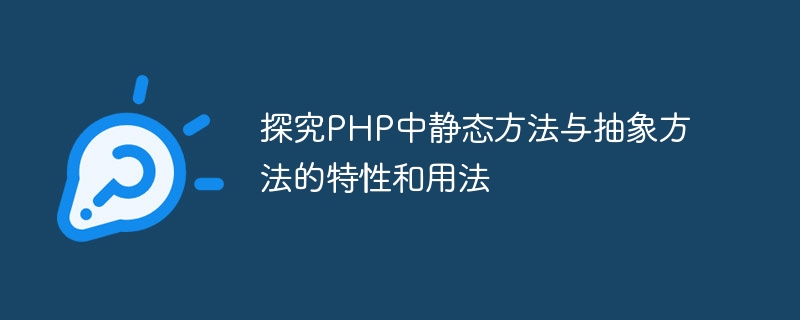
Characteristics and usage of static methods and abstract methods in PHP
In PHP programming, static methods and abstract methods are two different method types. They are oriented to Object programming plays an important role. This article will explore the characteristics and usage of static methods and abstract methods in PHP, and provide specific code examples.
1. Characteristics and Usage of Static Methods
- Characteristics of static methods
Static methods refer to methods defined in a class that can be called directly through the class name, rather than An instance of the class needs to be created. Its characteristics include:
- Can be called directly through the class name, no need to instantiate the object
- Static methods cannot access non-static properties or methods, only static properties or methods
- Static methods cannot use the $this keyword to reference the current object
- Usage of static methods
Static methods are usually used to implement some tool methods or global methods, such as logging, database operations, etc. Its usage includes:
- Static methods can be inherited by subclasses and can be rewritten in subclasses
- Static methods can be easily called in different places, improving code reusability and Maintainability
The following is a sample code that demonstrates how to define and call static methods in PHP:
class MathUtil {
public static function add($a, $b) {
return $a + $b;
}
}
// 调用静态方法
$result = MathUtil::add(3, 5);
echo $result; // 输出 8
Copy after login
2. Characteristics and usage of abstract methods
- Characteristics of abstract methods
Abstract methods refer to methods defined in abstract classes without specific implementation. Subclasses must implement these abstract methods. Its characteristics include: - Abstract methods have no method bodies, only method declarations
- Abstract methods can only exist in abstract classes
- An abstract class contains at least one abstract method, otherwise The abstract class must also be declared as an abstract class
- Usage of abstract methods
Abstract methods are usually used to define an interface with a set of methods, requiring subclasses to implement these methods to ensure the consistency of subclasses. Its usage includes: - The subclass must implement all abstract methods in the parent class, otherwise the subclass must also be declared as an abstract class
- Abstract methods can standardize the behavior of a group of classes and improve the code The readability and maintainability
The following is a sample code that demonstrates how to define and implement abstract methods in PHP:
abstract class Shape {
abstract public function getArea();
}
class Circle extends Shape {
private $radius;
public function __construct($radius) {
$this->radius = $radius;
}
public function getArea() {
return M_PI * $this->radius * $this->radius;
}
}
$circle = new Circle(5);
echo $circle->getArea(); // 输出 78.54
Copy after login
In summary, the Static methods and abstract methods are suitable for different scenarios and play an important role in programming. Through the rational use of static methods and abstract methods, the readability and maintainability of the code can be improved, while the reuse and expansion of the code can be achieved. I hope this article will help you understand the characteristics and usage of static methods and abstract methods in PHP.
The above is the detailed content of Explore the characteristics and usage of static methods and abstract methods in PHP. For more information, please follow other related articles on the PHP Chinese website!