


Advanced programming skills in Go language: implementing common algorithms and data structures
As an open source programming language, Go language has attracted much attention and has been widely used in recent years. Its simplicity, efficiency and concurrency characteristics make it perform well in various fields. This article will focus on advanced programming techniques in Go language and show specific code examples by implementing some common algorithms and data structures.
1. Arrays and slices
In the Go language, arrays and slices are commonly used data structures. An array is a collection of data with a fixed size, while a slice is a reference to an array, with a dynamic size. The following is a simple example showing how to create an array and a slice, and operate on the slice:
package main import ( "fmt" ) func main() { // 创建一个包含5个元素的整型数组 array := [5]int{1, 2, 3, 4, 5} // 创建一个切片,包含数组的前3个元素 slice := array[:3] // 在切片末尾添加一个元素 slice = append(slice, 6) // 打印切片的元素 for _, v := range slice { fmt.Println(v) } }
2. Linked list
The linked list is a common data structure used for storage A sequence of elements, each element containing a reference to the next element. The following is a simple one-way linked list implementation example:
package main import ( "fmt" ) type Node struct { data int next *Node } func main() { // 创建链表节点 node1 := Node{data: 1} node2 := Node{data: 2} node3 := Node{data: 3} // 构建链表关系 node1.next = &node2 node2.next = &node3 // 遍历链表并打印节点的值 current := &node1 for current != nil { fmt.Println(current.data) current = current.next } }
3. Stack and Queue
Stack and queue are two commonly used data structures. The stack is a last-in-first-out (LIFO) data structure, while the queue is a first-in-first-out (FIFO) data structure. The following is a simple stack and queue example:
package main import "fmt" func main() { // 栈的实现 stack := []int{} stack = append(stack, 1) // push v := stack[len(stack)-1] // top stack = stack[:len(stack)-1] // pop // 队列的实现 queue := []int{} queue = append(queue, 1) // enqueue v = queue[0] // front queue = queue[1:] // dequeue }
4. Sorting algorithm
The sorting algorithm is one of the very important algorithms. The following is an example of using the quick sort algorithm to sort slices:
package main import "fmt" func quickSort(arr []int) []int { if len(arr) < 2 { return arr } pivot := arr[0] var less, greater []int for _, v := range arr[1:] { if v <= pivot { less = append(less, v) } else { greater = append(greater, v) } } less = quickSort(less) greater = quickSort(greater) return append(append(less, pivot), greater...) } func main() { arr := []int{5, 2, 3, 1, 4} fmt.Println(quickSort(arr)) }
Through the above examples, we show how some common algorithms and data structures are implemented in the Go language, and give specific codes Example. hope
The above is the detailed content of Advanced programming skills in Go language: implementing common algorithms and data structures. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


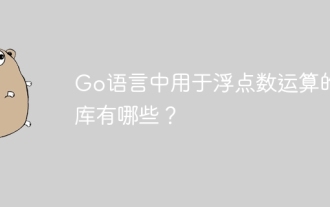
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
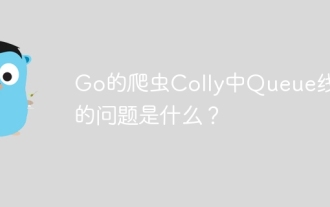
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
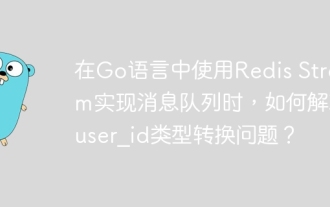
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
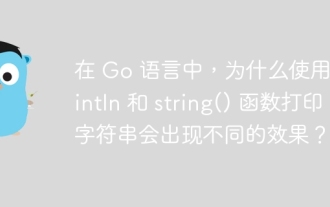
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
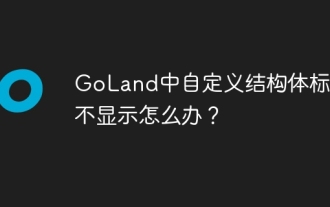
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
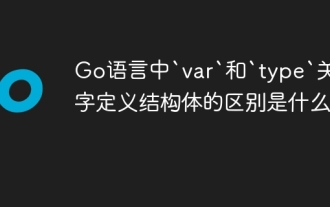
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
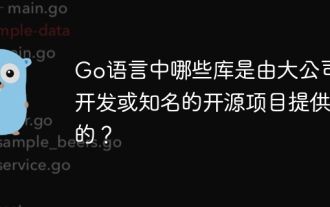
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
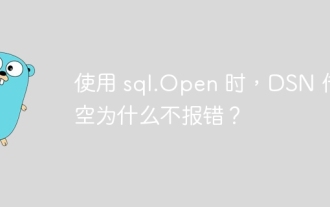
When using sql.Open, why doesn’t the DSN report an error? In Go language, sql.Open...
