Learn Golang from Scratch: A Quick Start Guide
Learn Golang from Scratch: Quick Start Guide
Golang is a programming language developed by Google, which has efficient concurrency processing capabilities and concise syntax structure , is widely used in cloud computing, network programming, big data processing and other fields. If you want to learn Golang and start writing your own programs, this article will provide you with a quick start guide to help you master the language from scratch.
Installing Golang
First, you need to install the Golang compiler on your computer. You can download the installation package suitable for your operating system through the Golang official website (https://golang.org/) and install it according to the official guide.
Writing a Hello World program
Below we will write a simple Hello World program step by step, so that you can quickly become familiar with Golang's syntax and basic structure.
package main import "fmt" func main() { fmt.Println("Hello World!") }
In this simple program, we first declare a package main
, which means that what we are writing is an executable program. Then, we introduced the fmt
package, which contains the input and output functions in the Golang standard library. Next, we defined a main
function as the entry point of the program, and called the fmt.Println
function to output "Hello World!".
Variables and data types
Next let’s learn about variables and data types in Golang. Golang is a statically typed language, which means you need to specify the type of the variable when declaring it.
package main import "fmt" func main() { var a int // 声明一个整型变量 a = 10 // 赋值 fmt.Println(a) var b, c string // 声明两个字符串变量 b = "Hello" c = "World" fmt.Println(b + " " + c) d := 3.14 // 使用短变量声明方式 fmt.Println(d) }
In this code, we first declare an integer variable a
, then assign it a value of 10 and output it. Next, we declared two string variables b
and c
, assigned the values to "Hello" and "World" respectively, and then used
to perform string concatenation and output. Finally, we used the short variable declaration method to declare a floating-point variable d
and output its value.
Process control
In addition to basic variables and data types, process control is also an essential part of programming. In Golang, you can use statements such as if
, for
, and switch
to control the execution flow of the program.
package main import "fmt" func main() { x := 5 if x > 0 { // if语句 fmt.Println("x is greater than 0") } for i := 0; i < 5; i++ { // for循环 fmt.Println(i) } switch x { // switch语句 case 1: fmt.Println("x is 1") case 5: fmt.Println("x is 5") default: fmt.Println("x is unknown") } }
In this code, we first use the if
statement to determine whether the variable x
is greater than 0, and then use the for
loop to output 0 to 4 . Finally, we use the switch
statement to match based on the value of x
and output the corresponding results.
Function
Function is the basic unit in the program, which can help us organize and reuse code. In Golang, function declaration and calling are very concise.
package main import "fmt" func add(x, y int) int { return x + y } func main() { result := add(3, 5) fmt.Println(result) }
In this code, we define a add
function that accepts two integer parameters and returns their sum. Then the add
function is called in the main
function and the result is output.
Conclusion
Through the above quick start guide, I hope you can have a preliminary understanding of Golang and be able to start writing simple programs. Of course, Golang still has many powerful features and functions waiting for you to explore. I hope you can continue to learn and continuously improve your programming abilities in practice. Happy programming!
The above is the detailed content of Learn Golang from Scratch: A Quick Start Guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



Kimi: In just one sentence, in just ten seconds, a PPT will be ready. PPT is so annoying! To hold a meeting, you need to have a PPT; to write a weekly report, you need to have a PPT; to make an investment, you need to show a PPT; even when you accuse someone of cheating, you have to send a PPT. College is more like studying a PPT major. You watch PPT in class and do PPT after class. Perhaps, when Dennis Austin invented PPT 37 years ago, he did not expect that one day PPT would become so widespread. Talking about our hard experience of making PPT brings tears to our eyes. "It took three months to make a PPT of more than 20 pages, and I revised it dozens of times. I felt like vomiting when I saw the PPT." "At my peak, I did five PPTs a day, and even my breathing was PPT." If you have an impromptu meeting, you should do it
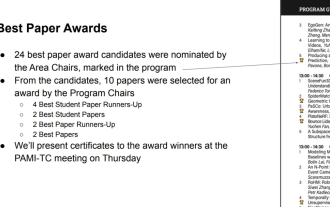
In the early morning of June 20th, Beijing time, CVPR2024, the top international computer vision conference held in Seattle, officially announced the best paper and other awards. This year, a total of 10 papers won awards, including 2 best papers and 2 best student papers. In addition, there were 2 best paper nominations and 4 best student paper nominations. The top conference in the field of computer vision (CV) is CVPR, which attracts a large number of research institutions and universities every year. According to statistics, a total of 11,532 papers were submitted this year, and 2,719 were accepted, with an acceptance rate of 23.6%. According to Georgia Institute of Technology’s statistical analysis of CVPR2024 data, from the perspective of research topics, the largest number of papers is image and video synthesis and generation (Imageandvideosyn
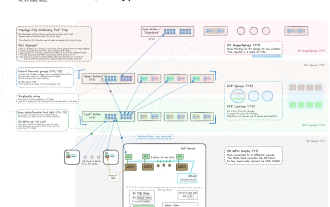
We know that LLM is trained on large-scale computer clusters using massive data. This site has introduced many methods and technologies used to assist and improve the LLM training process. Today, what we want to share is an article that goes deep into the underlying technology and introduces how to turn a bunch of "bare metals" without even an operating system into a computer cluster for training LLM. This article comes from Imbue, an AI startup that strives to achieve general intelligence by understanding how machines think. Of course, turning a bunch of "bare metal" without an operating system into a computer cluster for training LLM is not an easy process, full of exploration and trial and error, but Imbue finally successfully trained an LLM with 70 billion parameters. and in the process accumulate
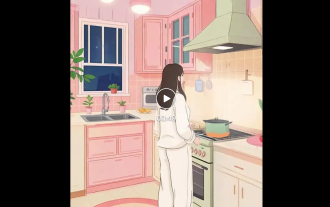
Editor of the Machine Power Report: Yang Wen The wave of artificial intelligence represented by large models and AIGC has been quietly changing the way we live and work, but most people still don’t know how to use it. Therefore, we have launched the "AI in Use" column to introduce in detail how to use AI through intuitive, interesting and concise artificial intelligence use cases and stimulate everyone's thinking. We also welcome readers to submit innovative, hands-on use cases. Video link: https://mp.weixin.qq.com/s/2hX_i7li3RqdE4u016yGhQ Recently, the life vlog of a girl living alone became popular on Xiaohongshu. An illustration-style animation, coupled with a few healing words, can be easily picked up in just a few days.
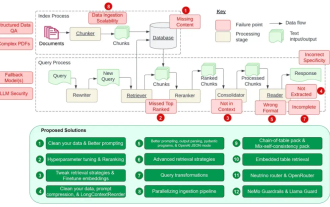
Retrieval-augmented generation (RAG) is a technique that uses retrieval to boost language models. Specifically, before a language model generates an answer, it retrieves relevant information from an extensive document database and then uses this information to guide the generation process. This technology can greatly improve the accuracy and relevance of content, effectively alleviate the problem of hallucinations, increase the speed of knowledge update, and enhance the traceability of content generation. RAG is undoubtedly one of the most exciting areas of artificial intelligence research. For more details about RAG, please refer to the column article on this site "What are the new developments in RAG, which specializes in making up for the shortcomings of large models?" This review explains it clearly." But RAG is not perfect, and users often encounter some "pain points" when using it. Recently, NVIDIA’s advanced generative AI solution
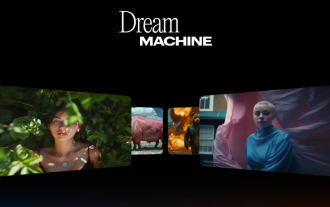
When Sora failed to come out, OpenAI's opponents used their weapons to destroy the streets. If Sora is not open for use, it will really be stolen! Today, San Francisco startup LumaAI played a trump card and launched a new generation of AI video generation model DreamMachine. Free and available to everyone. According to reports, the model can generate high-quality, realistic videos based on simple text descriptions, with effects comparable to Sora. As soon as the news came out, a large number of users crowded into the official website to try it out. Although officials claim that the model can generate 120-frame video in just two minutes, many users have been waiting for hours on the official website due to a surge in visits. BarkleyDai, Luma’s head of product growth, had to comment on Discord
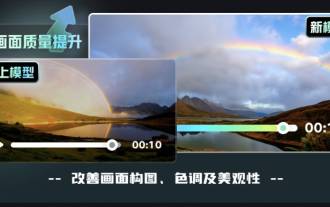
On July 24, Kuaishou video generation large model Keling AI announced that the basic model has been upgraded again and is fully open for internal testing. Kuaishou said that in order to allow more users to use Keling AI and better meet the different levels of usage needs of creators, from now on, on the basis of fully open internal testing, it will also officially launch a membership system for different categories of members. Provide corresponding exclusive functional services. At the same time, the basic model of Keling AI has also been upgraded again to further enhance the user experience. The basic model effect has been upgraded to further improve the user experience. Since its release more than a month ago, Keling AI has been upgraded and iterated many times. With the launch of this membership system, the basic model effect of Keling AI has once again undergone transformation. The first is that the picture quality has been significantly improved. The visual quality generated through the upgraded basic model
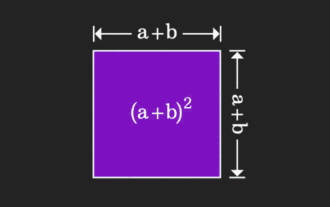
The matrix is difficult to understand, but it may be different if you look at it from another perspective. When learning mathematics, we are often frustrated by the difficulty and abstractness of the knowledge we learn; but sometimes, just by changing the perspective, we can find a simple and intuitive solution to the problem. For example, when we were learning the formula for the sum of squares (a+b)² when we were children, we may not understand why it is equal to a²+2ab+b². We only knew that it was written like this in the book and the teacher asked us to remember it like this; until one day we saw I saw this animated picture: It suddenly dawned on me that we can understand it from a geometric perspective! Now, this sense of enlightenment occurs again: a non-negative matrix can be equivalently converted into the corresponding directed graph! As shown in the figure below, the 3×3 matrix on the left can actually be
