fmt.Println(arr[i]) }
The above code demonstrates how to traverse an integer array of length 3 and print the value of each element.
Question 3: How to add elements to an array?
Since arrays in Golang are of fixed length, new elements cannot be added to the array. If you need to add elements dynamically, consider using slices instead of arrays. Slicing is implemented based on arrays at the bottom and has dynamic length characteristics.
slice := []int{1, 2, 3} slice = append(slice, 4)
The above code demonstrates how to add new elements to the slice. Use the append function to add new elements at the end of the slice.
Question 4: How to copy an array?
In Golang, you can use the copy function to copy an array. The sample code is as follows:
arr1 := [3]int{1, 2, 3} arr2 := [3]int{} copy(arr2[:], arr1[:])
The above code demonstrates how to copy the elements of the arr1 array to the arr2 array. It should be noted that the first parameter of the copy function is the slice representation of the target array, and the second parameter is the slice representation of the source array.
Question 5: How to find the maximum and minimum values in an array?
You can easily find the maximum and minimum values in an array by traversing the array. The sample code is as follows:
arr := [5]int{4, 2, 7, 1, 9} max := arr[0] min := arr[0] for _, v := range arr { if v > max { max = v } if v < min { min = v } } fmt.Println("Max:", max) fmt.Println("Min:", min)
The above code demonstrates how to find the maximum and minimum values in an array. The maximum and minimum values can be obtained by traversing and comparing the values of each element.
Summary:
This article introduces common problems and solutions to arrays in Golang, and provides corresponding code examples. In actual development, it is very important to be proficient in the basic operations of arrays. I hope this article can be helpful to everyone.
The above is the detailed content of Common problems and solutions for arrays in Golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


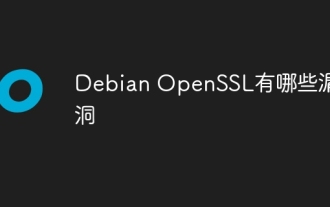
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
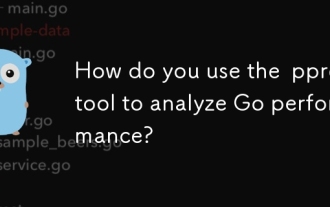
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
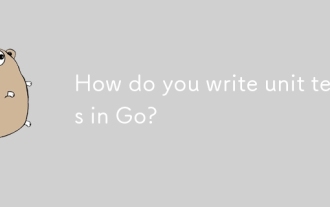
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
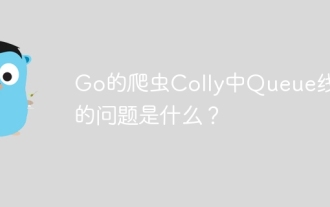
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
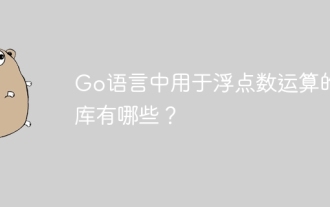
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
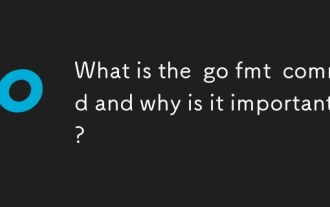
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
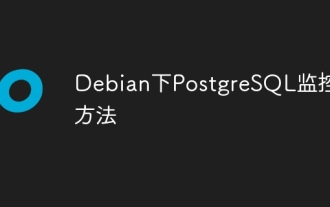
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
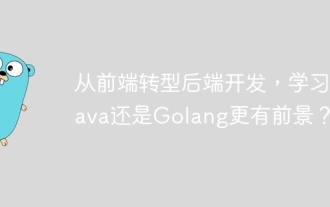
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
