What is the role of slicing in Golang? A comprehensive analysis of
What is the role of slicing in Golang? Comprehensive analysis
In Golang, slice is a very important and commonly used data structure. It can be regarded as an encapsulation of an array, realizing the function of a dynamic array. Through slicing, we can process data collections more flexibly and implement operations such as dynamic addition, deletion, modification, and query. This article will conduct a comprehensive analysis of the role of slicing in Golang and provide specific code examples to help readers deeply understand the use of slicing.
1. Definition and basic operations of slices
In Golang, you can define a slice in the following way:
var slice []int // 定义一个整型切片
The basic operations of slices include Create slices, obtain slice length and capacity, add elements to slices, traverse slices, etc. The following is sample code for some basic operations:
// 创建切片 slice := []int{1, 2, 3, 4, 5} // 获取切片长度和容量 fmt.Println("切片长度:", len(slice)) fmt.Println("切片容量:", cap(slice)) // 向切片中添加元素 slice = append(slice, 6) // 遍历切片 for i, v := range slice { fmt.Printf("索引:%d, 值:%d ", i, v) }
2. Dynamic expansion of slices
A slice corresponds to an array at the bottom layer. When the length of the slice exceeds its capacity , the underlying array will reallocate larger space to store elements, and copy the original elements to the new array. This automatic expansion mechanism makes slicing more flexible and eliminates the need for manual memory management.
slice := make([]int, 0, 5) fmt.Println("切片长度:", len(slice)) fmt.Println("切片容量:", cap(slice)) for i := 0; i < 10; i++ { slice = append(slice, i) fmt.Printf("切片长度:%d, 切片容量:%d ", len(slice), cap(slice)) }
3. Cutting and copying slices
By cutting a slice, you can get a new slice, which points to a part of the elements of the same underlying array. The slicing operation does not copy the contents of the underlying array, it just redefines the starting and ending indices of the slice.
slice1 := []int{1, 2, 3, 4, 5} slice2 := slice1[1:3] fmt.Println(slice2) // 输出:[2 3] // 切片的复制 slice3 := make([]int, 3) copy(slice3, slice1) fmt.Println(slice3) // 输出:[1 2 3]
4. Slice as function parameter
When a slice is passed as a parameter of a function, what is actually passed is a reference to the slice, that is, modifying the content of the slice within the function will Affects the original slice. This feature is very practical in actual development and can reduce memory usage and improve program execution efficiency.
func modifySlice(slice []int) { slice[0] = 100 } slice := []int{1, 2, 3} modifySlice(slice) fmt.Println(slice) // 输出:[100 2 3]
5. Notes on slicing
In the process of using slicing, you need to pay attention to the following points:
- Slicing is a reference Type, what is passed and copied is a reference, not a value
- The underlying array of the slice will reallocate memory as the slice expands
- The capacity of the slice is usually the length of the underlying array, but according to the actual The situation may change
Through the comprehensive analysis and specific code examples in this article, I believe readers will have a deeper understanding of slicing in Golang. As an important data structure, slicing is often used in actual development. Mastering the use of slicing can improve the efficiency and maintainability of the code. I hope this article can be helpful to readers. Everyone is welcome to practice and explore more and better apply slicing in Golang projects.
The above is the detailed content of What is the role of slicing in Golang? A comprehensive analysis of. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
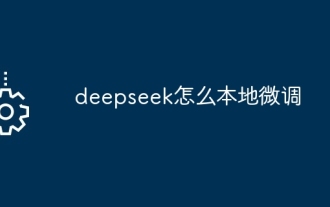
Local fine-tuning of DeepSeek class models faces the challenge of insufficient computing resources and expertise. To address these challenges, the following strategies can be adopted: Model quantization: convert model parameters into low-precision integers, reducing memory footprint. Use smaller models: Select a pretrained model with smaller parameters for easier local fine-tuning. Data selection and preprocessing: Select high-quality data and perform appropriate preprocessing to avoid poor data quality affecting model effectiveness. Batch training: For large data sets, load data in batches for training to avoid memory overflow. Acceleration with GPU: Use independent graphics cards to accelerate the training process and shorten the training time.
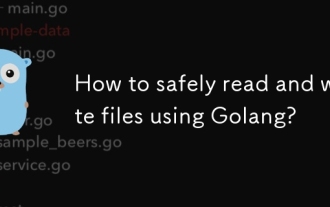
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
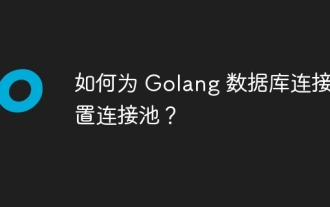
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
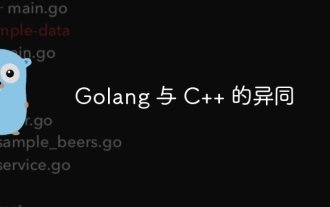
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
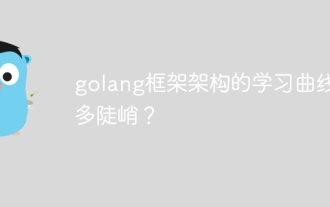
The learning curve of the Go framework architecture depends on familiarity with the Go language and back-end development and the complexity of the chosen framework: a good understanding of the basics of the Go language. It helps to have backend development experience. Frameworks that differ in complexity lead to differences in learning curves.
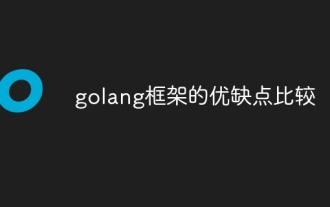
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.
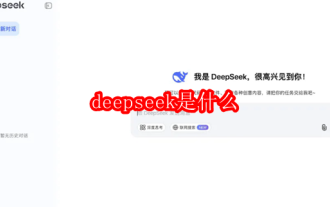
DeepSeek: An AI smart assistant you must not miss! DeepSeek is an AI tool that is popular among users. It has become a must-have software for many users recently. It provides powerful intelligent interactive communication functions, and the following is a detailed introduction to its powerful functions: DeepSeek's core functions: Text processing master: Easily create high-quality copywriting, translate and polish it, and improve your text expression ability. Programming tools: efficiently generate and complete code, quickly understand code logic, and effectively detect and correct code errors, greatly improving programming efficiency. Intelligent interaction expert: Built-in intelligent customer service and smart cockpit functions to provide a convenient interactive experience. Data analysis forecasting experts: supporters

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
