


How to determine whether a Golang string ends with a specified character
Title: How to determine whether a string ends with a specified character in Golang
In the Go language, sometimes we need to determine whether a string ends with a specific character at the end, which is very common when working with strings. This article will introduce how to use the Go language to implement this function, and provide code examples for your reference.
First, let’s take a look at how to determine whether a string ends with a specified character in Golang. The characters in a string in Golang can be obtained through indexing, and the length of the string can be obtained through the built-in len()
function. Therefore, we can determine whether it ends with the specified character by comparing the last few characters of the string.
The following is a sample code that demonstrates how to determine whether a string ends with a specified character:
package main import ( "fmt" ) func endsWith(s string, suffix string) bool { if len(suffix) > len(s) { return false } return s[len(s)-len(suffix):] == suffix } func main() { str := "Hello, World!" suffix := "ld!" if endsWith(str, suffix) { fmt.Printf("字符串 '%s' 以 '%s' 结尾 ", str, suffix) } else { fmt.Printf("字符串 '%s' 不以 '%s' 结尾 ", str, suffix) } }
In this code, the endsWith()
function is used Determine whether the string s
ends with the string suffix
. First, we compared the length of suffix
and the length of s
. If suffix
is longer than s
, then it is definitely impossible to use suffix
End. Next, we determine whether it ends with suffix
by comparing whether the last character in s
that is the same length as suffix
is equal to suffix
. Finally, in the main()
function, we call the endsWith()
function to make a judgment and print out the result.
The above is the method in Golang to determine whether a string ends with a specified character. I hope it will be helpful to you. If you have other questions or doubts, please leave a message to communicate.
The above is the detailed content of How to determine whether a Golang string ends with a specified character. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


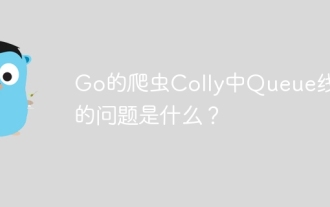
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
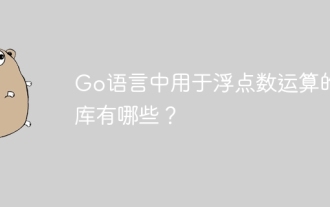
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
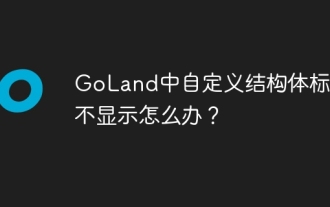
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
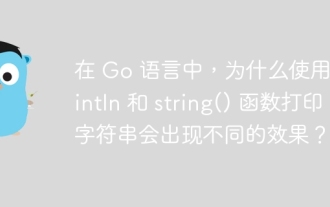
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
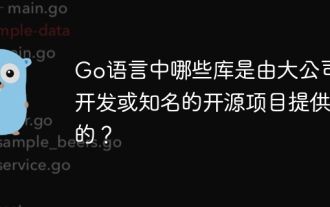
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
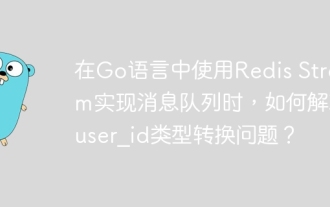
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
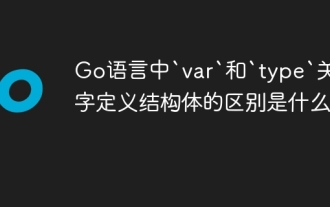
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
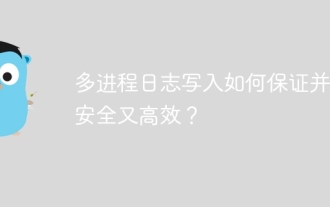
Efficiently handle concurrency security issues in multi-process log writing. Multiple processes write the same log file at the same time. How to ensure concurrency is safe and efficient? This is a...
