How to iterate through each character of a string in Golang?
How to iterate through each character of a string in Golang?
In Golang, strings are actually composed of bytes, and each character may be represented by multiple bytes. Therefore, to iterate over each character of a string, we need to first convert the string into a rune slice. rune is a data type in the Go language that is used to represent Unicode characters.
Next, I will introduce the method of string traversal using Go language and provide code examples.
First, we define a string containing Chinese characters:
package main import ( "fmt" ) func main() { str := "你好,世界!" runes := []rune(str) for i := 0; i < len(runes); i++ { fmt.Printf("%c ", runes[i]) } }
In this code, we first convert the string "Hello, world!" into a rune slice. Then, we use a for loop to traverse the rune slice and output each character through the fmt.Printf("%c ", runes[i])
statement.
When we run this code, the output is:
你 好 , 世 界 !
With the above example, we successfully iterated through the string containing Chinese characters and output each character. In Golang, this method is a relatively simple and efficient way to traverse strings. I hope this article is helpful to you, thank you for reading!
The above is the detailed content of How to iterate through each character of a string in Golang?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


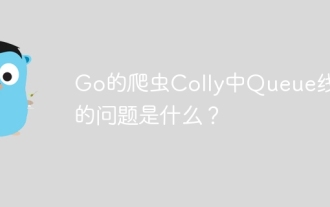
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
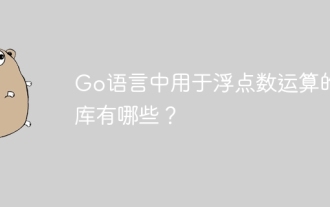
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
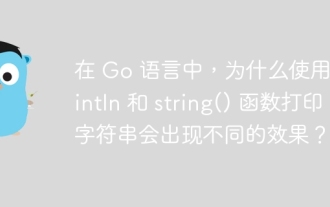
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
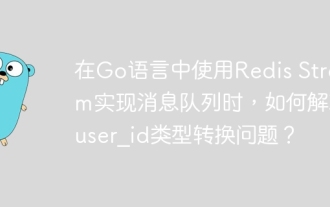
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
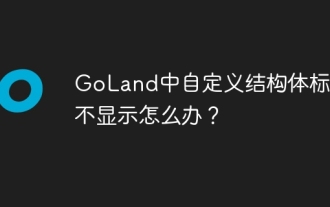
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
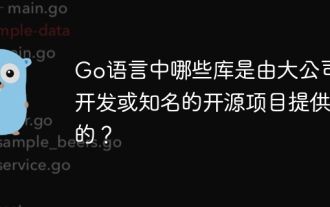
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
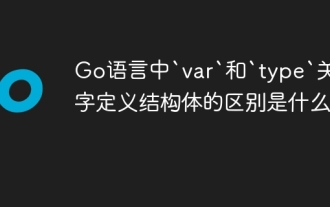
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
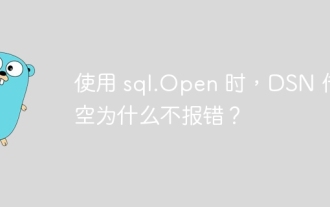
When using sql.Open, why doesn’t the DSN report an error? In Go language, sql.Open...
