Revealed: How Golang tackles big data challenges
In today’s digital era, big data has become one of the common challenges faced by various industries. With the rapid growth of data volume, traditional data processing technology has been unable to meet the needs of large-scale data processing. At the same time, due to the complexity and real-time requirements of big data itself, developers face more difficult tasks when processing big data.
In this context, Golang, as an efficient and concise programming language, is favored by developers. It uses a concurrency model and efficient garbage collection mechanism to make processing large-scale data more efficient and stable. So, how does Golang deal with big data challenges? Next, we will reveal the application of Golang in big data processing and provide some specific code examples.
1. Concurrent processing
When processing big data, it is usually necessary to process multiple data streams at the same time to improve processing efficiency and reduce time costs. Golang's concurrency model can help developers easily implement concurrent processing and improve program throughput. The following is a simple concurrent processing example:
package main import ( "fmt" "sync" ) func process(data int, wg *sync.WaitGroup) { defer wg.Done() // 模拟数据处理 result := data * 2 fmt.Println("Processed data:", result) } func main() { var wg sync.WaitGroup data := []int{1, 2, 3, 4, 5} for _, d := range data { wg.Add(1) go process(d, &wg) } wg.Wait() fmt.Println("All data processed") }
In the above example, we use the WaitGroup in the sync package to wait for the completion of all concurrent tasks. Through the concurrent execution of goroutines, we can process multiple data at the same time and improve processing efficiency.
2. Memory Management
Large-scale data processing often requires a large amount of memory space, and efficient memory management is particularly important in this case. Golang provides an efficient garbage collection mechanism that can automatically recycle unused memory and avoid memory leaks. The following is a simple memory management example:
package main import "fmt" func main() { var data []int for i := 0; i < 1000000; i++ { data = append(data, i) } // 使用完data后,及时释放内存 data = nil // 手动触发垃圾回收 _ = data }
In the above example, we release memory by setting data to nil, and garbage collection can be manually triggered through functions in the runtime package.
3. Parallel Computing
For large-scale data processing, complex calculation operations are usually required. Golang can achieve simple and efficient parallel computing through goroutine and channel. The following is a simple parallel computing example:
package main import ( "fmt" "time" ) func calculate(data int, result chan int) { time.Sleep(time.Second) // 模拟复杂计算 result <- data * 2 } func main() { data := []int{1, 2, 3, 4, 5} result := make(chan int, len(data)) for _, d := range data { go calculate(d, result) } for i := 0; i < len(data); i++ { fmt.Println("Processed data:", <-result) } close(result) }
In the above example, we transmit data through channels to achieve parallel computing, which can process large-scale data more efficiently.
To sum up, Golang, as an efficient and concise programming language, has the advantage of meeting the challenges of big data. Through concurrent processing, memory management, and parallel computing, developers can process large-scale data more efficiently. Of course, in actual applications, developers also need to choose appropriate technical solutions based on specific scenarios and needs. I believe that with the continuous development and application of Golang in the field of big data, it will bring more innovations and solutions to data processing.
The above is the detailed content of Revealed: How Golang tackles big data challenges. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


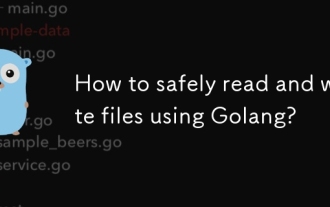
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
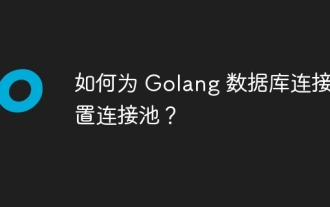
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
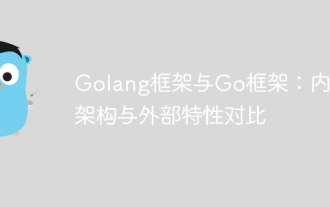
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
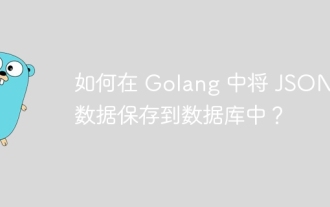
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
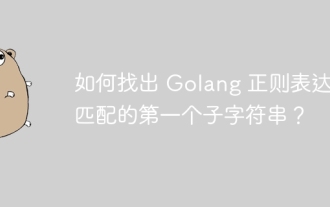
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
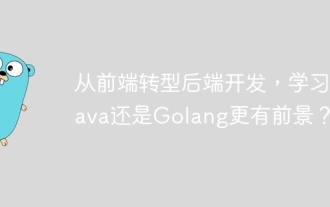
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
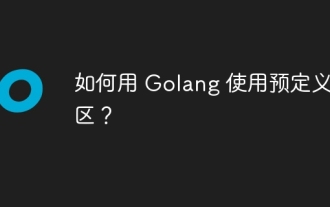
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
