What are the string processing methods in Golang?
Title: Golang's string processing methods and code examples
Golang is a fast, concise and efficient programming language that provides rich string processing Methods that allow developers to easily perform various operations on strings. In this article, we will introduce some commonly used Golang string processing methods and provide specific code examples.
- String length
In Golang, the length of the string, that is, the characters in the string, can be obtained through the built-in len()
function number. The following is a sample code:
package main import ( "fmt" ) func main() { str := "Hello, World!" length := len(str) fmt.Println("字符串长度:", length) }
The output result is:
字符串长度: 13
- String concatenation
You can use the
operation in Golang characters to concatenate strings. The following is an example of string concatenation:
package main import ( "fmt" ) func main() { str1 := "Hello" str2 := "World" result := str1 + ", " + str2 + "!" fmt.Println(result) }
The output is:
Hello, World!
- String splitting
By using strings
The Split()
function in the package can split a string according to the specified delimiter. The following is a sample code:
package main import ( "fmt" "strings" ) func main() { str := "apple,orange,banana" result := strings.Split(str, ",") for _, s := range result { fmt.Println(s) } }
The output result is:
apple orange banana
- String replacement
Use the strings
package Replace()
The function can realize the replacement operation of the specified substring in the string. The following is a sample code:
package main import ( "fmt" "strings" ) func main() { str := "Hello, Golang!" newStr := strings.Replace(str, "Golang", "World", -1) fmt.Println(newStr) }
The output result is:
Hello, World!
- String search
through the strings
package Contains()
The function can check whether a string contains a specified substring. The following is a sample code:
package main import ( "fmt" "strings" ) func main() { str := "Go is a programming language" if strings.Contains(str, "programming") { fmt.Println("包含子串programming") } else { fmt.Println("不包含子串programming") } }
The output result is:
包含子串programming
Summary:
In Golang, string processing is a common programming task. Through the methods and code examples introduced above, we can clearly understand how to process strings in Golang, including operations such as obtaining string length, string concatenation, string splitting, string replacement, and string search. These methods can help developers process string data more efficiently and improve code readability and maintainability. I hope readers can have a deeper understanding of string processing in Golang through this article.
The above is the detailed content of What are the string processing methods in Golang?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


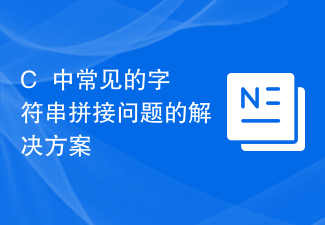
Solutions to common string splicing problems in C++ In C++ programming, string splicing is a common operation, usually used to splice two or more strings, or convert other data types into strings and then splice them. When dealing with string concatenation, we need to consider performance and code simplicity. This article will introduce several common string splicing schemes and give corresponding code examples. Splicing using the "+" Operator The simplest method of string concatenation is to concatenate two strings using the "+" operator. example
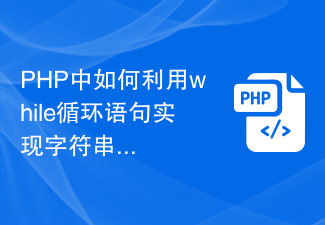
Title: Using while loops to implement string splicing in PHP In the PHP language, using while loop statements to implement string splicing is a common operation. Loop through an array, list, or other data source, concatenating each element or value into a string. This method is useful when dealing with large amounts of data or when strings need to be generated dynamically. Let's look at some specific code examples below. First, we prepare an array as the data source, and then use a while loop to implement string splicing
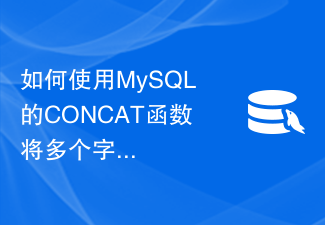
How to use MySQL's CONCAT function to splice multiple strings together. In the MySQL database, we often encounter situations where we need to splice multiple strings together. In this case, we can use the CONCAT function provided by MySQL to achieve this. The CONCAT function can concatenate multiple strings into one string, which is very convenient and practical. The method of using the CONCAT function is very simple. You only need to pass the string to be spliced as a parameter to the CONCAT function in a certain format. The following is done using C
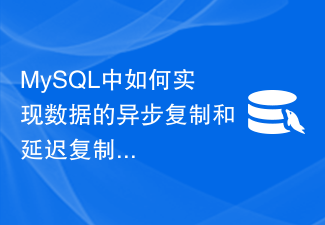
MySQL is a commonly used relational database management system. In practical applications, we often encounter scenarios that require data replication. Data replication can be divided into two forms: synchronous replication and asynchronous replication. Synchronous replication means that the data must be copied to the slave database immediately after the master database writes the data, while asynchronous replication means that the data can be delayed for a certain period of time after the master database writes the data before copying. This article will focus on how to implement asynchronous replication and delayed replication of data in MySQL. First, in order to implement asynchronous replication and delayed replication, I
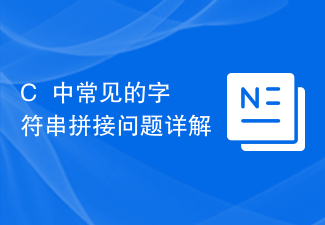
Detailed explanation of common string concatenation problems in C++, specific code examples are required In C++ programming, string concatenation is a common task. Whether it is simply splicing several strings or complex string operations, you need to master some basic skills and methods. This article will introduce in detail common string concatenation issues in C++ and provide specific code examples. Use the + operator to concatenate In C++, you can use the + operator to concatenate two strings. Here's a simple example: #include<i
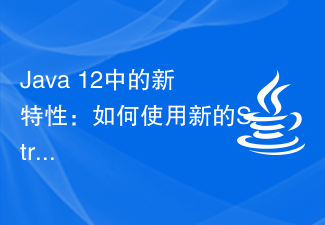
New features in Java12: How to use the new StringAPI for string concatenation Introduction: String concatenation is a very common operation in daily Java development. The traditional method is to use the "+" operator or the String.concat() method to achieve it. However, with the release of Java12, the new StringAPI was introduced, providing a more efficient and convenient way to concatenate strings. This article will introduce the new features in Java12 and demonstrate them through code examples
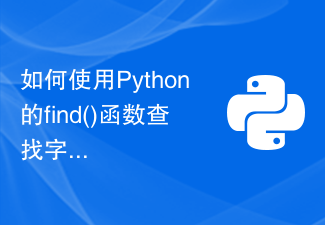
How to use Python's find() function to find substrings in a string. In Python's string processing, it is often necessary to find substrings in a string. Python provides the find() function to help us achieve this function. This article will introduce how to use Python's find() function to find substrings in a string, and give specific code examples. The find() function is a built-in method of the Python string object, which is used to find the position of a substring in a string. The letter
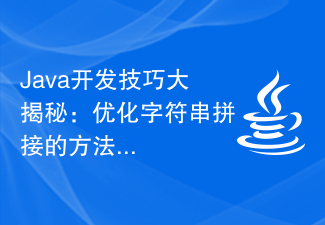
As a programming language widely used in software development, Java's flexibility and scalability make it the first choice for many developers. In Java development, string concatenation is a common and important task. However, incorrect string concatenation methods can lead to performance degradation and resource waste. In order to solve this problem, this article will reveal some methods to optimize string splicing to help developers process strings more efficiently in their daily work. First, let’s understand the immutability of strings in Java. in Java
