Is Go language suitable for back-end development?
Is Go language suitable for back-end development?
Go language, as a statically typed programming language, has attracted widespread attention and discussion in the programming community since its inception. Its concise syntax, efficient concurrency mechanism and fast compilation speed make Go language increasingly favored by developers in the field of Web development. So, is Go language suitable for back-end development? Next, we'll explore this issue through concrete code examples.
First, let’s look at a simple back-end development example of Go language. Suppose we need to write a simple web server that can accept GET requests and return a "Hello, World!" string. The following is a code example:
package main import ( "net/http" "fmt" ) func handler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, World!") } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) }
In this code, we define a handler function to handle HTTP requests, and bind the handler function to the root path "/" through http.HandleFunc. Finally, use http.ListenAndServe to start a web server listening on port 8080. This code is concise and clear, demonstrating the simplicity and efficiency of writing back-end services in Go language.
In addition to simple examples, the Go language also has excellent performance in handling concurrent tasks. Go language provides two concurrency primitives, goroutine and channel, making it very simple to write efficient and easy-to-understand concurrency code. The following is a simple concurrency sample code:
package main import ( "fmt" "time" ) func task(done chan bool) { fmt.Println("Task started") time.Sleep(2 * time.Second) fmt.Println("Task completed") done <- true } func main() { done := make(chan bool) go task(done) <-done fmt.Println("Main function completed") }
In this code, we define a task function to simulate a time-consuming task. Concurrently execute task functions through goroutine, and notify task completion through channels. Finally, wait for the task to complete via
To sum up, it can be seen that Go language has significant advantages in back-end development. Its simple, efficient syntax and excellent concurrency processing mechanism make Go language a programming language suitable for back-end development. Of course, in actual projects, the Go language also has its limitations, such as the relatively small ecosystem. But as the Go language continues to develop and improve, I believe it will play an increasingly important role in the field of back-end development.
The above is the detailed content of Is Go language suitable for back-end development?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
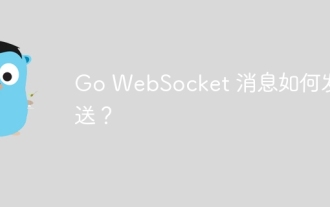
In Go, WebSocket messages can be sent using the gorilla/websocket package. Specific steps: Establish a WebSocket connection. Send a text message: Call WriteMessage(websocket.TextMessage,[]byte("Message")). Send a binary message: call WriteMessage(websocket.BinaryMessage,[]byte{1,2,3}).
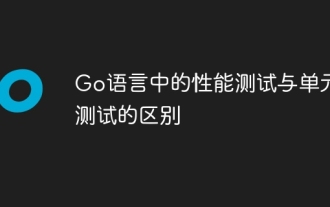
Performance tests evaluate an application's performance under different loads, while unit tests verify the correctness of a single unit of code. Performance testing focuses on measuring response time and throughput, while unit testing focuses on function output and code coverage. Performance tests simulate real-world environments with high load and concurrency, while unit tests run under low load and serial conditions. The goal of performance testing is to identify performance bottlenecks and optimize the application, while the goal of unit testing is to ensure code correctness and robustness.
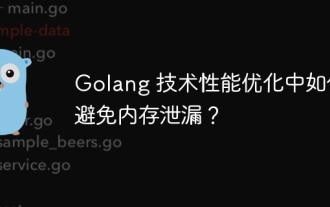
Memory leaks can cause Go program memory to continuously increase by: closing resources that are no longer in use, such as files, network connections, and database connections. Use weak references to prevent memory leaks and target objects for garbage collection when they are no longer strongly referenced. Using go coroutine, the coroutine stack memory will be automatically released when exiting to avoid memory leaks.
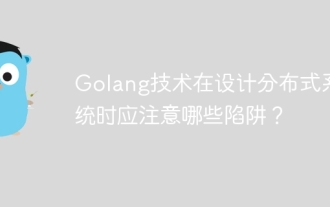
Pitfalls in Go Language When Designing Distributed Systems Go is a popular language used for developing distributed systems. However, there are some pitfalls to be aware of when using Go, which can undermine the robustness, performance, and correctness of your system. This article will explore some common pitfalls and provide practical examples on how to avoid them. 1. Overuse of concurrency Go is a concurrency language that encourages developers to use goroutines to increase parallelism. However, excessive use of concurrency can lead to system instability because too many goroutines compete for resources and cause context switching overhead. Practical case: Excessive use of concurrency leads to service response delays and resource competition, which manifests as high CPU utilization and high garbage collection overhead.
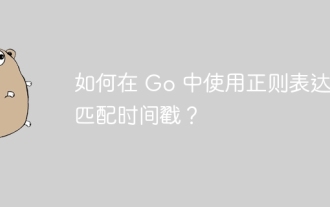
In Go, you can use regular expressions to match timestamps: compile a regular expression string, such as the one used to match ISO8601 timestamps: ^\d{4}-\d{2}-\d{2}T \d{2}:\d{2}:\d{2}(\.\d+)?(Z|[+-][0-9]{2}:[0-9]{2})$ . Use the regexp.MatchString function to check if a string matches a regular expression.
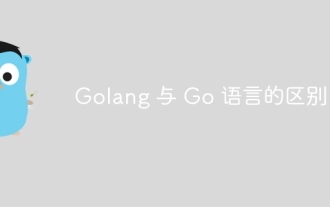
Go and the Go language are different entities with different characteristics. Go (also known as Golang) is known for its concurrency, fast compilation speed, memory management, and cross-platform advantages. Disadvantages of the Go language include a less rich ecosystem than other languages, a stricter syntax, and a lack of dynamic typing.
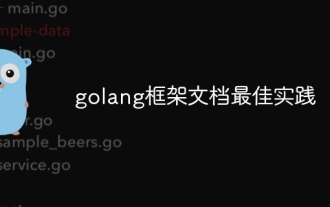
Writing clear and comprehensive documentation is crucial for the Golang framework. Best practices include following an established documentation style, such as Google's Go Coding Style Guide. Use a clear organizational structure, including headings, subheadings, and lists, and provide navigation. Provides comprehensive and accurate information, including getting started guides, API references, and concepts. Use code examples to illustrate concepts and usage. Keep documentation updated, track changes and document new features. Provide support and community resources such as GitHub issues and forums. Create practical examples, such as API documentation.
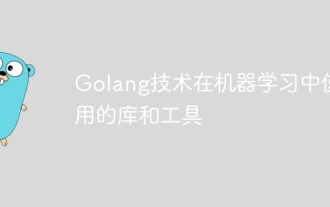
Libraries and tools for machine learning in the Go language include: TensorFlow: a popular machine learning library that provides tools for building, training, and deploying models. GoLearn: A series of classification, regression and clustering algorithms. Gonum: A scientific computing library that provides matrix operations and linear algebra functions.
