


Common Pitfalls with PHP ZipArchive Extensions: Avoid Common Mistakes
PHP ZipArchive extension is a very useful tool when processing compressed files, but you will also encounter some common pitfalls during use. PHP editor Strawberry will introduce to you how to avoid these common mistakes and help you use the ZipArchive extension to perform file compression and decompression operations more smoothly. By studying this article, you will be able to avoid some common ZipArchive extension usage errors, improve PHP development efficiency, and ensure the normal operation of the program.
When operating on compressed files using the ZipArcHive object, you must always ensure that the file handle is closed after the operation is completed. Failure to close a file handle can result in file corruption or resource leaks.
$zip = new ZipArchive(); $zip->open("file.zip"); // ... 进行操作 ... $zip->close(); // 关闭文件句柄
Trap 2: Processing unqualified files
ZipArchive will throw an exception if the file to be opened is damaged or does not conform to the ZIP specification. Documents should be checked for validity before being processed.
if ($zip->open("file.zip") !== TRUE) { // 处理异常 }
Trap 3: Byte Order Mark (BOM) Not Considered
If you are processing text files from different sources, you may encounter the byte order mark (BOM), which is an optional character that indicates the byte order of the text file. UTF-8 encoded files usually contain a BOM, while other encodings do not. ZipArchive may interpret the BOM as part of the file contents, causing decompression errors. To avoid this, use the ZipArchive::setExternalAttributesName() method to specify how the BOM is handled.
$zip->setExternalAttributesName(ZipArchive::FL_NODIR_ATTRIBUTES);
Trap 4: Unsupported compression method
ZipArchive supports multiple compression methods such as Deflate, Bzip2 and LZMA. However, some older systems may not support all of these methods. You should use the Deflate compression method if you plan to distribute ZIP files on systems that do not support these methods.
$zip->addFile("file.txt", "file.txt"); $zip->setCompressionIndex(ZIPARCHIVE::CM_DEFLATE);
Trap 5: Operating non-existent entries
When using the ZipArchive object to operate an entry in a compressed file, be sure to ensure that the entry exists. Otherwise, ZipArchive will throw an exception. Check the existence of an entry using the ZipArchive::locateName() method.
if ($zip->locateName("file.txt") === -1) { // 条目不存在,处理错误 }
Trap 6: Unhandled Error
Various errors may occur when using ZipArchive. Always check the ZipArchive object for error codes and take appropriate action if an error occurs.
switch ($zip->getStatus()) { case ZIPARCHIVE::ER_OK: // 没有错误 break; case ZIPARCHIVE::ER_NOZIP: // 并非 ZIP 文件 break; case ZIPARCHIVE::ER_INVAL: // 无效的 ZIP 文件 break; // ... 其他错误处理 ... }
Trap 7: Using indexes instead of names
ZipArchive supports using index or name to access entries in archives. However, using indexes can be risky. The index may change if entries are reordered or deleted. It is better to access the entry by name as it is less susceptible to modification of the compressed file.
$entry = $zip->getEntry("file.txt"); // 使用名称 $entry = $zip->getEntryByIndex(0); // 使用索引 (不推荐)
Trap 8: Handling symbolic links
ZipArchive does not support symbolic links. If you encounter a symbolic link in a compressed file, ZipArchive treats it as a normal file. In order to handle symbolic links, you need to use an external tool or library.
Trap 9: File size limits not taken into account
ZipArchive has file size limits that depend on the operating system used. Before adding large files to a compressed file, check the file size limit.
if ($filesize > 2e9) { // 2 GB // 超过文件大小限制,处理错误 }
Trap 10: Using temporary files
In some cases, you may need to use ZipArchive objects with temporary files. Please be sure to delete temporary files after use to free up system resources and avoid security issues.
// 创建临时文件 $tmpfile = tmpfile(); // 将 ZipArchive 对象与临时文件关联 $zip->open($tmpfile); // ... 进行操作 ... // 删除临时文件 fclose($tmpfile);
By avoiding these common pitfalls, you can ensure that your PHP ZipArchive code runs smoothly. By following these best practices, you can process compressed files efficiently and reliably.
The above is the detailed content of Common Pitfalls with PHP ZipArchive Extensions: Avoid Common Mistakes. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


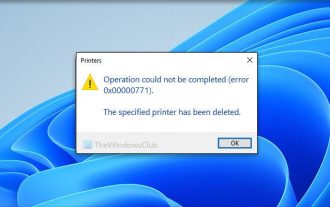
If you encounter an error message when using your printer, such as the operation could not be completed (error 0x00000771), it may be because the printer has been disconnected. In this case, you can solve the problem through the following methods. In this article, we will discuss how to fix this issue on Windows 11/10 PC. The entire error message says: The operation could not be completed (error 0x0000771). The specified printer has been deleted. Fix 0x00000771 Printer Error on Windows PC To fix Printer Error the operation could not be completed (Error 0x0000771), the specified printer has been deleted on Windows 11/10 PC, follow this solution: Restart Print Spool
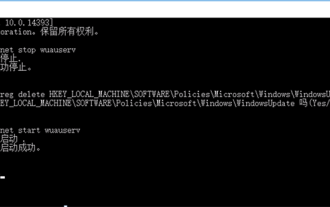
Table of Contents Solution 1 Solution 21. Delete the temporary files of Windows update 2. Repair damaged system files 3. View and modify registry entries 4. Turn off the network card IPv6 5. Run the WindowsUpdateTroubleshooter tool to repair 6. Turn off the firewall and other related anti-virus software. 7. Close the WidowsUpdate service. Solution 3 Solution 4 "0x8024401c" error occurs during Windows update on Huawei computers Symptom Problem Cause Solution Still not solved? Recently, the web server needs to be updated due to system vulnerabilities. After logging in to the server, the update prompts error code 0x8024401c. Solution 1
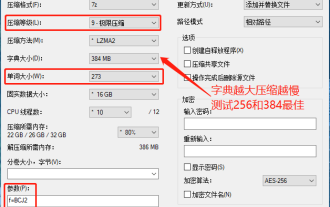
I found that the compressed package downloaded from a download website will be larger than the original compressed package after decompression. The difference is tens of Kb for a small one and several dozen Mb for a large one. If it is uploaded to a cloud disk or paid space, it does not matter if the file is small. , if there are many files, the storage cost will be greatly increased. I studied it specifically and can learn from it if necessary. Compression level: 9-Extreme compression Dictionary size: 256 or 384, the more compressed the dictionary, the slower it is. The compression rate difference is larger before 256MB, and there is no difference in compression rate after 384MB. Word size: maximum 273 Parameters: f=BCJ2, test and add parameter compression rate will be higher
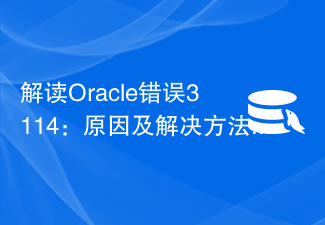
Title: Analysis of Oracle Error 3114: Causes and Solutions When using Oracle database, you often encounter various error codes, among which error 3114 is a relatively common one. This error generally involves database link problems, which may cause exceptions when accessing the database. This article will interpret Oracle error 3114, discuss its causes, and give specific methods to solve the error and related code examples. 1. Definition of error 3114 Oracle error 3114 pass
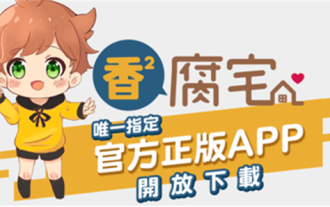
The display error is a problem that may occur in the Xiangxiang Fuzhai app. Some users are not sure why the Xiangxiang Fuzhai app displays errors. It may be due to network connection problems, too many background programs, incorrect registration information, etc. Next, This is the editor’s introduction to how to solve app display errors for users. Interested users should come and take a look! Why does the Xiangxiang Fuzhai app display an error answer: network connection problem, too many background programs, incorrect registration information, etc. Details: 1. [Network problem] Solution: Check the device connection network status, reconnect or choose another network connection to use. Can. 2. [Too many background programs] Solution: Close other running programs and release the system, which can speed up the running of the software. 3. [Incorrect registration information
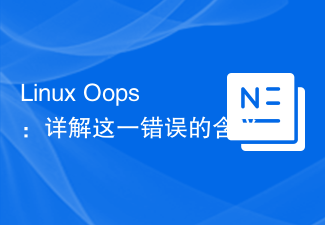
LinuxOops: Detailed explanation of the meaning of this error, need specific code examples What is LinuxOops? In Linux systems, "Oops" refers to a situation where a serious error in the kernel causes the system to crash. Oops is actually a kernel crash mechanism that stops the system when a fatal error occurs and prints out relevant error information so that developers can diagnose and fix the problem. Oops usually occur in kernel space and have nothing to do with user space applications. When the kernel encounters
![Streaming server throws error [FIXED]](https://img.php.cn/upload/article/000/465/014/171133083740341.jpg?x-oss-process=image/resize,m_fill,h_207,w_330)
Are you getting a streaming server throwing an error on your computer? Stremio is a cross-platform video streaming service that you can use to stream and watch movies, TV shows, live TV, and more. Some users have reported getting this error when trying to launch the application. Errors can occur under a variety of circumstances, including Internet and server issues. This error may also be encountered when using the web version of Stremio. Interference from antivirus software or firewalls can also cause errors to occur. Whatever the case, you can fix the problem with this guide. Streaming Server Throws Error While running Stremio application on Windows, you may encounter “Stremio Streaming Server threw error
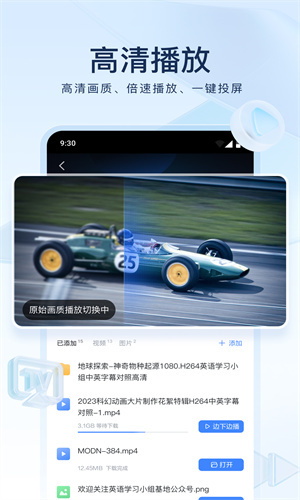
When we use this platform, we can use the method of free decompression of files, so that we can open some compressed packages we downloaded, and of course there are many files, etc., which can be understood directly from the above. Obtained, many users may not know much about this, so this also allows us to better use some functions. This is also more convenient for us when downloading other files in the future. It can be used better, and the effect is still good, so today I will bring you a variety of completely different usage effects. Why haven’t you come to know about such a useful function? For those of you who don’t know it yet, Friends, hurry up and take a look at some of the strategy explanations below. You can’t miss this excitement.
