Detailed explanation of parameters and usage of input method in Laravel
Title: Detailed explanation of parameters and usage of input method in Laravel
Laravel is a popular PHP framework that is widely used in web development. In Laravel, handling user input is a very important task. Among them, the input method is a convenient and easy-to-use method for obtaining input data in HTTP requests. This article will explain in detail the parameters and usage of the input method in Laravel, with specific code examples.
1. Basic usage of the input method
In Laravel, we can use the input method to obtain parameters in the HTTP request. Through the input method, we can easily access request data of types such as GET, POST, and JSON.
The following is a basic usage example of the input method:
use IlluminateHttpRequest; public function index(Request $request) { $name = $request->input('name'); $email = $request->input('email'); // 处理业务逻辑 }
In the above example, we first inject the $request object through the Request class, and then use the input method to get the incoming parameters. Here, we get the parameters named name
and email
.
2. Get specific parameters
If we want to get specific parameters instead of multiple parameters, we can add a second parameter to the input method as a default value. If this parameter is not included in the request, the default value we set will be returned.
$name = $request->input('name', 'Guest');
In the above example, if there is no parameter named name
in the request, $name will be assigned the value 'Guest'.
3. Check whether a parameter exists
Sometimes we need to check whether a parameter exists, we can use the has method.
if ($request->has('name')) { // 存在name参数 }
4. Get all parameters
If we want to get all input parameters, we can use the all method.
$inputs = $request->all();
In this way we can obtain all input parameters at once and perform further processing.
5. Get some parameters
Sometimes we only need to get some of the input parameters, you can use the only method.
$inputs = $request->only(['name', 'email']);
Through the above code, we only obtain the input parameters named name
and email
.
6. Exclude specific parameters
The method corresponding to only is except, which can be used to exclude specific parameters.
$inputs = $request->except(['password']);
Through the above code, we exclude the parameter named password
and obtain all input parameters except this.
Conclusion
The above is a detailed explanation of the parameters and usage of the input method in Laravel. Through the input method, we can easily obtain the input data in the HTTP request and perform further processing. I hope this article can help readers better understand and apply the input method in the Laravel framework.
The above is the detailed content of Detailed explanation of parameters and usage of input method in Laravel. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


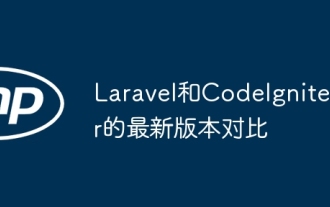
The latest versions of Laravel 9 and CodeIgniter 4 provide updated features and improvements. Laravel9 adopts MVC architecture and provides functions such as database migration, authentication and template engine. CodeIgniter4 uses HMVC architecture to provide routing, ORM and caching. In terms of performance, Laravel9's service provider-based design pattern and CodeIgniter4's lightweight framework give it excellent performance. In practical applications, Laravel9 is suitable for complex projects that require flexibility and powerful functions, while CodeIgniter4 is suitable for rapid development and small applications.
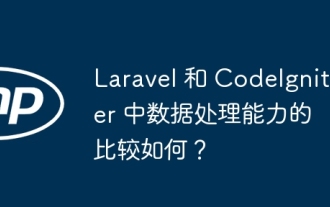
Compare the data processing capabilities of Laravel and CodeIgniter: ORM: Laravel uses EloquentORM, which provides class-object relational mapping, while CodeIgniter uses ActiveRecord to represent the database model as a subclass of PHP classes. Query builder: Laravel has a flexible chained query API, while CodeIgniter’s query builder is simpler and array-based. Data validation: Laravel provides a Validator class that supports custom validation rules, while CodeIgniter has less built-in validation functions and requires manual coding of custom rules. Practical case: User registration example shows Lar
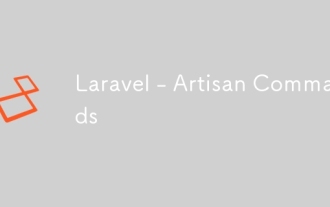
Laravel - Artisan Commands - Laravel 5.7 comes with new way of treating and testing new commands. It includes a new feature of testing artisan commands and the demonstration is mentioned below ?
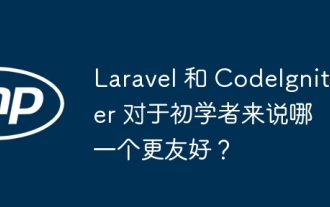
For beginners, CodeIgniter has a gentler learning curve and fewer features, but covers basic needs. Laravel offers a wider feature set but has a slightly steeper learning curve. In terms of performance, both Laravel and CodeIgniter perform well. Laravel has more extensive documentation and active community support, while CodeIgniter is simpler, lightweight, and has strong security features. In the practical case of building a blogging application, Laravel's EloquentORM simplifies data manipulation, while CodeIgniter requires more manual configuration.
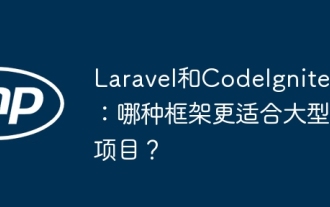
When choosing a framework for large projects, Laravel and CodeIgniter each have their own advantages. Laravel is designed for enterprise-level applications, offering modular design, dependency injection, and a powerful feature set. CodeIgniter is a lightweight framework more suitable for small to medium-sized projects, emphasizing speed and ease of use. For large projects with complex requirements and a large number of users, Laravel's power and scalability are more suitable. For simple projects or situations with limited resources, CodeIgniter's lightweight and rapid development capabilities are more ideal.
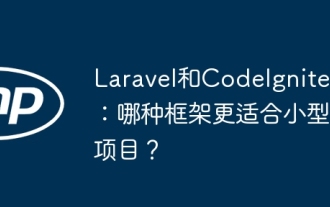
For small projects, Laravel is suitable for larger projects that require strong functionality and security. CodeIgniter is suitable for very small projects that require lightweight and ease of use.
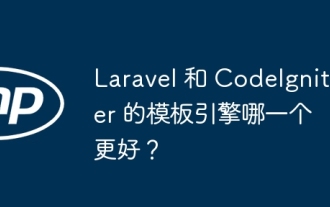
Comparing Laravel's Blade and CodeIgniter's Twig template engine, choose based on project needs and personal preferences: Blade is based on MVC syntax, which encourages good code organization and template inheritance. Twig is a third-party library that provides flexible syntax, powerful filters, extended support, and security sandboxing.
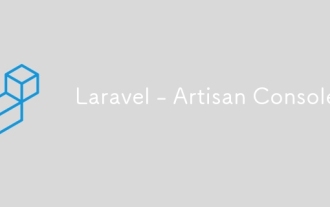
Laravel - Artisan Console - Laravel framework provides three primary tools for interaction through command-line namely: Artisan, Ticker and REPL. This chapter explains about Artisan in detail.
