


Explore the practical applications of the Go programming language in software development
Go language (also known as Golang) is a programming language developed by Google. It is efficient, concise and easy to learn, and is increasingly popular among developers. In software development, Go language can be widely used in server-side development, cloud computing, network programming, big data processing and other fields. This article will explore the practical application of the Go programming language in software development and provide some specific code examples.
1. Server-side development
The Go language performs well in server-side development. Its concurrency model and high performance make it a powerful tool for developing Web services and APIs. Here is a simple HTTP server example:
package main import ( "fmt" "net/http" ) func handler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, World!") } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) }
The above code creates a simple HTTP server that listens on port 8080 and returns "Hello, World!" when accessing the root path.
2. Concurrent programming
The Go language implements lightweight concurrency through goroutine, which can easily handle a large number of concurrent tasks. The following is a simple concurrent calculation example:
package main import ( "fmt" "sync" ) func calculate(wg *sync.WaitGroup, num int) { defer wg.Done() result := 0 for i := 0; i < num; i++ { result += i } fmt.Printf("Result for %d is %d ", num, result) } func main() { var wg sync.WaitGroup wg.Add(2) go calculate(&wg, 1000) go calculate(&wg, 2000) wg.Wait() }
The above code creates two goroutines to concurrently calculate the sum from 1 to 1000 and 1 to 2000, and finally outputs the result.
3. Database operation
Go language provides a rich database operation library and supports multiple database types. The following is a simple MySQL database operation example:
package main import ( "database/sql" "fmt" _ "github.com/go-sql-driver/mysql" ) func main() { db, err := sql.Open("mysql", "user:password@tcp(127.0.0.1:3306)/database") if err != nil { fmt.Println("Failed to connect to database") return } defer db.Close() _, err = db.Exec("CREATE TABLE IF NOT EXISTS users(id INT PRIMARY KEY, name VARCHAR(50))") if err != nil { fmt.Println("Failed to create table") return } _, err = db.Exec("INSERT INTO users(id, name) VALUES(1, 'Alice')") if err != nil { fmt.Println("Failed to insert data") return } fmt.Println("Data inserted successfully") }
The above code connects to the MySQL database, creates a table named users, and inserts a piece of data.
Conclusion
Through the above examples, we can see the practical application of Go language in software development. Whether it is server-side development, concurrent programming or database operations, Go language performs well. If you are interested in the Go language, you may wish to learn more and explore more possibilities.
The above is the detailed content of Explore the practical applications of the Go programming language in software development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


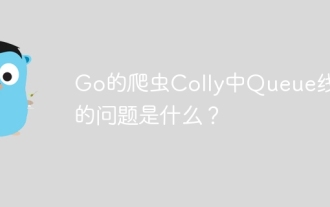
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
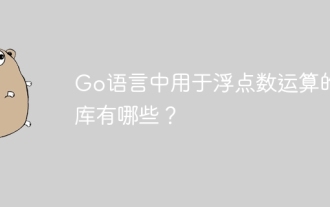
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
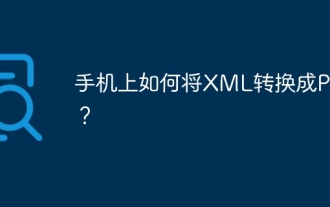
It is not easy to convert XML to PDF directly on your phone, but it can be achieved with the help of cloud services. It is recommended to use a lightweight mobile app to upload XML files and receive generated PDFs, and convert them with cloud APIs. Cloud APIs use serverless computing services, and choosing the right platform is crucial. Complexity, error handling, security, and optimization strategies need to be considered when handling XML parsing and PDF generation. The entire process requires the front-end app and the back-end API to work together, and it requires some understanding of a variety of technologies.
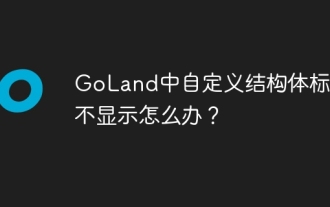
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
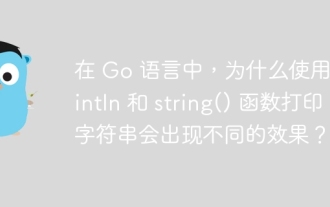
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
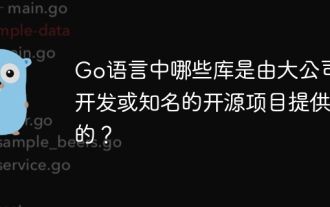
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
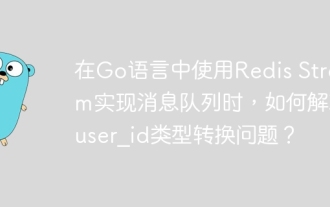
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
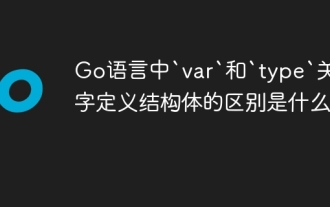
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
