Build efficient Go language programming patterns
Building efficient Go language programming patterns requires specific code examples
In the field of software development, design patterns are general solutions to specific problems. As a modern and efficient programming language, Go language can also improve the maintainability, scalability and readability of programs through design patterns. This article will introduce some commonly used Go language programming patterns and combine them with specific code examples to illustrate their practical applications.
- Singleton Pattern
The singleton pattern is a creational design pattern that ensures that a class has only one instance and provides a global access point. In Go language, you can implement the singleton pattern by using sync.Once. The following is a sample code for singleton mode:
package singleton import "sync" type singleton struct { data string } var instance *singleton var once sync.Once func getInstance() *singleton { once.Do(func() { instance = &singleton{ data: "Hello, singleton!", } }) return instance }
In the above example, we use sync.Once to ensure that the GetInstance function will only be executed once, thus ensuring that only one instance is created. This approach can effectively avoid race conditions in multi-threaded environments.
- Factory Pattern
Factory Pattern is a creational design pattern that defines an interface for creating objects, but lets subclasses decide to instantiate Which category. In Go language, the factory pattern can be implemented through interfaces and specific factory classes. The following is a sample code of factory mode:
package factory type Shape interface { Draw() string } type Circle struct{} func (c *Circle) Draw() string { return "Drawing a circle" } type Rectangle struct{} func (r *Rectangle) Draw() string { return "Drawing a rectangle" } type ShapeFactory struct{} func (sf *ShapeFactory) CreateShape(shapeType string) Shape { switch shapeType { case "circle": return &Circle{} case "rectangle": return &Rectangle{} default: return nil } }
In factory mode, ShapeFactory is responsible for creating corresponding object instances based on the incoming parameters. This design can make the program easier to extend, and new shape classes only need to implement the Shape interface.
- Observer Pattern
The Observer Pattern is a behavioral design pattern that defines a one-to-many dependency relationship between objects. Once an object When the state changes, all objects that depend on it are notified and updated automatically. In Go language, you can use channels to implement the observer pattern. The following is a sample code of the observer pattern:
package observer type Observer interface { Update(data interface{}) } type Subject struct { observers []Observer } func (s *Subject) Attach(o Observer) { s.observers = append(s.observers, o) } func (s *Subject) Notify(data interface{}) { for _, o := range s.observers { o.Update(data) } } type ConcreteObserver struct { name string } func (co *ConcreteObserver) Update(data interface{}) { println(co.name, "received data:", data) }
In the above example, Subject is the observed and ConcreteObserver is the observer. Observers implement the Update method to receive notifications from the observed and respond accordingly.
Conclusion:
Through the above examples, we introduced the implementation of singleton pattern, factory pattern and observer pattern in Go language. Design patterns can help us better organize code structure, improve code quality and maintainability. Of course, design patterns are not silver bullets, and you need to choose the appropriate pattern to solve the problem based on the actual situation. I hope this article can help you build efficient Go language programming patterns.
The above is the detailed content of Build efficient Go language programming patterns. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
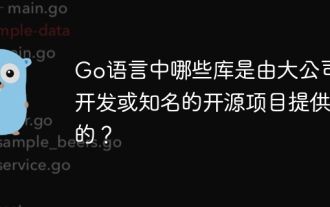
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
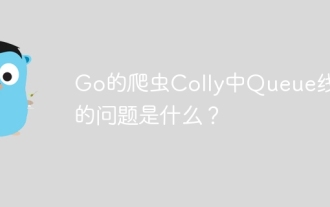
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
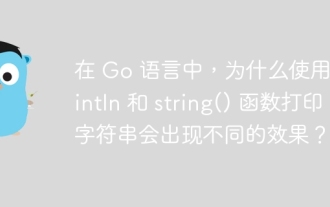
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
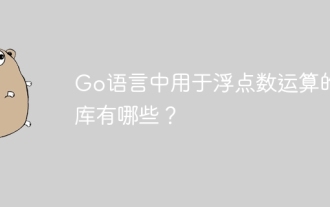
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
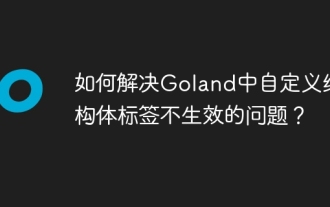
Regarding the problem of custom structure tags in Goland When using Goland for Go language development, you often encounter some configuration problems. One of them is...
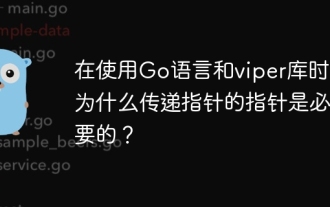
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
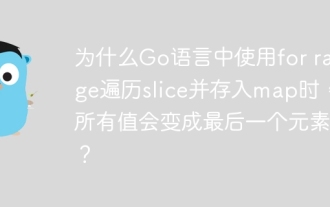
Why does map iteration in Go cause all values to become the last element? In Go language, when faced with some interview questions, you often encounter maps...
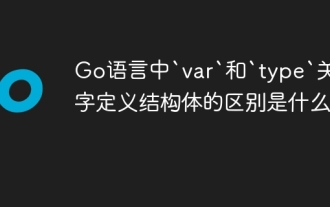
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
