The Magic of Java JSON Processing: From Basics to Advanced
The Magic of Java JSON Processing: From Basics to Advanced JSON (JavaScript Object Notation) is a lightweight data exchange format that is widely used in web development. In Java, processing JSON data is an essential skill. This article will give you a detailed introduction to the basic knowledge and advanced techniques of JSON processing in Java by PHP editor Baicao to help you better understand and use JSON data and improve development efficiency.
JSON (javascript Object Notation) is a lightweight data exchange format widely used in WEB Development and data transfer. Java is a popular programming language that provides a rich set of libraries and frameworks. By using these libraries, Java developers can easily parse and generate jsON data.
Parsing JSON
Parsing JSON data refers to converting JSON strings into Java objects. There are two popular Java libraries available for this purpose:
- Jackson: Jackson is a powerful JSON processing library that provides a variety of features, including annotation binding, custom serialization and deserialization.
- Gson: Gson is a simple JSON processing library known for its ease of use and performance.
Code example: Parsing JSON using Jackson
import com.fasterxml.jackson.databind.ObjectMapper; public class JSONParsingDemo { public static void main(String[] args) throws Exception { String json = "{"name": "John Doe", "age": 30}"; ObjectMapper mapper = new ObjectMapper(); Customer customer = mapper.readValue(json, Customer.class); System.out.println(customer.getName()); // 输出:John Doe System.out.println(customer.getAge()); // 输出:30 } } class Customer { private String name; private int age; // getters and setters... }
Generate JSON
Generating JSON data refers to converting Java objects into JSON strings. Again, both Jackson and Gson can achieve this.
Code example: Generating JSON using Gson
import com.Google.gson.Gson; public class JSONGenerationDemo { public static void main(String[] args) { Customer customer = new Customer("John Doe", 30); Gson gson = new Gson(); String json = gson.toJson(customer); System.out.println(json); // 输出:{"name":"John Doe","age":30} } }
Advanced Tips
In addition to basic functions, Java also provides some advanced techniques to enhance JSON processing.
Annotation Binding
Annotation binding allows direct mapping of Java class properties to JSON fields.
Code Example: Using Jackson Annotation Binding
@JsonProperty("customer_name") private String name; @JsonProperty("customer_age") private int age;
Custom serialization/deserialization
Sometimes it is necessary to customize the serialization or deserialization process. Both Jackson and Gson provide extension points for this purpose.
Streaming API
Streaming api Allows JSON data to be parsed and generated character by character, improving efficiency in processing large data sets.
Performance optimization
By performance optimization for JSON processing, the overall performance of Java applications can be improved.
- Reuse JSON parsers and generators using object pools.
- CacheThe parsed JSON object to avoid repeated parsing.
- Enable lazy loading to defer JSON parsing until needed.
in conclusion
Mastering the skills of Java JSON processing is critical to building robust and efficient web services and applications. By understanding basic concepts and advanced techniques, Java developers can harness the magic of JSON processing and get the most value from data exchange.
The above is the detailed content of The Magic of Java JSON Processing: From Basics to Advanced. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
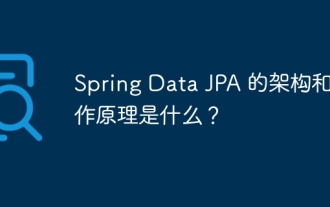
SpringDataJPA is based on the JPA architecture and interacts with the database through mapping, ORM and transaction management. Its repository provides CRUD operations, and derived queries simplify database access. Additionally, it uses lazy loading to only retrieve data when necessary, thus improving performance.

Article keywords: JavaJPA performance optimization ORM entity management JavaJPA (JavaPersistance API) is an object-relational mapping (ORM) framework that allows you to use Java objects to operate data in the database. JPA provides a unified API for interacting with databases, allowing you to use the same code to access different databases. In addition, JPA also supports features such as lazy loading, caching, and dirty data detection, which can improve application performance. However, if used incorrectly, JPA performance can become a bottleneck for your application. The following are some common performance problems: N+1 query problem: When you use JPQL queries in your application, you may encounter N+1 query problems. In this kind of
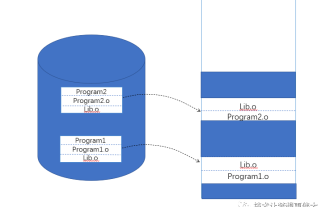
As usual, let’s ask a few questions: Why dynamic linking? How to do dynamic linking? What is address-independent code technology? What is delayed binding technology? How to do explicit linking while the program is running? Why dynamic linking? The emergence of dynamic linking is to solve some shortcomings of static linking: saving memory and disk space: As shown in the figure below, Program1 and Program2 contain two modules, Program1.o and Program2.o respectively, and they both require the Lib.o module. In the case of static linking, both target files use the Lib.o module, so they have copies in the executable files Program1 and program2 output by the link and run at the same time.

Decoding Laravel performance bottlenecks: Optimization techniques fully revealed! Laravel, as a popular PHP framework, provides developers with rich functions and a convenient development experience. However, as the size of the project increases and the number of visits increases, we may face the challenge of performance bottlenecks. This article will delve into Laravel performance optimization techniques to help developers discover and solve potential performance problems. 1. Database query optimization using Eloquent delayed loading When using Eloquent to query the database, avoid
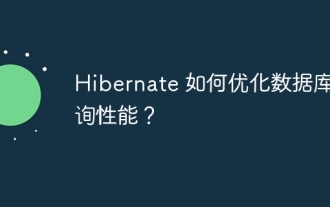
Tips for optimizing Hibernate query performance include: using lazy loading to defer loading of collections and associated objects; using batch processing to combine update, delete, or insert operations; using second-level cache to store frequently queried objects in memory; using HQL outer connections , retrieve entities and their related entities; optimize query parameters to avoid SELECTN+1 query mode; use cursors to retrieve massive data in blocks; use indexes to improve the performance of specific queries.
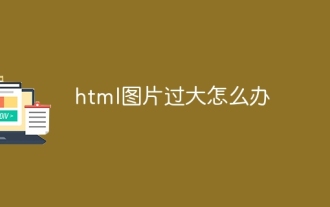
Here are some ways to optimize HTML images that are too large: Optimize image file size: Use a compression tool or image editing software. Use media queries: Dynamically resize images based on device. Implement lazy loading: only load the image when it enters the visible area. Use a CDN: Distribute images to multiple servers. Use image placeholder: Display a placeholder image while the image is loading. Use thumbnails: Displays a smaller version of the image and loads the full-size image on click.
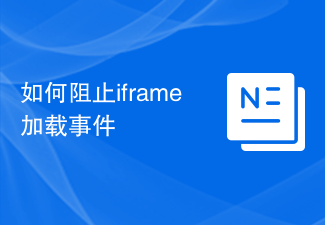
How to prevent iframe loading events In web development, we often use iframe tags to embed other web pages or content. By default, when the browser loads an iframe, the loading event is triggered. However, in some cases we may want to delay the loading of an iframe, or prevent the loading event entirely. In this article, we'll explore how to achieve this through code examples. 1. Delay loading of iframe If you want to delay loading of iframe, we can use
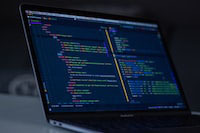
In the field of Java programming, JPA (JavaPersistence API), as a popular persistence framework, provides developers with a convenient way to operate relational databases. By using JPA, developers can easily persist Java objects into the database and retrieve data from the database, thus greatly improving application development efficiency and maintainability. This article carefully selects 10 high-quality JavaJPA open source projects, covering a variety of different functions and application scenarios, aiming to provide developers with more inspiration and solutions to help create more efficient and reliable applications. These projects include: SpringDataJPA: springDataJPA is the Spr
