Understand the mechanism and application of Golang stack management
Golang is an open source programming language developed by Google. It has many unique features in concurrent programming and memory management. Among them, Golang's stack management mechanism is an important feature. This article will focus on the mechanism and application of Golang's stack management, and give specific code examples.
1. Stack management in Golang
In Golang, each goroutine has its own stack. The stack is used to store information such as parameters, local variables, and function return addresses for function calls. Golang's stack grows and shrinks dynamically. When goroutine executes a function, the stack will dynamically allocate a certain amount of space. If there is not enough stack space, Golang will automatically expand the stack size. When the function completes execution, the stack space will be released.
Golang's stack management is based on segmented stack, that is, the stack is divided into multiple segments, each segment has a fixed size. When the stack needs to be expanded, Golang dynamically allocates more segments to expand the stack size.
2. Application of stack management
Golang’s stack management mechanism brings many conveniences to developers, especially in concurrent programming.
2.1 Avoid stack overflow
In the traditional thread model, each thread has a fixed-size stack. If the recursion level is too deep or there are too many function calls, it is easy to cause stack overflow. In Golang, since the stack is dynamically managed, the size of the stack can be dynamically expanded as needed to avoid stack overflow problems.
2.2 Improve concurrency performance
Since Golang's goroutines are lightweight threads, the stack size of each goroutine is usually only a few KB, so a large number of goroutines can be created. Moreover, due to the dynamic management of the stack, system resources can also be used more efficiently and concurrency performance can be improved.
2.3 Reduce memory usage
Golang’s stack management can dynamically allocate and release stack space as needed, effectively reducing memory usage. This is especially important for applications that need to create a large number of goroutines, which can save a lot of memory space.
3. Code example
A simple code example is given below to demonstrate how to use goroutine and stack management mechanism in Golang:
package main import ( "fmt" "runtime" "sync" ) func recursiveFunc(n int) { if n <= 0 { return } fmt.Println("Recursive:", n) recursiveFunc(n - 1) } func main() { runtime.GOMAXPROCS(1) // 限制仅使用单个CPU核心 var wg sync.WaitGroup wg.Add(1) go func() { defer wg.Done() recursiveFunc(1000) }() wg.Wait() fmt.Println("Done") }
In the above code, We created a goroutine that called a recursive function recursiveFunc
. Due to Golang's stack management mechanism, even if the function call level is very deep, the program will not crash due to stack overflow.
Conclusion
Through this article’s discussion of Golang’s stack management mechanism and demonstration of specific code examples, I hope readers will have a deeper understanding of Golang’s concurrent programming and memory management. With Golang's powerful concurrency support and excellent stack management mechanism, developers can more easily write high-performance concurrent applications.
The above is the detailed content of Understand the mechanism and application of Golang stack management. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
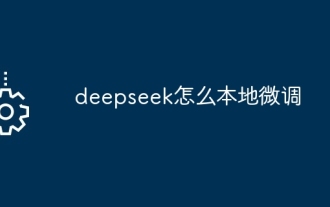
Local fine-tuning of DeepSeek class models faces the challenge of insufficient computing resources and expertise. To address these challenges, the following strategies can be adopted: Model quantization: convert model parameters into low-precision integers, reducing memory footprint. Use smaller models: Select a pretrained model with smaller parameters for easier local fine-tuning. Data selection and preprocessing: Select high-quality data and perform appropriate preprocessing to avoid poor data quality affecting model effectiveness. Batch training: For large data sets, load data in batches for training to avoid memory overflow. Acceleration with GPU: Use independent graphics cards to accelerate the training process and shorten the training time.
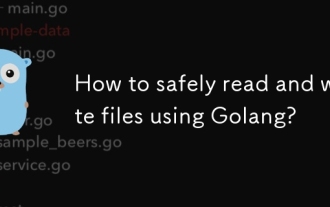
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
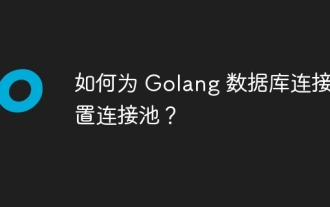
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
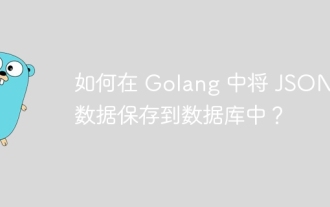
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
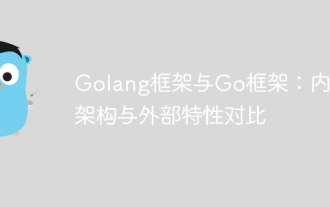
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
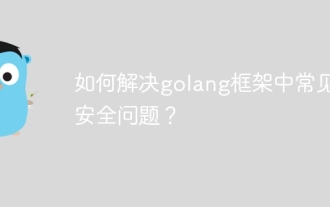
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
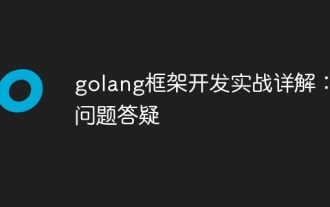
In Go framework development, common challenges and their solutions are: Error handling: Use the errors package for management, and use middleware to centrally handle errors. Authentication and authorization: Integrate third-party libraries and create custom middleware to check credentials. Concurrency processing: Use goroutines, mutexes, and channels to control resource access. Unit testing: Use gotest packages, mocks, and stubs for isolation, and code coverage tools to ensure sufficiency. Deployment and monitoring: Use Docker containers to package deployments, set up data backups, and track performance and errors with logging and monitoring tools.
