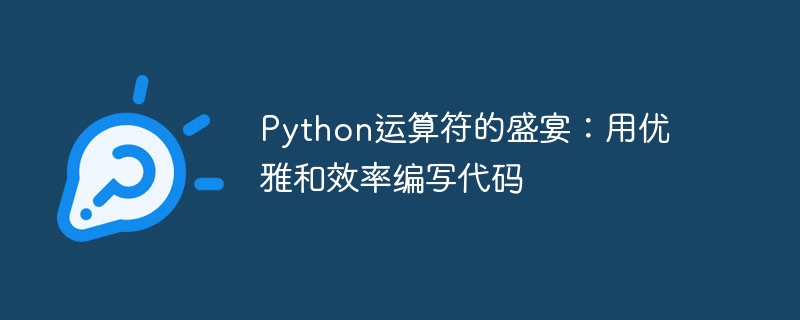
python, operator, mathematics, comparison, assignment
Mathematical operators
Python provides a series of mathematical operators for performing basic mathematical operations:
-
Addition ( ): Add two numbers
-
Subtraction (-): Subtract two numbers
- *Multiplication (): **Multiply two numbers
-
Division (/): Divide two numbers (the result is a floating point number)
-
Integer division (//): Divide two numbers (the result is an integer)
-
Modulo (%):Find the remainder after dividing two numbers
a = 5
b = 2
print(a + b)# 输出:7
print(a - b)# 输出:3
print(a * b)# 输出:10
print(a / b)# 输出:2.5
print(a // b)# 输出:2
print(a % b)# 输出:1
Copy after login
Comparison operators
Comparison operators are used to compare the values of two expressions:
- Equals (==): Checks whether two expressions are equal
- Not equal to (!=): Checks whether two expressions are not equal
- Greater than (>): Check whether the first expression is greater than the second expression
- Greater than or equal to (>=): Checks whether the first expression is greater than or equal to the second expression
- Less than (<): Check whether the first expression is less than the second expression
- Less than or equal to (<=): Checks whether the first expression is less than or equal to the second expression
a = 5
b = 2
print(a == b)# 输出:False
print(a != b)# 输出:True
print(a > b)# 输出:True
print(a >= b)# 输出:True
print(a < b)# 输出:False
print(a <= b)# 输出:False
Copy after login
Assignment operator
The assignment operator is used to assign a value to a variable:
- Assignment (=): Assign the value on the right to the variable on the left
- Additional assignment (=):Add the value on the right to the variable on the left
- Subtractive assignment (-=): Subtract the value on the right from the variable on the left
- *Multiplicative assignment (=): **Multiply the value on the right by the variable on the left
- Division assignment (/=): Divide the variable on the left by the value on the right
- Integer division assignment (//=): Divide the variable on the left by the value on the right (the result is an integer)
- Modulo assignment (%=): Take the variable on the left modulo and use the value on the right as the divisor
a = 5
a += 2# 等价于 a = a + 2
print(a)# 输出:7
Copy after login
Logical Operators
Logical operators are used to check the value of Boolean expressions:
- With (and): Check that all expressions are True only when they are True
- Or (or): Check that any expression is True if it is True
- Not (not): Reverse the value of the Boolean expression
a = True
b = False
print(a and b)# 输出:False
print(a or b)# 输出:True
print(not a)# 输出:False
Copy after login
Other operators
In addition to the above operators, Python also provides some other useful operators:
-
Identity operator (is, is not): Check whether two objects point to the same object
-
Member operator (in, not in): Check whether a value is contained in a sequence
-
Range operator (range): Create a range object, used to generate a sequence of numbers
By flexibly using these operators, programmers can create Python code that is concise, efficient, and highly readable.
The above is the detailed content of A feast of Python operators: Write code with elegance and efficiency. For more information, please follow other related articles on the PHP Chinese website!