


Efficient implementation of array difference calculation in PHP
Array difference calculation is a common operation in PHP. It is usually used to compare the difference between two arrays and find out the same, new and deleted items. In this article, an efficient implementation method will be introduced and specific code examples will be provided.
In PHP, you can use the array_diff
function to calculate the difference between two arrays. This function accepts two arrays as arguments and returns a new array consisting of elements present in the first array but not present in the other arguments. However, the array_diff
function can only calculate the difference between one array and another array, but cannot distinguish between newly added and deleted elements.
In order to analyze the differences in the arrays in more detail, we can customize a function to find out the new, deleted and identical items between them by comparing the elements of the two arrays. The following is an efficient implementation method with specific code examples:
function array_diff_detail($arr1, $arr2){ $diff = array(); $intersect = array(); foreach($arr1 as $val){ if(!in_array($val, $arr2)){ $diff['added'][] = $val; } else { $intersect[] = $val; } } foreach($arr2 as $val){ if(!in_array($val, $arr1)){ $diff['deleted'][] = $val; } } $diff['intersect'] = $intersect; return $diff; } // 示例用法 $arr1 = array('a', 'b', 'c', 'd'); $arr2 = array('a', 'c', 'e', 'f'); $result = array_diff_detail($arr1, $arr2); echo "新增的元素: "; print_r($result['added']); echo "删除的元素: "; print_r($result['deleted']); echo "相同的元素: "; print_r($result['intersect']);
In the above code example, we first define a array_diff_detail
function that accepts two arrays as parameters, And returns an associative array containing new, deleted and identical elements. We then use this function to calculate the difference between the two sample arrays and print out the new, deleted, and identical elements.
Through this method, we can analyze the difference between the two arrays more accurately, not only to find out the new and deleted elements, but also to obtain their specific values. This efficient implementation method can help us better handle array differences and perform corresponding business logic processing in actual projects.
The above is the detailed content of Efficient implementation of array difference calculation in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


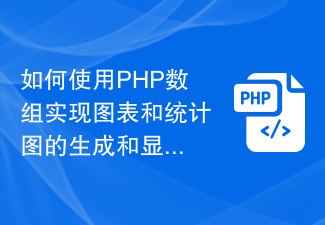
How to use PHP arrays to generate and display charts and statistical graphs. PHP is a widely used server-side scripting language with powerful data processing and graphic generation capabilities. In web development, we often need to display charts and statistical graphs of data. Through PHP arrays, we can easily implement these functions. This article will introduce how to use PHP arrays to generate and display charts and statistical graphs, and provide relevant code examples. Introducing the necessary library files and style sheets Before starting, we need to introduce some necessary library files into the PHP file
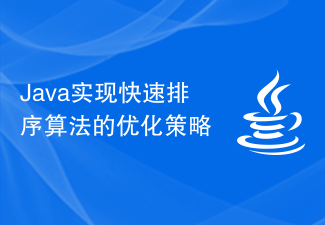
Title: Efficient method and code example to implement quick sort algorithm in Java Introduction: Quick sort is an efficient sorting algorithm, which is based on the idea of divide and conquer and has better performance under average circumstances. This article will introduce the implementation process of the quick sort algorithm in detail through Java code examples, along with performance optimization tips to improve its efficiency. 1. Algorithm principle: The core idea of quick sort is to select a benchmark element and divide the sequence to be sorted into two subsequences through one sorting. The elements of one subsequence are smaller than the benchmark element, and the elements of the other subsequence are smaller than the benchmark element.

How to use PHP arrays to generate dynamic slideshows and picture displays. Slideshows and picture displays are common functions in web design and are often used in scenarios such as carousels and gallery displays. As a popular server-side scripting language, PHP has the ability to process data and generate dynamic HTML pages, and is very suitable for generating dynamic slideshows and picture displays. This article will introduce how to use PHP arrays to generate dynamic slideshows and picture displays, and give corresponding code examples. Prepare image data First, we need to prepare a set of image path data

Implementing an efficient URL route resolution solution in PHP When developing web applications, URL route resolution is a very important link. It can help us implement a friendly URL structure and map requests to the corresponding handlers or controllers. This article will introduce an efficient URL routing resolution solution and provide specific code examples. 1. The basic principle of URL route parsing The basic principle of URL route parsing is to split the URL into different parts and match and map them based on the contents of these parts. Common UR
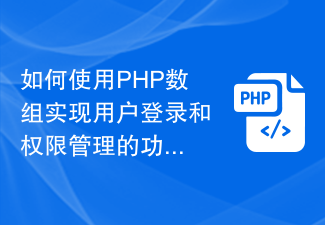
How to use PHP arrays to implement user login and permission management functions When developing a website, user login and permission management are one of the very important functions. User login allows us to authenticate users and protect the security of the website. Permission management can control users' operating permissions on the website to ensure that users can only access the functions for which they are authorized. In this article, we will introduce how to use PHP arrays to implement user login and permission management functions. We'll use a simple example to demonstrate this process. First we need to create
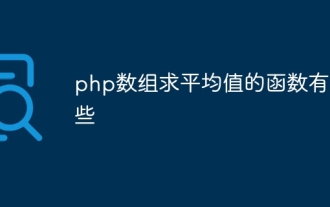
PHP array averaging functions include: 1. array_sum(), which is used to calculate the sum of all values in the array. In order to calculate the average, you can add all the values in the array and then divide by the number of array elements; 2 , array_reduce(), used to iterate the array and calculate each value with an initial value; 3. array_mean(), used to return the average of the array, first calculate the sum of the array, and calculate the number of array elements, then The sum is divided by the number of array elements to get the average.
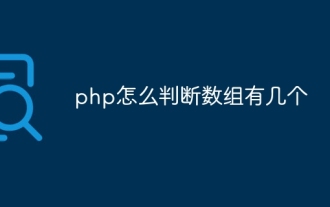
There are several ways to determine an array in PHP: 1. Use the count() function, which is suitable for all types of arrays. However, it should be noted that if the parameter passed in is not an array, the count() function will return 0; 2. Use the sizeof() function, which is more used to maintain compatibility with other programming languages; 3. Custom functions, By using a loop to traverse the array, each time it is traversed, the counter is incremented by 1, and finally the length of the array is obtained. Custom functions can be modified and expanded according to actual needs, making them more flexible.
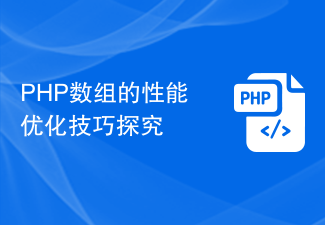
PHP array is a very common data structure that is often used during the development process. However, as the amount of data increases, array performance can become an issue. This article will explore some performance optimization techniques for PHP arrays and provide specific code examples. 1. Use appropriate data structures In PHP, in addition to ordinary arrays, there are some other data structures, such as SplFixedArray, SplDoublyLinkedList, etc., which may perform better than ordinary arrays in certain situations.
