How to hide unwanted database interfaces in PHP?
Hide unnecessary database interfaces in PHP is very important, especially when developing web applications. By hiding unnecessary database interfaces, you can increase program security and prevent malicious users from using these interfaces to attack the database. The following will introduce how to hide unnecessary database interfaces in PHP and provide specific code examples.
- Use PDO (PHP Data Objects) in PHP to connect to the database
PDO is an extension for connecting to the database in PHP. It provides a unified interface that can be used with Interact with multiple types of databases, such as MySQL, SQLite, PostgreSQL, etc. Using PDO effectively hides database details while also providing better security.
The following is an example of PDO connecting to a MySQL database:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
|
- Use PDO prepared statements to prevent SQL injection attacks
SQL injection attacks are a A common database attack method, hackers can obtain sensitive information of the database by entering malicious SQL statements in the input box. To prevent this from happening, you can use PDO prepared statements.
The following is an example of using PDO prepared statements to query the database:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
|
In the above code, the prepare method of PDO is used to prepare the query statement, and then the bindParam method is used to bind Enter the parameters and finally execute the query statement. This can effectively prevent SQL injection attacks.
- Use security functions in PHP to filter user input
When processing user-entered data, you should use security functions in PHP to filter and validate data to Prevent malicious user input from causing database attacks. The following are some examples of commonly used PHP security functions:
- htmlspecialchars function: Convert special characters into HTML entities to prevent XSS attacks.
- filter_var function: filter and verify variables, you can specify filtering rules, such as FILTER_SANITIZE_EMAIL, FILTER_SANITIZE_URL, etc.
- mysqli_real_escape_string function: Escape strings to prevent SQL injection attacks.
1 2 3 4 5 |
|
The above is an introduction and code example on how to hide unnecessary database interfaces in PHP. By using PDO to connect to the database and using PDO preprocessing statements and security functions to filter user input, you can effectively increase the security of the program and prevent database attacks. Hope the above content can be helpful to you.
The above is the detailed content of How to hide unwanted database interfaces in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


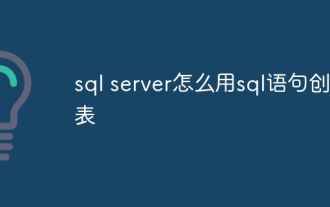
How to create tables using SQL statements in SQL Server: Open SQL Server Management Studio and connect to the database server. Select the database to create the table. Enter the CREATE TABLE statement to specify the table name, column name, data type, and constraints. Click the Execute button to create the table.
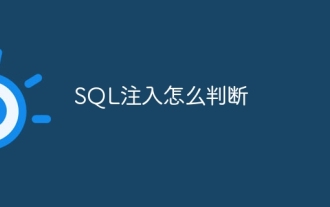
Methods to judge SQL injection include: detecting suspicious input, viewing original SQL statements, using detection tools, viewing database logs, and performing penetration testing. After the injection is detected, take measures to patch vulnerabilities, verify patches, monitor regularly, and improve developer awareness.
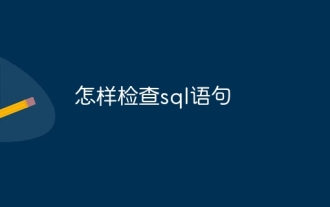
The methods to check SQL statements are: Syntax checking: Use the SQL editor or IDE. Logical check: Verify table name, column name, condition, and data type. Performance Check: Use EXPLAIN or ANALYZE to check indexes and optimize queries. Other checks: Check variables, permissions, and test queries.
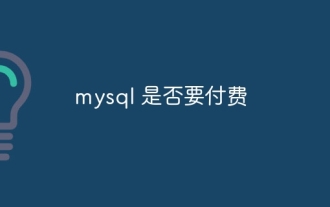
MySQL has a free community version and a paid enterprise version. The community version can be used and modified for free, but the support is limited and is suitable for applications with low stability requirements and strong technical capabilities. The Enterprise Edition provides comprehensive commercial support for applications that require a stable, reliable, high-performance database and willing to pay for support. Factors considered when choosing a version include application criticality, budgeting, and technical skills. There is no perfect option, only the most suitable option, and you need to choose carefully according to the specific situation.
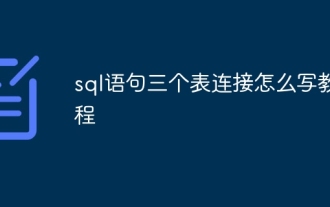
This article introduces a detailed tutorial on joining three tables using SQL statements to guide readers step by step how to effectively correlate data in different tables. With examples and detailed syntax explanations, this article will help you master the joining techniques of tables in SQL, so that you can efficiently retrieve associated information from the database.
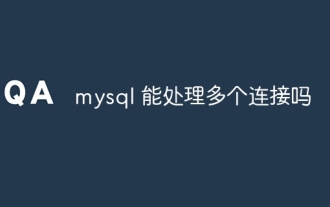
MySQL can handle multiple concurrent connections and use multi-threading/multi-processing to assign independent execution environments to each client request to ensure that they are not disturbed. However, the number of concurrent connections is affected by system resources, MySQL configuration, query performance, storage engine and network environment. Optimization requires consideration of many factors such as code level (writing efficient SQL), configuration level (adjusting max_connections), hardware level (improving server configuration).
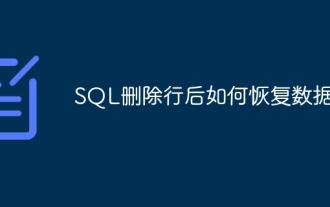
Recovering deleted rows directly from the database is usually impossible unless there is a backup or transaction rollback mechanism. Key point: Transaction rollback: Execute ROLLBACK before the transaction is committed to recover data. Backup: Regular backup of the database can be used to quickly restore data. Database snapshot: You can create a read-only copy of the database and restore the data after the data is deleted accidentally. Use DELETE statement with caution: Check the conditions carefully to avoid accidentally deleting data. Use the WHERE clause: explicitly specify the data to be deleted. Use the test environment: Test before performing a DELETE operation.
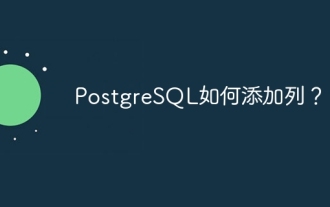
PostgreSQL The method to add columns is to use the ALTER TABLE command and consider the following details: Data type: Select the type that is suitable for the new column to store data, such as INT or VARCHAR. Default: Specify the default value of the new column through the DEFAULT keyword, avoiding the value of NULL. Constraints: Add NOT NULL, UNIQUE, or CHECK constraints as needed. Concurrent operations: Use transactions or other concurrency control mechanisms to handle lock conflicts when adding columns.
