A brief discussion on Jquery core functions_jquery
In Jquery, all DOM objects will be encapsulated into Jquery objects, and only Jquery objects can use Jquery methods or properties to perform corresponding operations.
So Jquery provides a function that can encapsulate DOM objects into Jquery objects, which is the Jquery core function jquery(), also known as the factory function.
The jquery core function has 7 overloads, as follows:
jquery() This function returns an empty jquery object.
jquery(elements) This function converts one or more DOM elements into Jquery objects (or jquery collections)
jquery(callback) This function is the abbreviation of jquery(document).ready(callback). This function will bind a function that will be executed after the DOM document is loaded. All jquery operations on the page that need to be performed when the DOM is loaded need to be included in this function. This function can appear multiple times on the page.
jquery(expression,[context])
jquery(html)
jquery(html,props)
jquery(html,[ownerDocument])
Let’s take a look at them in detail
jQuery(expression, [context])
This function receives a string containing a CSS selector, and then uses this string to match a set of elements.
You can retrieve a DOM object through doc[0] and doc[1] respectively, and the others are some properties and methods unique to jQuery objects; in fact, jQuery objects wrap DOM objects and also include some operations on DOM elements. jQuery method.
In the process of using jQuery, the first step and the most important step in most cases is to obtain the jQuery object that wraps the DOM object to be manipulated; and then complete the processing by calling the method of the obtained jQuery object. Operations on DOM objects.
for example
1. Find all elements with node p in #first context, and display the corresponding values in a loop.
$(function() { var items = $("p", "#first"); $.each(items, function(i, n) { alert(i); }); });
i is the corresponding index, n is the corresponding node
2. Find all p elements, and these elements must be child elements of the div element.
HTML code:
one
two
three
jQuery code:
$("div > p");
Result:
[
two
]3. In the first form of the document, find all radio buttons (ie: input elements with type value radio).
jQuery code:
$("input:radio", document.forms[0]);
jQuery(html, [ownerDocument])
Dynamically creates a DOM element wrapped by a jQuery object based on a supplied raw HTML markup string.You can pass a handwritten HTML string, a string created by some template engine or plugin, or a string loaded via AJAX.
jQuery(html, props)
Dynamically creates a DOM element wrapped by a jQuery object based on a supplied raw HTML markup string. Set a series of properties, events, etc. at the same time.Parameters
htmlString
HTML tag string used to dynamically create DOM elements
propsMap
Properties, events and methods for attaching to newly created elements
Example
Description:
Dynamicly create a div element (and all content within it) and append it to the body element. Inside this function, markup to DOM element conversion is achieved by temporarily creating an element and setting the element's innerHTML property to the given markup string. Therefore, this function has both flexibility and limitations.
jQuery code:
$("<div>", { "class": "test", text: "Click me!", click: function(){ $(this).toggleClass("test"); } }).appendTo("body");
When the DOM is loaded, execute the functions in it.
jQuery code:
$(function(){ // 文档就绪 });

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


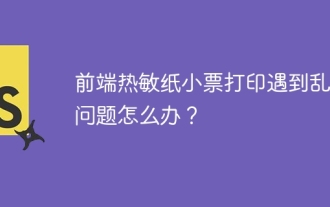
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
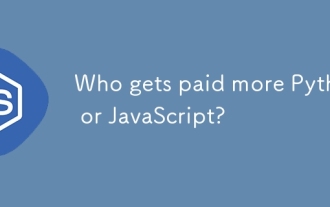
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
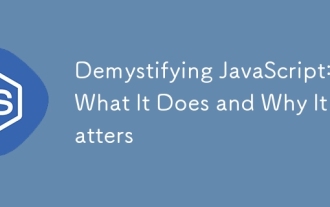
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
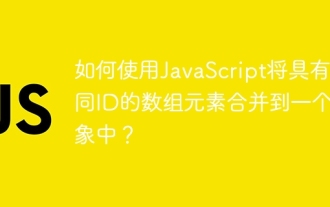
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
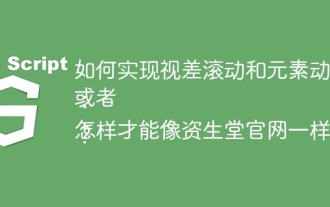
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
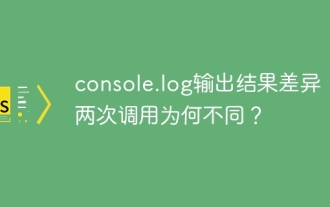
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
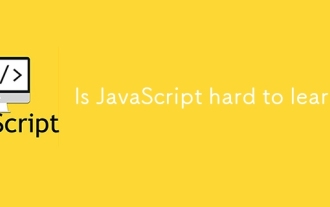
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
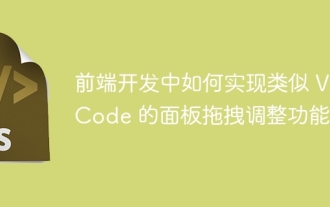
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
