


Uncovering the Power of Python Operators: Writing Elegant and Efficient Code
python Operators are a key component of programming languages, enabling developers to perform a wide range of operations, From simple arithmetic to complex bit operations. Mastering the syntax, semantics, and functionality of operators is critical to using Python effectively.
Arithmetic operators
Arithmetic operators are used to perform basic arithmetic operations. They include addition ( ), subtraction (-), multiplication (*), division (/), modulo (%), exponentiation (**), and floor division (//). The following example demonstrates the use of arithmetic operators:
>> a = 10 >> b = 5 # 加法 c = a + b print(c)# 输出:15 # 减法 c = a - b print(c)# 输出:5 # 乘法 c = a * b print(c)# 输出:50
Comparison operators
Comparison operators are used to compare two values. They include equal (==), not equal (!=), less than (<), greater than (>), less than or equal to (<=), and greater than or equal to (>=). Comparison operators return Boolean values (True or False). The following example demonstrates the use of comparison operators:
>> a = 10 >> b = 5 # 相等 print(a == b)# 输出:False # 不等于 print(a != b)# 输出:True # 小于 print(a < b)# 输出:False
Logical Operators
Logical operators are used to combine Boolean values. They include logical not (not), logical AND (and), and logical OR (or). Logical operators return Boolean values. The following example demonstrates the use of logical operators:
>> a = True >> b = False # 逻辑非 print(not a)# 输出:False # 逻辑与 print(a and b)# 输出:False # 逻辑或 print(a or b)# 输出:True
Assignment operator
Assignment operator is used to assign a value to a variable. They include simple assignment (=), additive assignment (=), subtractive assignment (-=), multiplicative assignment (*=), division assignment (/=), and modulo assignment (%=). Assignment operators perform operations and return results. The following example demonstrates the use of the assignment operator:
>> a = 10 # 简单赋值 b = a print(b)# 输出:10 # 加法赋值 a += 5 print(a)# 输出:15
Bitwise operators
Bitwise operators are used to perform bit operations. They include bitwise AND (&), bitwise OR (|), bitwise XOR (^), bitwise complement (~), and left shift (<<) and right shift (>>). Bitwise operators return integers. The following example demonstrates the use of bitwise operators:
>> a = 10 >> b = 5 # 位与 print(a & b)# 输出:0 # 位或 print(a | b)# 输出:15 # 位异或 print(a ^ b)# 输出:15
Member operator
The membership operator is used to test whether the element belongs to a set or sequence. They include in and not in. Member operators return Boolean values. The following example demonstrates the use of membership operators:
>> my_list = [1, 2, 3] # in if 2 in my_list: print("2 is in the list")# 输出:2 is in the list # not in if 4 not in my_list: print("4 is not in the list")# 输出:4 is not in the list
in conclusion
Python operators provide a wide range of powerful features that enable developers to write elegant and efficient code. By understanding and becoming proficient in using these operators, developers can take full advantage of Python's capabilities and create maintainable, readable, and performant applications. Through continued practice and exploration, developers can master the full potential of Python operators and improve their programming skills.
The above is the detailed content of Uncovering the Power of Python Operators: Writing Elegant and Efficient Code. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


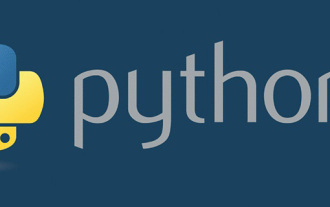
In Python, "+=" refers to the "addition assignment" operator, which is a type of assignment operator. Its function is to perform an addition operation first, and then assign the result to the variable on the left side of the operator; the syntax is "x += y", the equivalent form is "x = x + y". The "+=" operator can only assign values to existing variables, because the variable itself needs to participate in the operation during the assignment process. If the variable is not defined in advance, its value is unknown and cannot participate in the operation.
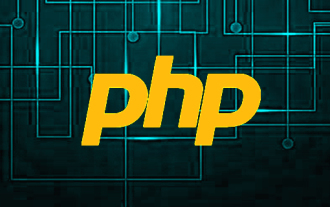
In PHP, the "==" symbol is a comparison operator that can compare whether two operands are equal. The syntax is "operand 1 == operand 2". The "==" operator compares and tests whether the variable on the left (expression or constant) has the same value as the variable on the right (expression or constant); it only compares the values of the variables, not the data types. If the two values are the same, it returns a true value; if the two values are not the same, it returns a false value.
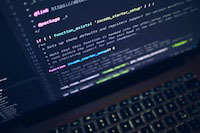
Introduction to python operators Operators are special symbols or keywords used to perform operations between two or more operands. Python provides a variety of operators covering a wide range of uses, from basic mathematical operations to complex data manipulation. Mathematical operators Mathematical operators are used to perform common mathematical operations. They include: operator operation examples + addition a + b - subtraction a-b * multiplication a * b / division a / b % modulo operation (take the remainder) a % b ** power operation a ** b // integer division (discard the remainder) a//b Logical Operators Logical operators are used to concatenate Boolean values and evaluate conditions. They include: operator operations examples and logical and aandbor logical or aorbnot logical not nota comparison operations
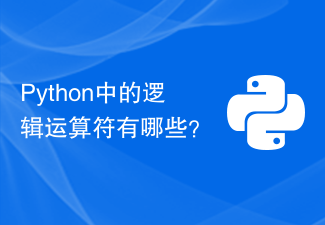
What are the logical operators in Python? Logical operators in Python are used to logically compare expressions and return a Boolean value (True or False). There are three commonly used logical operators in Python: and, or and not. and operator and operator is used to check whether all operands are true (True). The and operator returns True only if all operands are true; otherwise it returns False. Here is a sample code: a=10b=
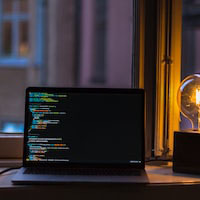
The Secret Garden of Operators Python operators are symbols or keywords used to perform various operations. They enable developers to express complex logic concisely and clearly and improve code efficiency. Python provides a wide range of operator types, each with its specific purpose and usage. Logical Operators Logical operators are used to combine Boolean values and perform logical operations. The main ones are: and: Returns the Boolean value True, if all operands are True, otherwise it returns False. or: Returns a Boolean value True if any operand is True, otherwise returns False. not: Negate the Boolean value, change True to False, and change False to True. Demo code: x=Truey
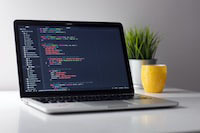
Python operators are a key component of the programming language, enabling developers to perform a wide range of operations, from simple arithmetic to complex bit manipulation. Mastering the syntax, semantics, and functionality of operators is essential to using Python effectively. Arithmetic Operators Arithmetic operators are used to perform basic arithmetic operations. They include addition (+), subtraction (-), multiplication (*), division (/), modulo (%), exponentiation (**), and floor division (//). The following example demonstrates the use of arithmetic operators: >>a=10>>b=5#Addition c=a+bprint(c)#Output: 15#Subtraction c=a-bprint(c)#Output: 5#Multiplication c=a*bprint(c)#output
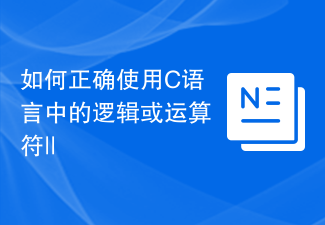
Title: How to correctly use the logical OR operator || in C language. In C language, the logical OR operator || is a commonly used logical operator, which is used to determine whether any of the conditions is true. Proper use of logical OR operators can help us write more concise and efficient code. The following will introduce in detail how to correctly use the logical OR operator || in C language and provide specific code examples. The basic syntax of the logical OR operator || is: expression 1 || expression 2. When either expression1 or expression2
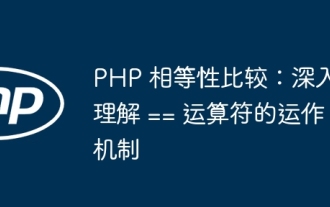
Equality comparison in PHP involves the == operator. It has two types: strict comparison (===) and non-strict comparison (==). The latter can produce unexpected results because variables of different types can be converted to the same type and then compared. To ensure that values are equal and of the same type, use strict comparison.
