


From beginner to proficient: Code implementation of commonly used data structures in Go language
Title: From Beginner to Mastery: Code Implementation of Commonly Used Data Structures in Go Language
Data structures play a vital role in programming, it is a program The basis of design. In the Go language, there are many commonly used data structures, and mastering the implementation of these data structures is crucial to becoming a good programmer. This article will introduce the commonly used data structures in the Go language and give corresponding code examples to help readers from getting started to becoming proficient in these data structures.
1. Array
An array is a basic data structure, which is a set of elements of the same type. In Go language, the length of arrays is fixed. The following is an example of the declaration and use of a simple integer array:
package main import "fmt" func main() { var arr [5]int arr[0] = 1 arr[1] = 2 arr[2] = 3 arr[3] = 4 arr[4] = 5 fmt.Println(arr) // 打印数组 // 遍历数组 for i := 0; i < len(arr); i++ { fmt.Printf("%d ", arr[i]) } }
2. Slice
Slice is an array-based data structure that can dynamically increase or decrease the length. The following is an example of the declaration and use of a simple integer slice:
package main import "fmt" func main() { var slice []int slice = append(slice, 1) slice = append(slice, 2, 3, 4, 5) fmt.Println(slice) // 打印切片 // 遍历切片 for _, value := range slice { fmt.Printf("%d ", value) } }
3. Queue (Queue)
The queue is a first-in, first-out (FIFO) data structure. The following is a simple implementation example of an integer queue:
package main import "fmt" type Queue struct { items []int } func (q *Queue) Enqueue(item int) { q.items = append(q.items, item) } func (q *Queue) Dequeue() int { if len(q.items) == 0 { return -1 } item := q.items[0] q.items = q.items[1:] return item } func main() { queue := Queue{} queue.Enqueue(1) queue.Enqueue(2) queue.Enqueue(3) fmt.Println(queue.Dequeue()) // 输出1 fmt.Println(queue.Dequeue()) // 输出2 }
4. Stack
The stack is a last-in-first-out (LIFO) data structure. The following is a simple implementation example of an integer stack:
package main import "fmt" type Stack struct { items []int } func (s *Stack) Push(item int) { s.items = append(s.items, item) } func (s *Stack) Pop() int { if len(s.items) == 0 { return -1 } item := s.items[len(s.items)-1] s.items = s.items[:len(s.items)-1] return item } func main() { stack := Stack{} stack.Push(1) stack.Push(2) stack.Push(3) fmt.Println(stack.Pop()) // 输出3 fmt.Println(stack.Pop()) // 输出2 }
By studying the above example code, readers can gradually master the implementation of commonly used data structures in the Go language, from entry to proficiency, and improve their programming skills. . Hope this article helps you!
The above is the detailed content of From beginner to proficient: Code implementation of commonly used data structures in Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


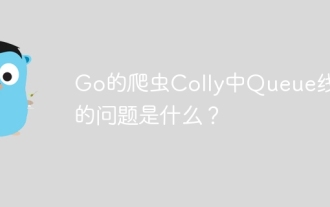
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
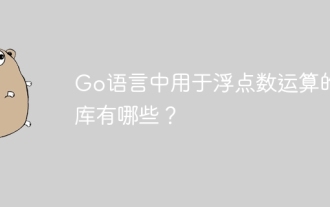
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
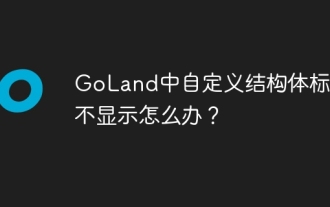
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
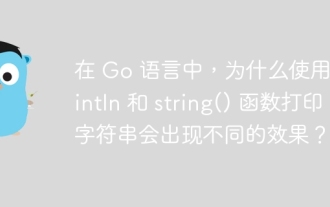
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
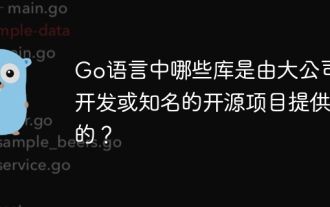
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
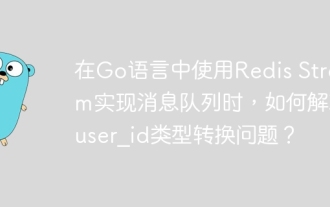
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
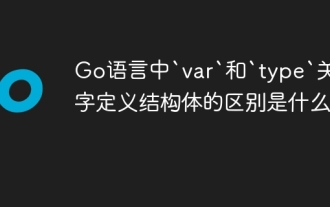
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
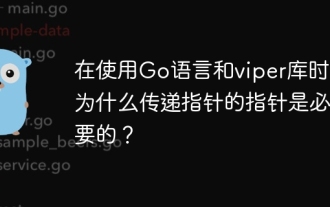
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
