Understand in which areas the GO language performs well?
GO language is an efficient, concise, and prominent programming language with outstanding concurrency features. It has excellent performance in many fields. This article will introduce the application of GO language in network programming, cloud computing, blockchain, artificial intelligence and other fields, and provide some specific code examples.
First, let us look at the performance of GO language in network programming. Because the GO language inherently supports concurrency, it is outstanding in network programming. GO language provides a rich standard library, including net, http and other packages, which can easily realize network programming needs. The following is a simple TCP server code example in GO language:
package main import ( "fmt" "net" ) func handleConnection(conn net.Conn) { defer conn.Close() buffer := make([]byte, 1024) _, err := conn.Read(buffer) if err != nil { fmt.Println("Error reading:", err) return } fmt.Println("Received data:", string(buffer)) } func main() { listener, err := net.Listen("tcp", "127.0.0.1:8080") if err != nil { fmt.Println("Error listening:", err) return } defer listener.Close() fmt.Println("Server started, listening on port 8080") for { conn, err := listener.Accept() if err != nil { fmt.Println("Error accepting connection:", err) continue } go handleConnection(conn) } }
The above code implements a simple TCP server, listens to the local 8080 port, and can process data sent by the client.
Secondly, GO language is also very good in the field of cloud computing. Due to the advantages of GO language in concurrent programming, it has great advantages in writing high-concurrency and distributed systems. In the field of cloud computing, GO language can quickly build high-performance cloud services. The following is a simple HTTP server code example written in GO language:
package main import ( "fmt" "net/http" ) func handler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, World!") } func main() { http.HandleFunc("/", handler) fmt.Println("Server started, listening on port 8080") http.ListenAndServe(":8080", nil) }
The above code implements a simple HTTP server, listens to the local 8080 port, and returns "Hello, World" when accessing the root path !".
In addition, GO language is also widely used in the blockchain field. Due to its efficient concurrency features and relatively concise syntax, GO language has become one of the preferred languages for many blockchain projects. Through the SDK or library of GO language, developers can quickly build blockchain applications. The following is a simple code example of a blockchain transaction implemented in GO language:
package main import ( "fmt" "crypto/sha256" ) type Transaction struct { Sender string Recipient string Amount float64 } func (t *Transaction) Hash() string { data := []byte(t.Sender + t.Recipient + fmt.Sprint(t.Amount)) hash := sha256.Sum256(data) return fmt.Sprintf("%x", hash) } func main() { transaction := Transaction{ Sender: "Alice", Recipient: "Bob", Amount: 10.0, } fmt.Println("Transaction Hash:", transaction.Hash()) }
The above code implements a simple blockchain transaction structure and calculates the hash value of the transaction.
Finally, GO language also performs well in the field of artificial intelligence. Due to the fast speed and good concurrency characteristics of the GO language, it has been widely used in large-scale data processing, machine learning, etc. The following is a code example of a simple linear regression machine learning algorithm implemented in GO language:
package main import ( "fmt" "github.com/sajari/regression" ) func main() { model := new(regression.Regression) model.SetObserved("x") model.SetVar(0, "y") data := [][]float64{ {1, 1}, {2, 2}, {3, 3}, {4, 4}, } for _, dataPoint := range data { x, y := dataPoint[0], dataPoint[1] model.Train(regression.DataPoint(y, []float64{x})) } model.Run() fmt.Printf("Regression formula: y = %.2f + %.2fx ", model.Coeff(0), model.Coeff(1)) }
The above code implements a simple linear regression machine learning algorithm, fitting a set of data and outputting regression formula.
In general, GO language has excellent performance in network programming, cloud computing, blockchain, artificial intelligence and other fields. Its efficiency, simplicity and concurrency features have won the favor of many developers. Through the code examples provided in this article, I believe readers can better understand the application of GO language in various fields.
The above is the detailed content of Understand in which areas the GO language performs well?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


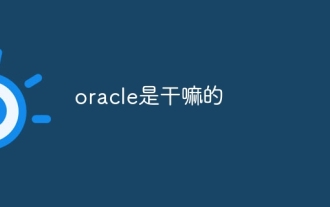
Oracle is the world's largest database management system (DBMS) software company. Its main products include the following functions: relational database management system (Oracle database) development tools (Oracle APEX, Oracle Visual Builder) middleware (Oracle WebLogic Server, Oracle SOA Suite) cloud service (Oracle Cloud Infrastructure) analysis and business intelligence (Oracle Analytics Cloud, Oracle Essbase) blockchain (Oracle Blockchain Pla
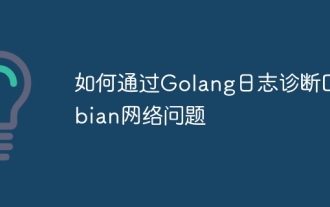
This article introduces how to use the Golang logging mechanism to efficiently diagnose network problems in Debian systems. We will explore several practical methods to help you quickly locate and resolve network connection failures. 1. Logging standard library log package: Golang's log package is ideal for recording network requests and response details. Adding logs before and after sending a request can clearly track the sending and receiving process of the request. Here is a simple example: packagemainimport("log""net/http""time")funcmain(){client:=&
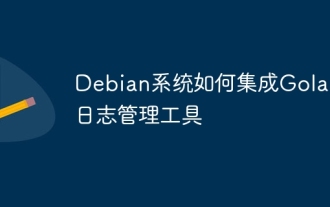
Integrate Go language log management tools on the Debian system. The steps are as follows: 1. Install the Go locale First, make sure that your Debian system has Go installed. If not installed, execute the following command: sudoaptupdatesudoaptinstallgolang-go Verification Installation: Goversion 2. Select logging tool Go language has a variety of logging tools, such as logrus, zap, zerolog, etc. This article takes logrus as an example. 3. Install logrus using goget command to install: gogetgithub.com/sirupsen/logrus IV. Configuration l
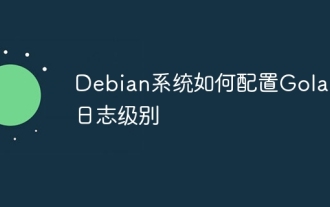
To configure the log level of the Golang application on the Debian system, you need to follow the following steps: Select the log library: First, select the appropriate log library. The Go standard library's log packages are simple to use, while third-party libraries such as logrus and zap provide more powerful features and performance. Set log level: Set the corresponding log level according to the selected log library. The settings of different libraries vary. The log package using the standard library logGo standard library does not directly support the log level, but can be simulated by custom output formats. The following example demonstrates how to control output based on a preset level: packagemainimport("log""os"
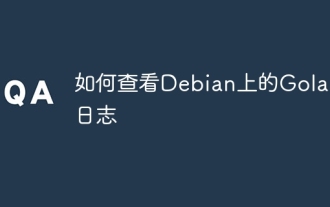
This article introduces several methods to view Go language application logs on Debian systems: Method 1: Use the journalctl command If your Go application runs in the form of a systemd service, you can use the journalctl command to view its logs. Assuming your service is called my-go-app, use the following command: the sudojournalctl-umy-go-appjournalctl command also supports multiple options, such as viewing the log of the last startup: sudojournalctl-b or viewing the log of a specific time period: sudojournalctl--since"2024-01-
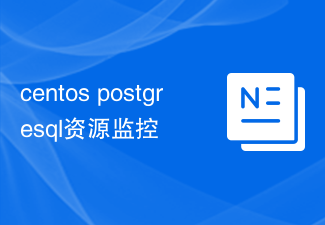
Detailed explanation of PostgreSQL database resource monitoring scheme under CentOS system This article introduces a variety of methods to monitor PostgreSQL database resources on CentOS system, helping you to discover and solve potential performance problems in a timely manner. 1. Use PostgreSQL built-in tools and views PostgreSQL comes with rich tools and views, which can be directly used for performance and status monitoring: pg_stat_activity: View the currently active connection and query information. pg_stat_statements: Collect SQL statement statistics and analyze query performance bottlenecks. pg_stat_database: provides database-level statistics, such as transaction count, cache hit
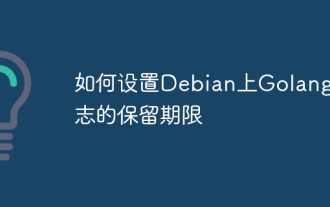
This article describes how to control the log file retention time of Golang applications on the Debian system. This usually requires the following steps: Identify the log library: First, determine which log library your Golang application uses, such as standard library log, logrus, or zap. Different libraries have different configuration methods. Log Rotation: Most log libraries need to be accompanied by log rotation tools to achieve automatic management of log files. logrotate is a commonly used tool. Use the standard library log to the standard library log itself does not provide log rotation function, and logrotate is required. Install logrotate:sudoapt
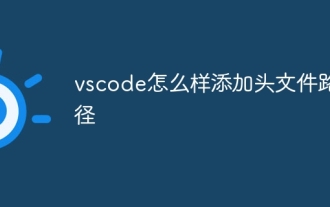
How to add header file paths in Visual Studio Code? Open the Settings page and search for the "c_cpp_properties.includePath" setting. Under "Include Path", add the path to the header file you want to include, separated by a semicolon. Use the #include keyword to specify the location of the header files in the standard library or project folder. Create a Makefile and add an INCLUDES row, specifying the include path. Compile the project and restart VSCode.
