How to quickly troubleshoot PHP open 500 errors
During the web development process, PHP developers often encounter 500 errors returned by the server. This is a common internal server error that may be caused by various reasons, including syntax Errors, server configuration issues, database connection issues, etc. When faced with this kind of error, it is crucial to troubleshoot and solve the problem in a timely manner. This article will introduce how to quickly troubleshoot PHP open 500 errors and provide specific code examples.
1. Check for PHP syntax errors
Syntax errors in PHP code are one of the common causes of 500 errors. When there are syntax errors in PHP code, the server cannot parse the code properly, resulting in an internal error. You can check PHP syntax errors through the following steps:
<?php // 检查PHP语法错误示例 echo "Hello World"; ?>
2. Check the PHP error log
The PHP error log is an important tool for troubleshooting 500 errors. You can find specific error information by checking the error log. and location. You can view the PHP error log through the following code example:
<?php // 查看PHP错误日志示例 ini_set('display_errors', 0); ini_set('log_errors', 1); ini_set('error_log', '/var/log/php_errors.log');
3. Check the server configuration
500 errors may be related to the server configuration, such as PHP version, PHP extension, etc. You can view the server configuration information through the following code example:
<?php // 查看服务器配置信息示例 phpinfo();
4. Check database connection problems
If the PHP code involves database operations, the 500 error may also be caused by database connection problems. You can check whether the database connection is normal through the following code example:
<?php // 检查数据库连接示例 $host = 'localhost'; $user = 'username'; $password = 'password'; $database = 'database'; $conn = new mysqli($host, $user, $password, $database); if ($conn->connect_error) { die("Database connection failed: " . $conn->connect_error); } else { echo "Database connection successful"; }
5. Use try-catch to catch exceptions
Use try-catch blocks in PHP code to catch exceptions and handle them appropriately, Avoid 500 errors. The following is an example:
<?php // 使用try-catch捕获异常示例 try { // 有可能抛出异常的代码 } catch (Exception $e) { echo 'Caught exception: ' . $e->getMessage(); }
Through the above aspects of troubleshooting, you can quickly locate and solve the problem of PHP opening 500 error. When solving problems, it is recommended to check with the above code examples and debug and fix them according to the specific situation. Hope this information is helpful in solving PHP500 error.
The above is the detailed content of How to quickly troubleshoot PHP open 500 errors. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


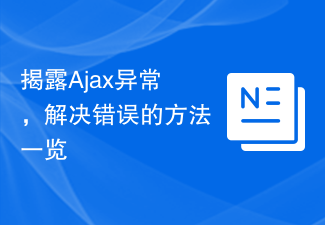
The secret of Ajax anomaly is revealed. How to deal with various errors requires specific code examples. In 2019, front-end development has become an important position that cannot be ignored in the Internet industry. As one of the most commonly used technologies in front-end development, Ajax can realize asynchronous page loading and data interaction, and its importance is self-evident. However, various errors and exceptions are often encountered when using Ajax technology. How to deal with these errors is a problem that every front-end developer must face. 1. Network errors When using Ajax to send requests, the most common error is
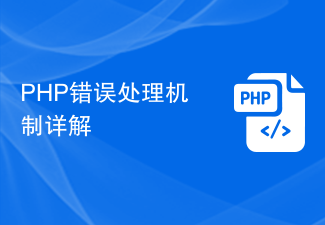
In PHP development, the error handling mechanism is crucial, because whether you are writing a web page or developing an application, you will inevitably encounter errors. In PHP, error handling mainly includes three aspects: error reporting, error logging and exception handling. This article will elaborate on the error handling mechanism in PHP. 1. Error reporting In PHP, error reporting is enabled by default. It can display error messages to users when running PHP programs. Error reporting levels are divided into the following four levels: E_ERROR:
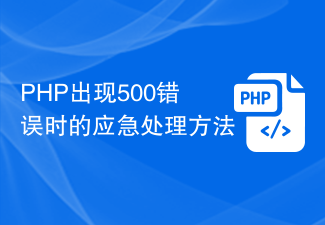
Emergency handling methods when 500 errors occur in PHP When using PHP to develop websites or applications, 500 errors are one of the common problems. When a 500 error occurs, it means that the server cannot handle the request correctly, and users will see an "InternalServerError" prompt when browsing the website. This error can be caused by many factors, such as PHP code errors, server configuration issues, incorrect permission settings, etc. This article will introduce in detail the emergency handling methods when a 500 error occurs in PHP.
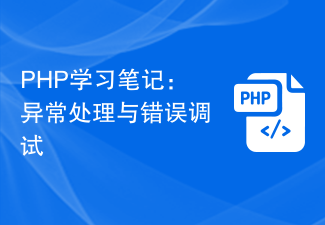
PHP study notes: Exception handling and error debugging In the process of writing PHP code, you will inevitably encounter various errors and exceptions. Good exception handling and error debugging can help us better locate problems and fix bugs, and improve the reliability and stability of the code. This article will introduce specific methods of exception handling and error debugging in PHP, and give relevant code examples. 1. The concept of exception handling. During program execution, if an unexpected situation occurs, such as database connection failure, file does not exist, etc., PHP will throw an exception.
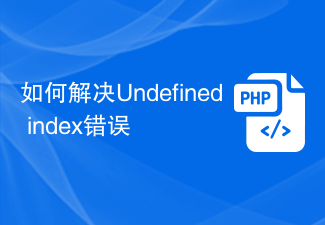
How to Fix UndefinedIndex Errors When writing and debugging PHP code, encountering UndefinedIndex errors is a very common situation. This error is usually caused by accessing an element that does not exist in the array. Solving this error is not only to avoid code errors, but also to ensure the robustness and reliability of the code. In the following article, some common solutions and tips will be introduced to help you deal with UndefinedIndex errors. First, let's look at a
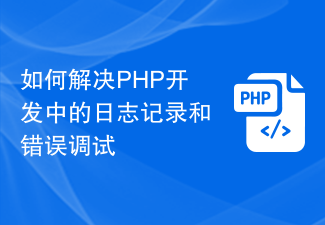
How to solve logging and error debugging in PHP development. In the PHP development process, logging and error debugging are very important links. Reasonable logging can help us better track problems during program execution and effectively troubleshoot errors; error debugging methods can help us quickly locate and solve problems. This article will provide you with some solutions and specific code examples from two aspects: logging and error debugging, to help you develop and debug PHP projects more efficiently. 1. Logging 1. Create log files
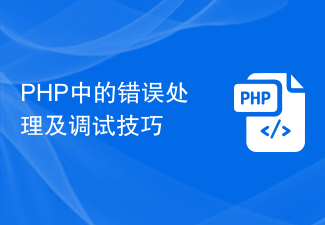
PHP is a widely used programming language, but even experienced developers will inevitably encounter errors. Therefore, it is very important to understand error handling and debugging techniques in PHP when developing code, and when debugging and maintaining existing code. This article will discuss error handling and debugging skills in PHP, including the following aspects: Error levels and types in PHP Error handling functions PHP debugging tools Common problems and solutions 1. Error levels and types in PHP In PHP, errors Divided into different levels and types.
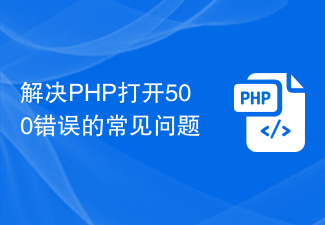
To solve the common problem of PHP opening 500 error, specific code examples are needed. In the process of developing PHP applications, 500 Internal Server Error is often encountered, which is often a headache. A 500 error means that an unrecognizable error occurred when the server was processing the request, causing the server to be unable to respond normally. It usually returns HTTP status code 500 to the client. In actual development, when encountering a 500 error, you need to carefully investigate the cause of the error and make corresponding repairs. The following will open the 500 error for PHP
